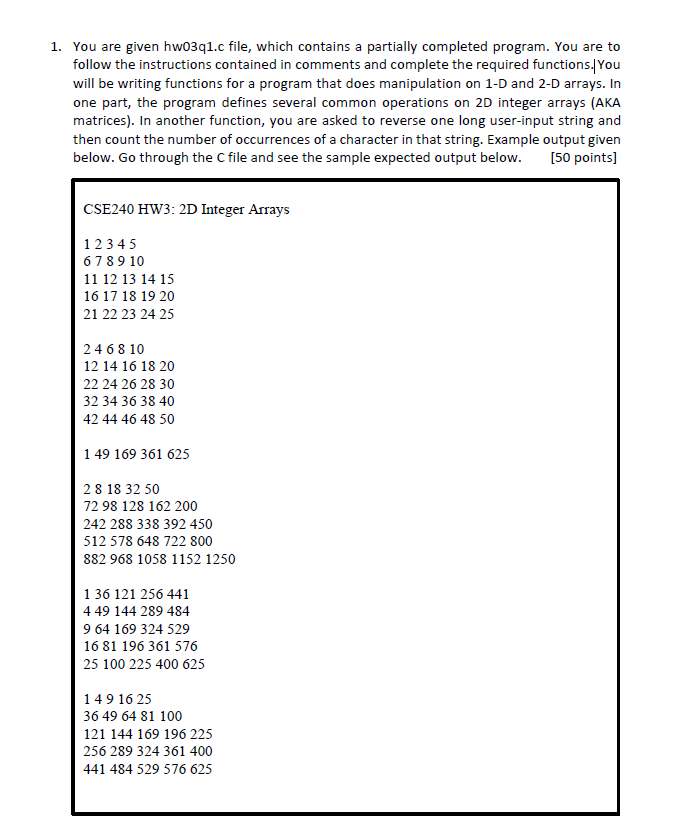
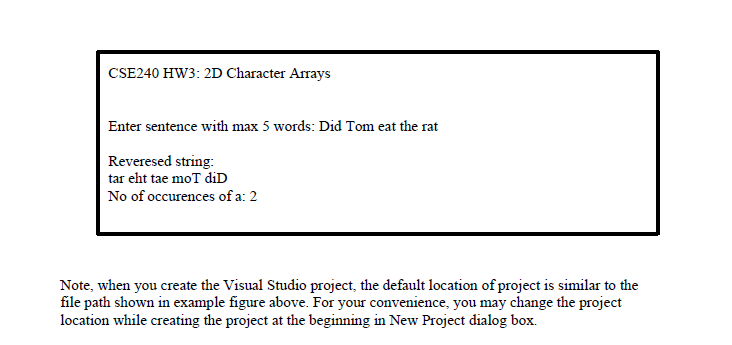
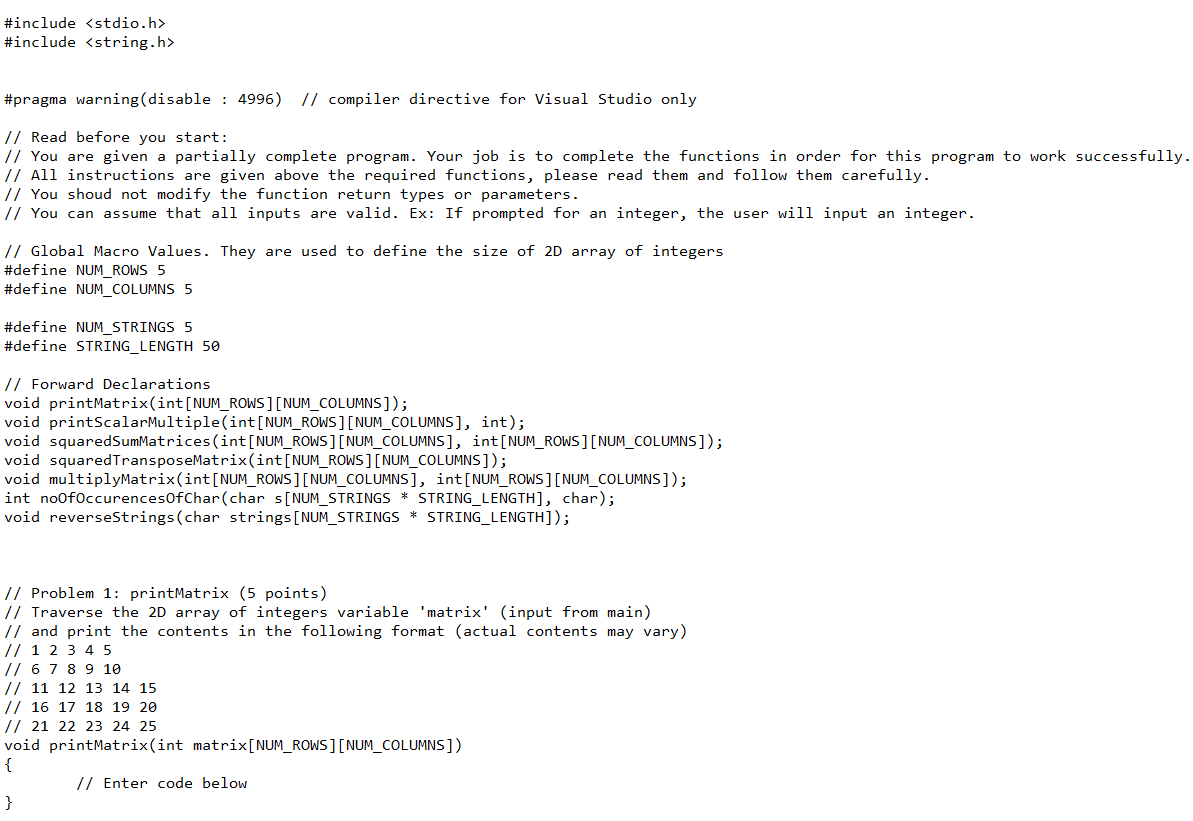
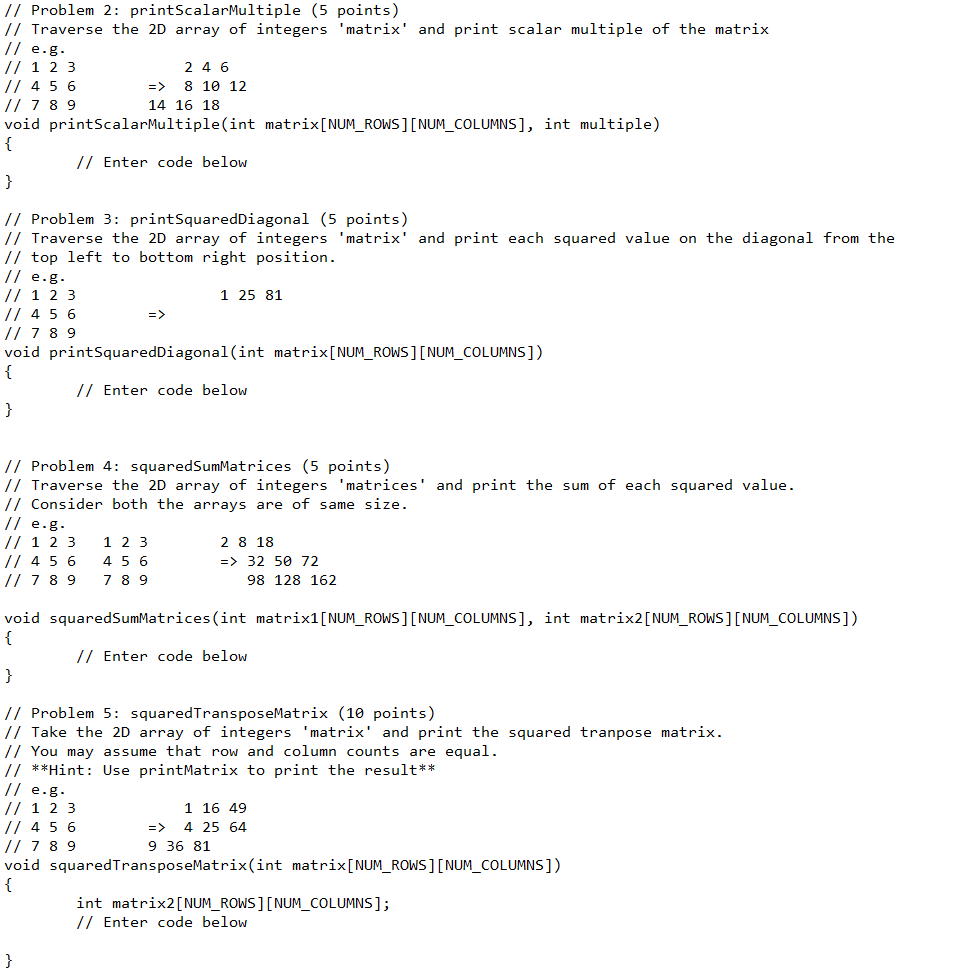
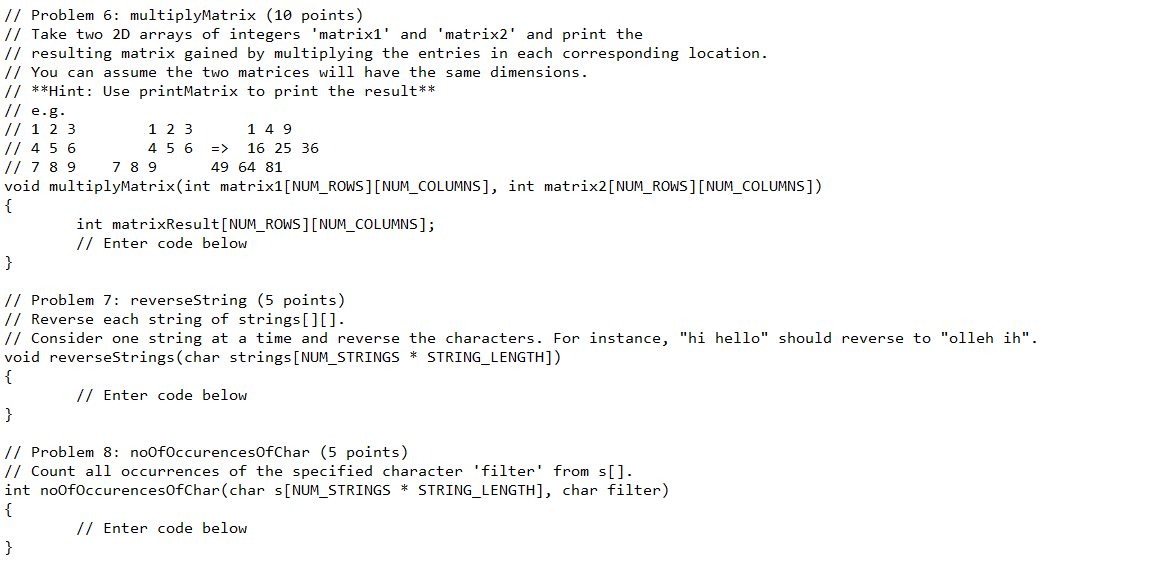
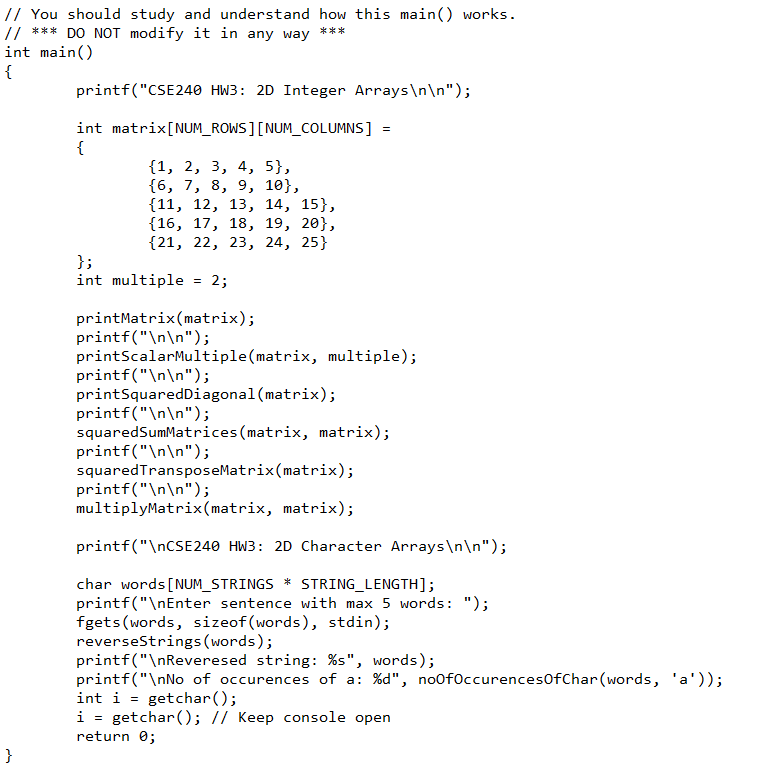
1. You are given hw031.c file, which contains a partially completed program. You are to follow the instructions contained in comments and complete the required functions. You will be writing functions for a program that does manipulation on 1-D and 2-D arrays. In one part, the program defines several common operations on 2D integer arrays (AKA matrices). In another function, you are asked to reverse one long user-input string and then count the number of occurrences of a character in that string. Example output given below. Go through the file and see the sample expected output below. (50 points) CSE240 HW3: 2D Integer Arrays 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 24 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 1 49 169 361 625 2 8 18 32 50 72 98 128 162 200 242 288 338 392 450 512 578 648 722 800 882 968 1058 1152 1250 1 36 121 256 441 4 49 144 289 484 9 64 169 324 529 16 81 196 361 576 25 100 225 400 625 14 9 16 25 36 49 64 81 100 121 144 169 196 225 256 289 324 361 400 441 484 529 576 625 CSE240 HW3: 2D Character Arrays Enter sentence with max 5 words: Did Tom eat the rat Reveresed string tar eht tae mot did No of occurences of a: 2 Note, when you create the Visual Studio project, the default location of project is similar to the file path shown in example figure above. For your convenience, you may change the project location while creating the project at the beginning in New Project dialog box. #include
#include #pragma warning( disable : 4996) // compiler directive for Visual Studio only // Read before you start: // You are given a partially complete program. Your job is to complete the functions in order for this program to work successfully. // All instructions are given above the required functions, please read them and follow them carefully. // You shoud not modify the function return types or parameters. // You can assume that all inputs are valid. Ex: If prompted for an integer, the user will input an integer. // Global Macro Values. They are used to define the size of 2D array of integers #define NUM_ROWS 5 #define NUM_COLUMNS 5 #define NUM_STRINGS 5 #define STRING_LENGTH 50 // Forward Declarations void printMatrix(int[NUM_ROWS] [NUM_COLUMNS]); void printScalarMultiple(int[NUM_ROWS] [NUM_COLUMNS], int); void squaredSumMatrices(int[NUM_ROWS] [NUM_COLUMNS], int[NUM_ROWS] [NUM_COLUMNS]); void squaredTransposeMatrix(int[NUM_ROWS][NUM_COLUMNS]); void multiplyMatrix(int[NUM_ROWS] [NUM_COLUMNS], int[NUM_ROWS] [NUM_COLUMNS]); int noofOccurencesOfChar(char s[NUM_STRINGS * STRING_LENGTH], char); void reverseStrings (char strings [NUM_STRINGS * STRING_LENGTH]); // Problem 1: printMatrix (5 points) // Traverse the 2D array of integers variable 'matrix' (input from main) // and print the contents in the following format (actual contents may vary) // 1 2 3 4 5 // 6 7 8 9 10 // 11 12 13 14 15 // 16 17 18 19 20 // 21 22 23 24 25 void printMatrix(int matrix[NUM_ROWS] [NUM_COLUMNS]) { // Enter code below } 1/ Problem 2: printScalarMultiple (5 points) // Traverse the 2D array of integers 'matrix' and print scalar multiple of the matrix // e.g. // 1 2 3 2 4 6 // 4 5 6 => 8 10 12 // 7 8 9 14 16 18 void printScalarMultiple(int matrix[NUM_ROWS] [NUM_COLUMNS], int multiple) { // Enter code below } // Problem 3: printSquaredDiagonal (5 points) // Traverse the 2D array of integers 'matrix' and print each squared value on the diagonal from the // top left to bottom right position. // e.g. // 1 2 3 1 25 81 // 4 5 6 // 7 8 9 void printSquaredDiagonal(int matrix[NUM_ROWS] [NUM_COLUMNS]) { // Enter code below } // Problem 4: squaredSumMatrices (5 points) // Traverse the 2D array of integers 'matrices' and print the sum of each squared value. // Consider both the arrays are of same size. // e.g. // 1 2 3 1 2 3 2 8 18 // 4 5 6 4 5 6 => 32 50 72 // 7 8 9 789 98 128 162 void squaredSumMatrices(int matrix1[NUM_ROWS] [NUM_COLUMNS], int matrix2[NUM_ROWS] [NUM_COLUMNS]) { // Enter code below } // Problem 5: squared TransposeMatrix (10 points) // Take the 2D array of integers 'matrix' and print the squared tranpose matrix. // You may assume that row and column counts are equal. // **Hint: Use printMatrix to print the result** // e.g. // 1 2 3 1 16 49 // 4 5 6 => 4 25 64 // 7 8 9 9 36 81 void squaredTransposeMatrix(int matrix[NUM_ROWS] [NUM_COLUMNS]) { int matrix2[NUM_ROWS] [NUM_COLUMNS]; // Enter code below } // Problem 6: multiplyMatrix (10 points) // Take two 2D arrays of integers 'matrixl' and 'matrix2' and print the // resulting matrix gained by multiplying the entries in each corresponding location. // You can assume the two matrices will have the same dimensions. // **Hint: Use printMatrix to print the result** // e.g. // 1 2 3 1 2 3 1 49 // 4 5 6 4 5 6 16 25 36 // 7 8 9 7 8 9 49 64 81 void multiplyMatrix(int matrix1[NUM_ROWS] [NUM_COLUMNS], int matrix2[NUM_ROWS] [NUM_COLUMNS]) { int matrixResult[NUM_ROWS] [NUM_COLUMNS]; // Enter code below } // Problem 7: reverseString (5 points) // Reverse each string of strings[][]. // Consider one string at a time and reverse the characters. For instance, "hi hello" should reverse to "olleh ih". void reverseStrings (char strings [NUM_STRINGS * STRING_LENGTH]) { // Enter code below } // Problem 8: noofOccurencesOfChar (5 points) // Count all occurrences of the specified character 'filter' from s[]. int noofOccurencesOfChar(char s[NUM_STRINGS * STRING_LENGTH], char filter) { // Enter code below } *** // You should study and understand how this main() works. // *** DO NOT modify it in any way int main() { printf("CSE240 HW3: 2D Integer Arrays "); int matrix[NUM_ROWS] [NUM_COLUMNS] = { {1, 2, 3, 4, 5}, {6, 7, 8, 9, 10}, {11, 12, 13, 14, 15}, {16, 17, 18, 19, 20}, {21, 22, 23, 24, 25} }; int multiple 2; printMatrix(matrix); printf(" "); printScalarMultiple (matrix, multiple); printf(" "); printSquaredDiagonal(matrix); printf(" "); squared SumMatrices (matrix, matrix); printf(" "); squaredTransposeMatrix(matrix); printf(" "); multiplyMatrix(matrix, matrix); printf(" CSE240 HW3: 2D Character Arrays "); char words [NUM_STRINGS * STRING_LENGTH]; printf(" Enter sentence with max 5 words: "); fgets (words, sizeof(words), stdin); reverseStrings (words); printf(" Reveresed string: %s", words); printf(" No of occurences of a: %d", noof OccurencesOfChar(words, 'a')); int i = getchar(); i = getchar(); // Keep console open return 0; }