Question
4) The following code is for Linear Algebra's Gaussian Elimination. A client with no technical background wants to know what is going on in this
4) The following code is for Linear Algebra's Gaussian Elimination. A client with no technical background wants to know what is going on in this code. How would you explain this code line-for-line?
package GaussElim;
import java.util.Scanner;
public class GaussianElimination
{
public void solve(double[][] A, double[] B)
{
int N = B.length;
for (int k = 0; k < N; k++)
{
//find pivot row
int max = k;
for (int i = k + 1; i < N; i++)
if (Math.abs(A[i][k]) > Math.abs(A[max][k]))
max = i;
// swap row in A matrix
double[] temp = A[k];
A[k] = A[max];
A[max] = temp;
// swap corresponding values in constants matrix
double t = B[k];
B[k] = B[max];
B[max] = t;
// pivot within A and B
for (int i = k + 1; i < N; i++)
{
double factor = A[i][k] / A[k][k];
B[i] -= factor * B[k];
for (int j = k; j < N; j++)
A[i][j] -= factor * A[k][j];
}
}
// Print row echelon form
printRowEchelonForm(A, B);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
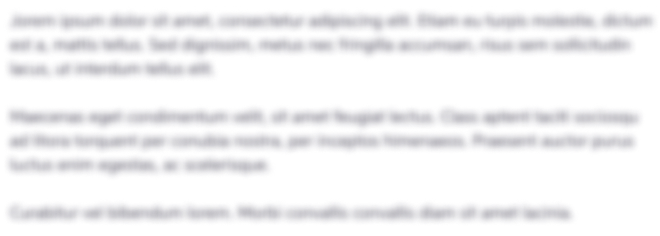
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started