Question
13.4 Creditcard Account (Individual Assignment) Required Skills Inventory Write an derived class in Java according to Interface specifications given in UML. Implement base class Interfaces
13.4 Creditcard Account (Individual Assignment)
Required Skills Inventory
Write an derived class in Java according to Interface specifications given in UML.
Implement base class Interfaces in java according to specifications given in UML.
Problem Description and Given Info
You must write a public class named CreditcardAccount with fields and methods as defined below, and that inherits from (extends) the BankAccount class.
UML CLass Diagram: CreditcardAccount Inherits BankAccount
Structure of the Fields
As described by the UML Class Diagram above, your CreditcardAccount class must have the following fields:
a private field named limit of type int initialized to 0
Structure of the Methods
As described by the UML Class Diagram above, your CreditcardAccount class must have the following methods:
a public method named debit that takes an int argument and returns a boolean
a public method named setLimit that takes an int and returns nothing
a public method named getLimit that takes no arguments and returns an int
a public method named applyInterest that takes no arguments and returns nothing
a public method named accountInfo that takes no arguments and returns a String
Note that three of these methods are defined as abstract in the BankAccount base class. You will be overriding and implementing these methods in this CreditcardAccount concrete derived class.
Behavior of the Methods
The debit method should subtract the argument amount from the balance, but only if the amount would not cause the current balance to violate the credit limit. This method should return true if the amount was subtracted from the balance, otherwise it should return false.
The setLimit method should store the argument amount in the limit field.
The getLimit method should return the value stored in the limit field.
The applyInterest method should compute the interest amount and add this amount to the balance, but only if the balance is less than 0.
The accountInfo method will return a string formatted exactly like this:
Type of Account : Creditcard Account ID : 1111-2222-3333-4444 Current Balance : $123.45 Interest rate : 1.50% Credit Limit : $10000.00
Note that, while the current balance of a CreditcardAccount will almost always be negative, it should be shown as a positive value in the String returned by the accoutnInfo method.
Additional Information
BankAccount Class
Copy and paste your BankAccount class code from your Bank Account (Individual Assignment) into the BankAccount.Java file in the editor below.
All Bank Accounts
All accounts have balance, credit and debit amounts, fees, and limits stored and passed as a number of pennies (int).
All debit amounts will be subtracted from the balance, and all credit amounts will be added to the balance.
All bank accounts have a non-negative interest rate (0.02 would be a 2% interest rate).
When applying interest, interest amount is calculated by multiplying the balance by the interest rate.
When applying interest, interest amount is always added to the balance, and any fractional part will be rounded down.
Interest will not be applied to any Savings or Checking account with a balance of zero or less.
Debit methods will return false if the transaction cannot be made because of insufficient balance or insufficient credit limit. Otherwise they will return true.
The credit method will always return true.
Creditcard Accounts
The balance of a CreditcardAccount cannot overrun its credit limit.
The debit method will return false if an attempt to overdraw the account is made.
The balance of a CreditcardAccount will generally be negative, because when you spend money on a credit card, you are borrowing money, and the negative balance reflects money that you owe.
The credit limit will be stored as a positive value. For example, a credit limit of $10000.00 will be stored in the limit field as the int value 1000000.
Interest will not be applied to a CreditcardAccount with a positive or zero balance.
public abstract class BankAccount { protected String accountID = "0000-0000-0000-0000";
protected double interestRate = 0;
protected int balance = 0;
public boolean credit(int pennies) { balance = balance + pennies; return true; }
public abstract boolean debit(int pennies);
public int getBalance() { return balance; }
public String getAccountID() { return accountID; }
public void setAccountID (String accountID) { this.accountID=accountID; }
public double getInterestRate() { return interestRate; }
public void setInterestRate(double interestRate) { this.interestRate = interestRate; }
public abstract void applyInterest();
public abstract String accountInfo(); }
Please write code for CreditcardAccount.java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
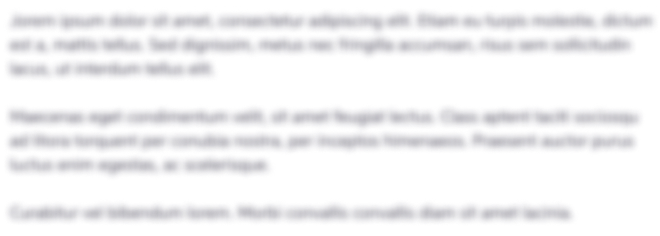
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started