Question
1. Write a Java program that prompts for and reads 10 integers from the user into an integer array of size 10, it then: -
1. Write a Java program that prompts for and reads 10 integers from the user into an integer array of size 10, it
then:
- calculates the sum and average of positive numbers of the array and displays them.
[Note: consider 0 as positive]
- Displays the array indexes where positive integers appear in the array.
Note: The program must display the message: "There are no positive values" if the array does not contain any
positive value.
2. Write a Java program that prompts for and reads the size n of a 1D-array of type double. If the size is invalid,
the program displays an appropriate error message and loops until the user enters a valid size. If the size is
valid, the program prompts for and reads n numbers into the array, it then finds and displays the maximum
and minimum values and the indexes (subscripts) of the first occurrences of these maximum and minimum
values in the array.
Sample program run:
3.Write a Java program that prompts for and reads the size n of an integer array, it prints an appropriate error message and loops as long as n is not a valid array size. If the array size is valid the program initializes an integer array of size n with n pseudo-random integers from 1 to 30.
Print the initialized array then prompt the user to enter a target value to search for in the array by using linear search. If present, your program should display its first position in the array, otherwise print a message that the value is not in the array.
Note: To generate a random integer in the interval [1, 30] use the method call:
(int)(Math.random( )*30) + 1
random( ) returns a double value in the interval [0.0, 1.0)
4.Write a Java program that prompts for and reads the size of an array of type double. If the size is invalid, the program displays an appropriate error message and loops until the user enters a valid size. If the array size is valid the program prompts for and reads the array elements. The program then determines whether the array is sorted in non-decreasing order or not by displaying one of the following messages: The array is sorted in non-decreasing order or The array is not sorted in non-decreasing order.
Note: An array x with n elements is sorted in non-decreasing order if:
x1 x2 x3 . . . xn
Step by Step Solution
There are 3 Steps involved in it
Step: 1
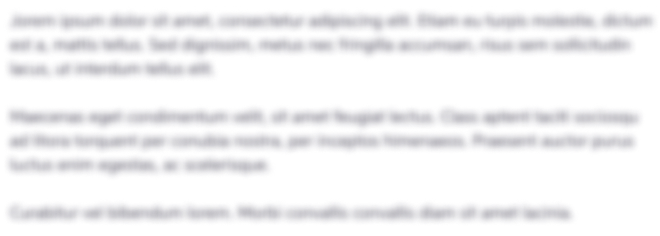
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started