Question
4 python programs: 1- Write a function 'music_func' that takes 3 parameters -- music type, music group, vocalist -- and prints them all out as
4 python programs:
1- Write a function 'music_func' that takes 3 parameters -- music type, music group, vocalist -- and prints them all out as shown in the example below. In case no input is provided by the user, the function should assume these values for the parameters: "Classic Rock", "The Beatles", "Freddie Mercury".
For example:
Input:
Alternative Rock,Pearl Jam,Chris Cornell
Output:
The best kind of music is Alternative Rock The best music group is Pearl Jam The best lead vocalist is Chris Cornell
Note: The print statements will go inside the music_func(). For example: print("The best kind of music is" + ...)
This the skeleton for 1:
#definition for music_func goes here
def main(): music, group, singer = input().split(',') music_func(music, group, singer) music_func() main()
2-
Write a function 'sort_list()' that accepts a list of integers and sorts it. The function should not explicitly 'return' this list and yet the list will be sorted when printed within main() after being passed to sort_list() as a parameter. Complete the main() module such that it accepts numbers from the user, until an empty string is entered, and stores them in a list called 'a_list'.
Input:
2
32
43
12
24
32
Output:
[2, 32, 43, 12, 24, 32] [2, 12, 24, 32, 32, 43]
Hint: Functions have the ability to modify mutable objects in the calling program. A 'list' is a mutable object. Read section 8.1.2 in the book.
This the skeleton for 2:
#sort_list() function goes here
def main(): #loop to accept integers until an empty string is entered goes here ######Do not modify this part###### print(a_list) sort_list(a_list) print(a_list) ######Do not modify this part###### ######main() ends here main()
3-
This program reads a file called 'test.txt'. You are required to write two functions that build a wordlist out of all of the words found in the file and print all of the unique words found in the file. Remove punctuations using 'string.punctuation' and 'strip()' before adding words to the wordlist.
Write a function build_wordlist() that takes a 'file pointer' as an argument and reads the contents, builds the wordlist after removing punctuations, and then returns the wordlist. Another function find_unique() will take this wordlist as a parameter and return another wordlist comprising of all unique words found in the wordlist.
Example:
Contents of 'test.txt':
test file another line in the test file
Output:
['another', 'file', 'in', 'line', 'test', 'the']
This the skeleton for 3:
#build_wordlist() function goes here
#find_unique() function goes here
def main(): infile = open("test.txt", 'r') word_list = build_wordlist(infile) new_wordlist = find_unique(word_list) new_wordlist.sort() print(new_wordlist) main()
4-
Write a function called 'game_of_eights()' that accepts a list of numbers as an argument and then returns 'True' if two consecutive eights are found in the list. For example: [2,3,8,8,9] -> True. The main() function will accept a list of numbers separated by commas from the user and send it to the game_of_eights() function. Within the game_of_eights() function, you will provide logic such that:
the function returns True if consecutive eights (8) are found in the list; returns False otherwise.
the function can handle the edge case where the last element of the list is an 8 without crashing.
the function prints out an error message saying 'Error. Please enter only integers.' if the list is found to contain any non-numeric characters. Note that it only prints the error message in such cases, not 'True' or 'False'.
Examples:
Enter elements of list separated by commas: 2,3,8,8,5 True
Enter elements of list separated by commas: 3,4,5,8 False
Enter elements of list separated by commas: 2,3,5,8,8,u Error. Please enter only integers.
Hint: You will need to use try-except to catch exceptions.
This is the skeleton for 4:
#game_of_eights() function goes here
def main(): a_list = input("Enter elements of list separated by commas: ").split(',') result = game_of_eights(a_list)
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
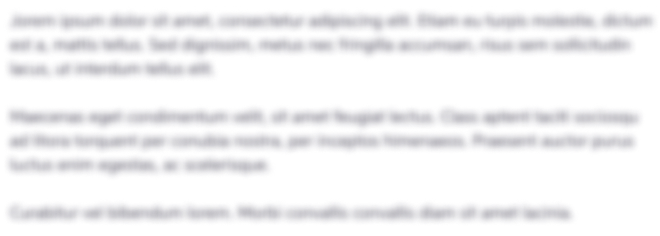
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started