Question
10.11 Consider the partially completed Coin class below. A Coin has a year it made mintYear, a value at the time it was made faceValue
10.11
Consider the partially completed Coin class below. A Coin has a year it made mintYear, a value at the time it was made faceValue and the country of origin.
public class Coin{ private int mintYear; //year coin was made private double faceValue; //value of coin at time it was made private String country; //origin country public Coin(int year, double value, String ctry){ mintYear = year; faceValue = value; country = ctry; } //other constructors and methods not shown public int getYear(){ return mintYear; } }
The CoinCollection class has an ArrayList of Coins. The constructor of the CoinCollection class takes arrays for the years, values and countries. These arrays are parallel arrays. Thus if I have three coins: A US quarter from 1900, a US dime from 1910 and a US nickel from 1920, the arrays would contain: Years = { 1900, 1910, 1920} Values = { 0.25, 0.10, 0.05} Countries = { "US", "US", "US"} The partially completed CoinCollection class is below.
public class CoinCollection{ private ArrayList
Part A Write the CoinCollection constructor. The USCoinCollection class extends the CoinCollection class. A partially completed CoinCollection and USCoinCollection classes are below:
import java.util.ArrayList; public class CoinCollection{ private ArrayList
Part B A rare coin in one that was minted prior to a certain year. A rare coin collection will have all coins minted prior to a certain year. The isRare() method takes a year parameter and determines if a coin collection is rare. Below is the method header for the isRare() method:
public boolean isRare(int year) { } Given a CoinCollection myCoins with the following coins: 1930 Mexico 1.00, 1925 US 0.25, 1925 Britain 0.05 Given a USCoinCollection usCoins with the following coins: 1925 US 0.25, 1920 US 0.50, 1908 US 1.00
myCoins.isRare(1930) would return false usCoins.isRare(1930) would return true
Identify which class(es) the isRare() method belongs in and write out the method below.
Rubric:
Part A
- Instantiation of the Coin ArrayList
- Loop set up correctly (initialization, termination, counter, no bounds error)
- Add to the ArrayList correctly
- Constructing new Coin object
- Correct parameters in Coin object
Part B
- Correct placement of method in CoinCollection class (point not awarded if in the USCoinCollection or both classes)
- Looping through the Coin ArrayList with no bounds error
- Correct comparison of coin to the rareYear
- Returning true if and only if all coins have a mint year before the rareYear parameter
- Returning false if one or more of the coin years is not less than the rareYear parameter
Step by Step Solution
There are 3 Steps involved in it
Step: 1
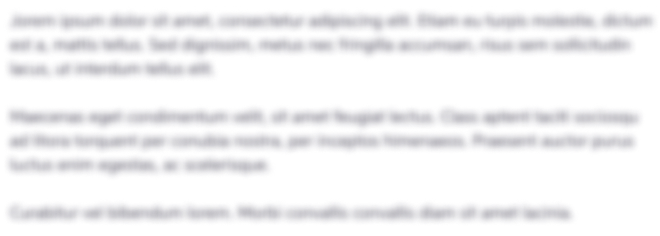
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started