2. Extend the class linkedListType by adding the following operations: a. Find and delete the node with the smallest info in the list. (Delete only
2. Extend the class linkedListType by adding the following operations: a. Find and delete the node with the smallest info in the list. (Delete only the first occurrence and traverse the list only once.) b. Find and delete all occurrences of a given info from the list. (Traverse the list only once.) Add these as abstract functions in the class linkedListType and provide the definitions of these functions in the class unorderedLinkedList. Also write a program to test these functions. This is what was given. Any help is appreciated and please post an output.
arrayListType.h
#pragma once
//***********************************************************
// Author: D.S. Malik
//
// This class specifies the members to implement the basic
// properties of array-based lists.
//***********************************************************
#include
#include
#include "unorderedLinkedList.h"
using namespace std;
template
class arrayListType
{
public:
bool isEmpty() const;
//Function to determine whether the list is empty
//Postcondition: Returns true if the list is empty;
// otherwise, returns false.
bool isFull() const;
//Function to determine whether the list is full.
//Postcondition: Returns true if the list is full;
// otherwise, returns false.
int listSize() const;
//Function to determine the number of elements in the list
//Postcondition: Returns the value of length.
int maxListSize() const;
//Function to determine the size of the list.
//Postcondition: Returns the value of maxSize.
void print() const;
//Function to output the elements of the list
//Postcondition: Elements of the list are output on the
// standard output device.
bool isItemAtEqual(int location, const elemType& item) const;
//Function to determine whether the item is the same
//as the item in the list at the position specified by
//Postcondition: Returns true if the list[location]
// is the same as the item; otherwise,
// returns false.
bool insertAt(int location, const elemType& insertItem);
//Function to insert an item in the list at the
//position specified by location. The item to be inserted
//is passed as a parameter to the function.
//Postcondition: Starting at location, the elements of the
// list are shifted down, list[location] = insertItem;,
// and length++;. If the list is full or location is
// out of range, an appropriate message is displayed.
bool insertEnd(const elemType& insertItem);
//Function to insert an item at the end of the list.
//The parameter insertItem specifies the item to be inserted.
//Postcondition: list[length] = insertItem; and length++;
// If the list is full, an appropriate message is
// displayed.
bool removeAt(int location);
//Function to remove the item from the list at the
//position specified by location
//Postcondition: The list element at list[location] is removed
// and length is decremented by 1. If location is out of
// range,an appropriate message is displayed.
bool retrieveAt(int location, elemType& retItem) const;
//Function to retrieve the element from the list at the
//position specified by location.
//Postcondition: retItem = list[location]
// If location is out of range, an appropriate message is
// displayed.
bool replaceAt(int location, const elemType& repItem);
//Function to replace the elements in the list at the
//position specified by location. The item to be replaced
//is specified by the parameter repItem.
//Postcondition: list[location] = repItem
// If location is out of range, an appropriate message is
// displayed.
void clearList();
//Function to remove all the elements from the list.
//After this operation, the size of the list is zero.
//Postcondition: length = 0;
int seqSearch(const elemType& item) const;
//Function to search the list for a given item.
//Postcondition: If the item is found, returns the location
// in the array where the item is found; otherwise,
// returns -1.
bool insert(const elemType& insertItem);
//Function to insert the item specified by the parameter
//insertItem at the end of the list. However, first the
//list is searched to see whether the item to be inserted
//is already in the list.
//Postcondition: list[length] = insertItem and length++
// If the item is already in the list or the list
// is full, an appropriate message is displayed.
bool remove(const elemType& removeItem);
//Function to remove an item from the list. The parameter
//removeItem specifies the item to be removed.
//Postcondition: If removeItem is found in the list,
// it is removed from the list and length is
// decremented by one.
arrayListType(int size = 100);
//constructor
//Creates an array of the size specified by the
//parameter size. The default array size is 100.
//Postcondition: The list points to the array, length = 0,
// and maxSize = size
protected:
unorderedLinkedList list; //array to hold the list elements
int maxSize; //to store the maximum size of the list
};
template
bool arrayListType::isEmpty() const
{
return list.isEmptyList();
}
template
bool arrayListType::isFull() const
{
return list.length() >= maxSize;
}
template
int arrayListType::listSize() const
{
return list.length();
}
template
int arrayListType::maxListSize() const
{
return maxSize;
}
template
void arrayListType::print() const
{
list.print();
}
template
bool arrayListType::isItemAtEqual(int location, const elemType& item) const
{
if (location < 0 || list.length() <= locaton)
return false;
linkedListIterator it = list.begin();
while (location > 0)
{
++it;
location--;
}
return item == it->info;
}
template
bool arrayListType::insertAt(int location, const elemType& insertItem)
{
if (isFull()) return false;
return list.insertAt(location, insertItem);
} //end insertAt
template
bool arrayListType::insertEnd(const elemType& insertItem)
{
if (isFull()) return false;
list.insertLast(insertItem);
return true;
} //end insertEnd
template
bool arrayListType::removeAt(int location)
{
return list.deleteAt(location);
} //end removeAt
template
bool arrayListType::retrieveAt(int location, elemType& retItem) const
{
return list.retrieveAt(location, retItem);
} //end retrieveAt
template
bool arrayListType::replaceAt(int location, const elemType& repItem)
{
return list.replaceAt(location, repItem);
} //end replaceAt
template
void arrayListType::clearList()
{
list.destroyList();
} //end clearList
template
int arrayListType::seqSearch(const elemType& item) const
{
return list.search(item);
} //end seqSearch
template
bool arrayListType::insert(const elemType& insertItem)
{
if (isFull()) return false;
return list.insertLast(insertItem);
} //end insert
template
bool arrayListType::remove(const elemType& removeItem)
{
return list.deleteNode(removeItem);
} //end remove
template
arrayListType::arrayListType(int size)
{
if (size <= 0) size = 100;
maxSize = size;
}
linkedList.h
#pragma once
#include
#include
using namespace std;
//Definition of the node
template
struct nodeType
{
Type info;
nodeType *link;
};
//***********************************************************
// Author: D.S. Malik
//
// This class specifies the members to implement an iterator
// to a linked list.
//***********************************************************
template
class linkedListIterator
{
public:
linkedListIterator();
//Default constructor
//Postcondition: current = NULL;
linkedListIterator(nodeType *ptr);
//Constructor with a parameter.
//Postcondition: current = ptr;
Type operator*();
//Function to overload the dereferencing operator *.
//Postcondition: Returns the info contained in the node.
linkedListIterator operator++();
//Overload the preincrement operator.
//Postcondition: The iterator is advanced to the next node.
bool operator==(const linkedListIterator& right) const;
//Overload the equality operator.
//Postcondition: Returns true if this iterator is equal to
// the iterator specified by right, otherwise it returns
// false.
bool operator!=(const linkedListIterator& right) const;
//Overload the not equal to operator.
//Postcondition: Returns true if this iterator is not equal to
// the iterator specified by right, otherwise it returns
// false.
private:
nodeType *current; //pointer to point to the current
//node in the linked list
};
template
linkedListIterator::linkedListIterator()
{
current = NULL;
}
template
linkedListIterator::linkedListIterator(nodeType *ptr)
{
current = ptr;
}
template
Type linkedListIterator::operator*()
{
return current->info;
}
template
linkedListIterator linkedListIterator::operator++()
{
current = current->link;
return *this;
}
template
bool linkedListIterator::operator==(const linkedListIterator& right) const
{
return (current == right.current);
}
template
bool linkedListIterator::operator!=(const linkedListIterator& right) const
{
return (current != right.current);
}
//***********************************************************
// Author: D.S. Malik
//
// This class specifies the members to implement the basic
// properties of a linked list. This is an abstract class.
// We cannot instantiate an object of this class.
//***********************************************************
template
class linkedListType
{
public:
const linkedListType& operator= (const linkedListType&);
//Overload the assignment operator.
void initializeList();
//Initialize the list to an empty state.
//Postcondition: first = NULL, last = NULL, count = 0;
bool isEmptyList() const;
//Function to determine whether the list is empty.
//Postcondition: Returns true if the list is empty, otherwise
// it returns false.
void print() const;
//Function to output the data contained in each node.
//Postcondition: none
int length() const;
//Function to return the number of nodes in the list.
//Postcondition: The value of count is returned.
void destroyList();
//Function to delete all the nodes from the list.
//Postcondition: first = NULL, last = NULL, count = 0;
Type front() const;
//Function to return the first element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program terminates;
// otherwise, the first element of the list is returned.
Type back() const;
//Function to return the last element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the last
// element of the list is returned.
virtual int search(const Type& searchItem) const = 0;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is in the list,
// otherwise the value false is returned.
virtual void insertFirst(const Type& newItem) = 0;
//Function to insert newItem at the beginning of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the beginning of the list, last points to
// the last node in the list, and count is incremented by 1.
virtual void insertLast(const Type& newItem) = 0;
//Function to insert newItem at the end of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the end of the list, last points to the
// last node in the list, and count is incremented by 1.
virtual bool deleteNode(const Type& deleteItem) = 0;
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing deleteItem is
// deleted from the list. first points to the first node,
// last points to the last node of the updated list, and
// count is decremented by 1.
linkedListIterator begin();
//Function to return an iterator at the beginning of the linked list.
//Postcondition: Returns an iterator such that current is set to first.
linkedListIterator end();
//Function to return an iterator one element past the last element of the linked list.
//Postcondition: Returns an iterator such that current is set to NULL.
linkedListType();
//default constructor
//Initializes the list to an empty state.
//Postcondition: first = NULL, last = NULL, count = 0;
linkedListType(const linkedListType& otherList);
//copy constructor
~linkedListType();
//destructor
//Deletes all the nodes from the list.
//Postcondition: The list object is destroyed.
protected:
int count; //variable to store the number of list elements
//
nodeType *first; //pointer to the first node of the list
nodeType *last; //pointer to the last node of the list
private:
void copyList(const linkedListType& otherList);
//Function to make a copy of otherList.
//Postcondition: A copy of otherList is created and assigned
// to this list.
};
template
bool linkedListType::isEmptyList() const
{
return (first == NULL);
}
template
linkedListType::linkedListType() //default constructor
{
first = NULL;
last = NULL;
count = 0;
}
template
void linkedListType::destroyList()
{
nodeType *temp; //pointer to deallocate the memory
//occupied by the node
while (first != NULL) //while there are nodes in the list
{
temp = first; //set temp to the current node
first = first->link; //advance first to the next node
delete temp; //deallocate the memory occupied by temp
}
last = NULL; //initialize last to NULL; first has already
//been set to NULL by the while loop
count = 0;
}
template
void linkedListType::initializeList()
{
destroyList(); //if the list has any nodes, delete them
}
template
void linkedListType::print() const
{
nodeType *current; //pointer to traverse the list
current = first; //set current so that it points to
//the first node
while (current != NULL) //while more data to print
{
cout << current->info << " ";
current = current->link;
}
}//end print
template
int linkedListType::length() const
{
return count;
} //end length
template
Type linkedListType::front() const
{
assert(first != NULL);
return first->info; //return the info of the first node
}//end front
template
Type linkedListType::back() const
{
assert(last != NULL);
return last->info; //return the info of the last node
}//end back
template
linkedListIterator linkedListType::begin()
{
linkedListIterator temp(first);
return temp;
}
template
linkedListIterator linkedListType::end()
{
linkedListIterator temp(NULL);
return temp;
}
template
void linkedListType::copyList(const linkedListType& otherList)
{
nodeType *newNode; //pointer to create a node
nodeType *current; //pointer to traverse the list
if (first != NULL) //if the list is nonempty, make it empty
destroyList();
if (otherList.first == NULL) //otherList is empty
{
first = NULL;
last = NULL;
count = 0;
}
else
{
current = otherList.first; //current points to the list to be copied
count = otherList.count;
//copy the first node
first = new nodeType; //create the node
first->info = current->info; //copy the info
first->link = NULL; //set the link field of the node to NULL
last = first; //make last point to the first node
current = current->link; //make current point to the next node
//copy the remaining list
while (current != NULL)
{
newNode = new nodeType; //create a node
newNode->info = current->info; //copy the info
newNode->link = NULL; //set the link of newNode to NULL
last->link = newNode; //attach newNode after last
last = newNode; //make last point to the actual last node
current = current->link; //make current point to the next node
}//end while
}//end else
}//end copyList
template
linkedListType::~linkedListType() //destructor
{
destroyList();
}//end destructor
template
linkedListType::linkedListType(const linkedListType& otherList)
{
first = NULL;
copyList(otherList);
}//end copy constructor
//overload the assignment operator
template
const linkedListType& linkedListType::operator=(const linkedListType& otherList)
{
if (this != &otherList) //avoid self-copy
copyList(otherList);
return *this;
}
unorderedLinkedList.h
#pragma once
//***********************************************************
// Author: D.S. Malik
//
// This class specifies the members to implement the basic
// properties of an unordered linked list. This class is
// derived from the class linkedListType.
//***********************************************************
#include "linkedList.h"
using namespace std;
template
class unorderedLinkedList: public linkedListType
{
public:
int search(const Type& searchItem) const;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is in the list,
// otherwise the value false is returned.
bool insertAt(int distance, const Type& insertItem);
//Function to insert newItem distance number of nodes from the
//beginning of the list.
//Postcondition: if location is greater than 0 and less than or equal
// to the number nodes in the list then first points to the first
// node, newItem is inserted distance number of nodes from the
// beginning of the list, last points to the last node, count is
// incremented by 1, and the function returns true
// else the function returns false
void insertFirst(const Type& newItem);
//Function to insert newItem at the beginning of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the beginning of the list, last points to
// the last node, and count is incremented by 1.
//
void insertLast(const Type& newItem);
//Function to insert newItem at the end of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the end of the list, last points to the
// last node, and count is incremented by 1.
bool deleteNode(const Type& deleteItem);
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing deleteItem
// is deleted from the list. first points to the first
// node, last points to the last node of the updated
// list, and count is decremented by 1.
bool deleteAt(int distance);
//Function to delete an item at a given distance from the list.
//Postcondition: If the distance is good, the node
// is deleted from the list. first points to the first
// node, last points to the last node of the updated
// list, and count is decremented by 1.
bool retrieveAt(int location, Type& retItem) const;
//Function to retrieve the element from the list at the
//position specified by location.
//Postcondition: retItem = list[location]
// If location is in range, function returns true
// else function returns false.
bool replaceAt(int location, const Type& repItem);
//Function to replace the element in the list at the
//position specified by location. The item to be replaced
//is specified by the parameter repItem.
//Postcondition: list node's item at location = repItem
// and function returns true.
//If location is out of range, function return false
};
template
bool unorderedLinkedList::replaceAt(int location, const Type& repItem)
{
if (location < 0 || count <= location) return false;
nodeType*current = first;
while (location > 0)
{
current = current->link;
location--;
}
current->info = repItem;
return true;
}
template
bool unorderedLinkedList::retrieveAt(int location, Type& retItem) const
{
if (location < 0 || count <= location) return false;
nodeType* current = first;
while (location > 0)
{
current = current->link;
location--;
}
retItem = current->info;
return true;
}
template
bool unorderedLinkedList::insertAt(int distance, const Type& insertItem)
{
if (distance < 0 || count < distance) return false;
nodeType* trailCurrent = NULL;
nodeType* current = first;
while (0 < distance)
{
trailCurrent = current;
current = current->link;
distance--;
}
nodeType* new_node = new nodeType;
new_node->info = insertItem;
new_node->link = NULL;
if (trailCurrent == NULL)
{
new_node->link = first;
first = new_node;
if (count == 0) last = first;
}
else if (trailCurrent == last)
{
trailCurrent->link = new_node;
last = new_node;
}
else
{
new_node->link = trailCurrent->link;
trailCurrent->link = new_node;
}
++count;
return true;
}
template
bool unorderedLinkedList::deleteAt(int distance)
{
if (distance < 0 || length() <= distance) return false;
nodeType *trailCurrent = NULL;
nodeType *current = first;
while (distance > 0)
{
trailCurrent = current;
current = current->link;
distance--;
}
if (current == first)
first = current->link;
else if (current == last)
{
last = trailCurrent;
last->link = NULL;
}
else
trailCurrent->link = current->link;
delete current;
count--;
if (count == 0)
first = last = NULL;
return true;
}
template
int unorderedLinkedList::search(const Type& searchItem) const
{
nodeType *current; //pointer to traverse the list
int distance = 0;
bool found = false;
current = linkedListType::first; //set current to point to the
// first node in the list
while (current != NULL && current->info != searchItem) //search the list
{
current = current->link; //make current point to
distance++;
}
if (current != NULL)
return distance;
else
return -1;
}//end search
template
void unorderedLinkedList::insertFirst(const Type& newItem)
{
nodeType *newNode; //pointer to create the new node
newNode = new nodeType; //create the new node
newNode->info = newItem; //store the new item in the node
newNode->link = first; //insert newNode before first
first = newNode; //make first point to the actual first node
count++; //increment count
if (last == NULL) //if the list was empty, newNode is also the last node in the list
last = newNode;
}//end insertFirst
template
void unorderedLinkedList::insertLast(const Type& newItem)
{
nodeType *newNode; //pointer to create the new node
newNode = new nodeType; //create the new node
newNode->info = newItem; //store the new item in the node
newNode->link = NULL; //set the link field of newNode
//to NULL
if (first == NULL) //if the list is empty, newNode is
//both the first and last node
{
first = newNode;
last = newNode;
count++; //increment count
}
else //the list is not empty, insert newNode after last
{
last->link = newNode; //insert newNode after last
last = newNode; //make last point to the actual
//last node in the list
count++; //increment count
}
}//end insertLast
template
bool unorderedLinkedList::deleteNode(const Type& deleteItem)
{
nodeType *current; //pointer to traverse the list
nodeType *trailCurrent; //pointer just before current
bool found = true;
if (first == NULL) //Case 1; the list is empty.
return false;
else
{
if (first->info == deleteItem) //Case 2
{
current = first;
first = first->link;
count--;
if (first == NULL) //the list has only one node
last = NULL;
delete current;
}
else //search the list for the node with the given info
{
found = false;
trailCurrent = first; //set trailCurrent to point to the first node
current = first->link; //set current to point to the second node
while (current != NULL && !found)
{
if (current->info != deleteItem)
{
trailCurrent = current;
current = current-> link;
}
else
found = true;
}//end while
if (found) //Case 3; if found, delete the node
{
trailCurrent->link = current->link;
count--;
if (last == current) //node to be deleted was the last node
last = trailCurrent; //update the value
//of last
delete current; //delete the node from the list
}
else
found = false;
}//end else
}//end else
return found;
}//end deleteNode
source.cpp
#include
#include
#include
#include
#include "arrayListType.h"
using namespace std;
int main()
{
default_random_engine dre(423);
uniform_int_distribution uid(10, 99);
int size = 16;
uniform_int_distribution uid1(-2, size + 5);
arrayListType a(size);
cout << a.listSize() << '/' << a.maxListSize() << endl;
while (!a.isFull())
{
a.insertEnd(uid(dre));
a.print(); cout << endl;
}
system("pause");
while (!a.isEmpty())
{
a.remove(uid(dre));
a.print(); cout << endl;
}
system("pause");
while (!a.isFull())
{
a.insertAt(uid1(dre), uid(dre));
a.print(); cout << endl;
}
system("pause");
while (!a.isEmpty())
{
a.removeAt(uid1(dre));
a.print(); cout << endl;
}
system("pause");
while (!a.isFull())
{
a.insertEnd(uid(dre));
a.print(); cout << endl;
}
system("pause");
for (int i = 0; i < a.maxListSize(); ++i)
{
a.replaceAt(i, i + 10);
a.print(); cout << endl;
}
system("pause");
cout << a.listSize() << '/' << a.maxListSize() << endl;
a.clearList();
cout << a.listSize() << '/' << a.maxListSize() << endl;
while (!a.isFull())
{
a.insertAt(uid1(dre), uid(dre));
a.print(); cout << endl;
}
system("pause");
for (int i = 0; i < a.listSize() / 2; ++i)
{
int t1, t2;
a.retrieveAt(i, t1);
a.retrieveAt(a.listSize() - i - 1, t2);
a.replaceAt(a.listSize() - i - 1, t1);
a.replaceAt(i, t2);
a.print(); cout << endl;
}
cout << a.listSize() << '/' << a.maxListSize() << endl;
a.clearList();
cout << a.listSize() << '/' << a.maxListSize() << endl;
system("pause");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
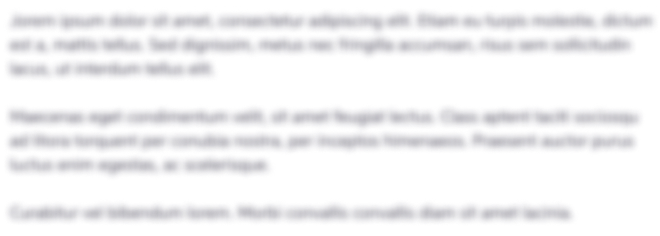
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started