Question
4. Suppose an external file is made up entirely of integers. In the model we've been using in this unit, the file is actually read
4. Suppose an external file is made up entirely of integers. In the model we've been using in this unit, the file is actually read in, line by line, as a sequence of Strings. Use String method split inside processLine() to produce individual tokens that look like numbers, but are actually Strings that are composed of digits. To convert each token from String format to integer format, you need to use the static parseInt() method from the Integer class. Thus int birthYear = Integer.parseInt("1983"); correctly stores the integer 1983 into the birthYear integer variable. For this assignment you should create a complete processLine method in the EchoSum class, so that it transforms each number in each line to integer format, and then sums the entries on the line and prints their sum on a separate line. For example, if the external text file looks like this:
1 2 3 4 3 4 7 1 11 2 3
your program should display this:
10 26 5
import java.io.*; public class EchoSum extends Echo { public EchoSum (String datafile) throws IOException { super(datafile); } // Prints the sum of each line. public void processLine(String line){
//code here
} //end method } //end class
5. For this problem we extend the Echo class to a class called EchoNoSpace. Here the external text file is written to the console as before, but now all spaces are removed first before lines are displayed. Thus a line like The best things in life are free becomes Thebestthingsinlifearefree The code for doing this uses split from the String class. The links below give the classes that participate in the solution, and the answer block below provides the implementation for the processLine() method in that derived class. The EchoNoSpace class has an addtional integer attribute, wordCount, which is initialized to 0 in the class constructor. After the file has been processed, this variable should hold the exact number of words (tokens) in the file. The EchoTester code at the link below reports this value as follows: System.out.println("wordCount: " + e.getWordCount()); For this assignment, edit the processLine() code in the answer box so that the EchoTester's main() method reports the correct value for the number of words in the file. Important: your code should count non-empty words only. Remember that split may place empty strings - strings of length 0 - in the array words, and thus your code must be able to ignore such entries.
import java.io.*; public class EchoNoSpace extends Echo { // the number of the words counted private int wordCount; public EchoNoSpace (String datafile) throws IOException { super( datafile); wordCount=0; } // Prints the given line without any spaces. // Overrides the processLine method in Echo class public void processLine(String line){
String result = "";
String[] words = line.split(" ");
for(String w : words)
{
result = result + w;
}
System.out.println(result);
} // returns the number of words in the file public int getWordCount(){ return wordCount; } //end method } //end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
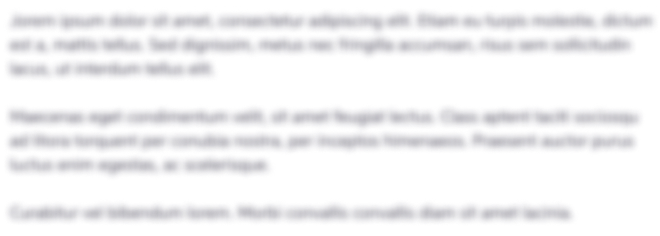
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started