Question
37.8 Advanced Java IO You are given a text file that contains six lines. Each line contains two floating point numbers followed by two integers.
37.8 Advanced Java IO
You are given a text file that contains six lines. Each line contains two floating point numbers followed by two integers. You will write a program that reads and writes this data using DataInputStream, DataOutputStream, RandomAccessFile, ObjectInputStream, and ObjectoutputStream.
Step 1) Create an ArrayList that will hold objects of the Holder class. Create a Scanner object wrapped around the text file in the variable textFileName. Each line of the file looks like
3.14159 24.4 100 42
That is, each line has two float value followed by two int values. Read the values on each line into variables and use use the variables to create a Holder object that is put into your ArrayList. Display the line
Data from Input.txt:
followed by the Holder object from your ArrayList. Submit your code. You should pass test 1.
Step 2) Create a File object with the string "MyFolder". Use the mkdir() method of File to create a folder with this name. Use the exists() and isDirectory() methods of file to test that your folder was created. If both methods return true, your program should display
MyFolder exists and is a directory.
Submit your code and you should pass test 2.
Step 3) Create a new FileOutputStream object using the string "MyFolder/Binary.dat" as the pathname. Wrap the file object in a DataOutputStream. Use the writeFloat() and writeInt() methods of DataOutputStream to write Holder objects to Binary.dat. The size of Binary.dat should be 96 bytes. The variables of the Holder class are a, b, c, and d. Variables a and b are float and variables c and d are integer. Write each Holder object variables in the order a, b, c, d. Use the get methods of the Holder class. Close the DataOutputStream. Submit your code. You should now pass test 3.
Step 4) Create a RandomAccessFile object using Binary.dat. Use the "rws" flag to ensure that the file is updated. Seek to byte 64 and read the next 16 bytes. The values you will read will be a two floats followed two integers, so you can use the RandomAccessFile methods readFloat() and readInt(). Add 20 to each of the values that you have written. Again, seek to byte 64. Now use the RandomAccessFile methods writeFloat() and writeInt() to update the RandomAccessFile. Submit your code. You should now pass test 4
import java.io.*; import java.util.*;
public class FileIOProg {
public static void main(String[] args) { /* Do not touch this code! */ String textFileName = "Input.txt"; if(args != null && args.length > 0) textFileName = args[0]; /* Do not touch the above code! */ /* Replace this comment with your code as directed by instructions */ } }
import java.io.*;
public class Holder implements Comparable
public int compareTo(Holder o) { int aDiffs = (int) (a - o.a); int bDiffs = (int) (b - o.b); int cDiffs = c - o.c; int dDiffs = d - o.d;
if(aDiffs != 0) return aDiffs; if(bDiffs != 0) return bDiffs; if(cDiffs != 0) return cDiffs; return dDiffs; } public String toString() { return "Holder [a=" + a + ", b=" + b + ", c=" + c + ", d=" + d + "]"; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
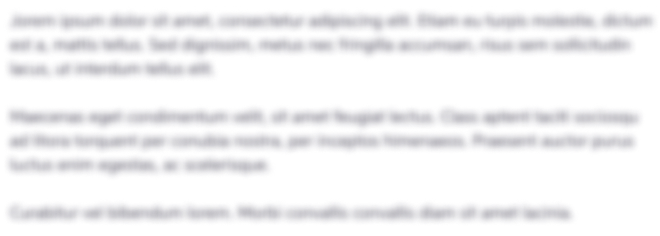
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started