Answered step by step
Verified Expert Solution
Question
1 Approved Answer
a) Add a students information (name and roll number). In HW7, the new student needs to be added to the head of the linked list.
studentList student *student *student "next *next *next null student name rollNumber courses name rollNumber courses name rollNumber courses null course name name name next *next null *next null studentList student *student *student "next *next *next null student name rollNumber courses name rollNumber courses name rollNumber courses null course name name name next *next null *next nulla) Add a students information (name and roll number). In HW7, the new student needs to be added to the head of the linked list. We do not ask user for courses to add in this function. So simply assign NULL to *courses member of the student node when a new student is added to the list in this function. (*courses is used in HW8) b) Display the student list. This function should print student's name and roll number. It should not print courses of the student. You must write a recursive function to implement this function. You may print the list forward or backward. See expected output for this function below. c) Search a student in the list by name. This function should return the student node if that student is found in the list, else return NULL. It is used as helper function in executeAction() to check if the student exists in the list. d) Remove the student from the list. This function removes the students name and roll number. a) Add a course to a students profile. This function assumes that the student is added in the list and assigns course name using the *courses member of student node. You may add the new course to the head or tail of the course linked list. (Sample solution adds the course to the tail) b) Display the last added course for a student. Add course option can be used multiple times to add multiple courses under a students profile. This function should return the most recently added course of the student. See expected output below. c) Display the list of students and their course list. This function should display students name, roll number and courses. See expected output below. (Display function in HW 7 did not display courses of the student). The removeStudent() of HW7 removed only the students name and roll number while removing the student form the list. In HW8, you need to modify the removeStudent() of HW7 to remove courses of the student as well when removing the student from the list. This is mentioned in the end of C file as HW8 Q4.
------------------------------------------------------------------------------------------------------ // READ BEFORE YOU START: // You are given a partially completed program that creates a linked list of student information, say for a school database. // The global linked list 'list' is a list of students with each node being struct 'studentList'. // 'studentList' consists of struct 'student' which has: student name, roll number, and a linked list of 'courses'. // The linked list of courses has each node containing simply the name of the course. // HW7 ignores the 'courses' linked list since there is no operation to be done on 'courses' in HW7. // HW8 has operations to be done with 'courses' list like add a course, display last added course. // To begin, you should trace through the given code and understand how it works. // Please read the instructions above each required function and follow the directions carefully. // If you modify any of the given code, the return types, or the parameters, you risk getting compile error. // You are not allowed to modify main (). // You can use string library functions. // ***** WRITE COMMENTS FOR IMPORANT STEPS OF YOUR CODE. ***** // ***** GIVE MEANINGFUL NAMES TO VARIABLES. ***** // ***** Before implementing any function, see how it is called in executeAction() ***** #include
#include #include #include // needed to use tolower() #pragma warning(disable: 4996) // for Visual Studio Only #define MAX_NAME_LENGTH 35 // global linked list 'list' contains the list of students struct studentList { struct student *student; struct studentList *next; } *list = NULL; // currently empty // structure "student" contains the student's name, library's name and linked list of courses struct student { char name[MAX_NAME_LENGTH]; unsigned int rollNumber; struct course *courses; }; // linked list of 'course' contains name of courses struct course { char name[MAX_NAME_LENGTH]; struct course *next; }; // forward declaration of functions (already implmented) void flushStdIn(); void executeAction(char); // functions that need implementation: // HW 7 void addStudent(char* studentNameInput, int rollNoInput); // 15 points void displayStudentList(struct studentList* tempList); // 10 points struct student* searchStudent(char* studentNameInput); // 10 points void removeStudent(char* studentNameInput); // 15 points //HW 8 void addCourse(char* studentNameInput, char* courseInput); // 15 points char* lastCourse(char* studentNameInput); // 15 points void displayStudentAndCourseList(struct studentList* tempList); // 10 points // edit removeStudent() // 10 points int main() { char choice = 'i'; // initialized to a dummy value do { printf(" CSE240 HW 7,8 "); printf("Please enter your selection: "); printf("HW7: "); printf("\t a: add a new student to the list "); printf("\t d: display student list (no courses) "); printf("\t r: remove a student "); printf("\t q: quit "); printf("HW8: "); printf("\t c: add course of a student "); printf("\t l: display last added course of a student "); printf("\t b: display student list including courses "); printf("\t q: quit "); choice = tolower(getchar()); flushStdIn(); executeAction(choice); } while (choice != 'q'); return 0; } // flush out leftover ' ' characters void flushStdIn() { char c; do c = getchar(); while (c != ' ' && c != EOF); } // Ask for details from user for the given selection and perform that action // Read the function case by case void executeAction(char c) { char studentNameInput[MAX_NAME_LENGTH], courseInput[MAX_NAME_LENGTH]; unsigned int rollNoInput; struct student* searchResult = NULL; switch (c) { case 'a': // add student // input student details from user printf(" Please enter student name: "); fgets(studentNameInput, sizeof(studentNameInput), stdin); studentNameInput[strlen(studentNameInput) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter roll number: "); scanf("%d", &rollNoInput); flushStdIn(); // if (searchStudent(studentNameInput) == NULL) // un-comment this line after implementing searchStudent() if (1) // comment out this line after implementing searchStudent() { addStudent(studentNameInput, rollNoInput); printf(" Student successfully added to the list! "); } else printf(" That student is already on the list! "); break; case 'd': // display the list displayStudentList(list); break; case 'r': // remove student printf(" Please enter student name: "); fgets(studentNameInput, sizeof(studentNameInput), stdin); studentNameInput[strlen(studentNameInput) - 1] = '\0'; // discard the trailing ' ' char //if (searchStudent(studentNameInput) == NULL) // un-comment this line after implementing searchStudent() if (0) // comment out this line after implementing searchStudent() printf(" Student name does not exist or the list is empty! "); else { removeStudent(studentNameInput); printf(" Student successfully removed from the list! "); } break; case 'c': // add course printf(" Please enter student name: "); fgets(studentNameInput, sizeof(studentNameInput), stdin); studentNameInput[strlen(studentNameInput) - 1] = '\0'; // discard the trailing ' ' char // if (searchStudent(studentNameInput) == NULL) // un-comment this line after implementing searchStudent() if (0) // comment out this line after implementing searchStudent() printf(" Student name does not exist or the list is empty! "); else { printf(" Please enter course name: "); fgets(courseInput, sizeof(courseInput), stdin); courseInput[strlen(courseInput) - 1] = '\0'; // discard the trailing ' ' char addCourse(studentNameInput, courseInput); printf(" Course added! "); } break; case 'l': // last course printf(" Please enter student name: "); fgets(studentNameInput, sizeof(studentNameInput), stdin); studentNameInput[strlen(studentNameInput) - 1] = '\0'; // discard the trailing ' ' char // if (searchStudent(studentNameInput) == NULL) // un-comment this line after implementing searchStudent() if (0) // comment out this line after implementing searchStudent() printf(" Student name does not exist or the list is empty! "); else { printf(" Last course added: %s ", lastCourse(studentNameInput)); } break; case 'b': // display student details and courses displayStudentAndCourseList(list); break; case 'q': // quit break; default: printf("%c is invalid input! ", c); } } // HW7 Q1: addStudent (15 points) // This function is used to insert a new student into the list. // You must insert the new student to the head of linked list 'list'. // You need NOT check if the student already exists in the list because that is taken care by searchStudent() called in executeAction(). Look at how this function is used in executeAction(). // Don't bother to check how to implement searchStudent() while implementing this function. Simple assume that student does not exist in the list while implementing this function. // NOTE: The function needs to add the student to the head of the list, unlike the previous HW. // NOTE: This function does not add courses to the student info. There is another function addCourse() in HW8 for that. // Hint: In this question, no courses means NULL courses. void addStudent(char* studentNameInput, int rollNoInput) { } // HW7 Q2: displayStudentList (10 points) // This function displays the student details (struct elements) of each student. // Parse through the linked list 'list' and print the student details (student name and roll no.) one after the other. See expected output screenshots in homework question file. // You should not display course names (because they are not added in HW7). // You MUST use recursion in the function to get full points. Notice that 'list' is passed to the function argument. Use recursion to keep calling this function till end of list. void displayStudentList(struct studentList* tempList) { } // HW7 Q3: searchStudent (10 points) // This function searches the 'list' to check if the given student exists in the list. Search by student name. // If it exists then return the 'student' node of the list. Notice the return type of this function. // If the student does not exist in the list, then return NULL. // NOTE: After implementing this fucntion, go to executeAction() to comment and un-comment the lines mentioned there which use searchStudent() // in case 'a', case 'r', case 'b', case 'l' (total 4 places) struct student* searchStudent(char* studentNameInput) { return NULL; // comment out this line when implementing the function // it is placed here to avoid compile error in empty function } // HW7 Q4: removeStudent (15 points) // This function removes a student from the list. // Parse the list to locate the student and delete the 'student' node. // You need not check if the student exists because that is done in executeAction() // NOTE: In HW 8, you will need to add code to this function to remove courses as well, when you remove the student. void removeStudent(char* studentNameInput) { struct studentList* tempList = list; // work on a copy of 'list' } // HW8 Q1: addCourse (15 points) // This function adds course's name to student info. // Parse the list to locate the student and add the course to the 'courses' linked list. No need to check if the student name exists on the list. That is done in executeAction(). // If the 'courses' list is empty, then add the course. If the student has existing courses, then you may add the new course to the head or the tail of the 'courses' list. // You can assume that the same course name does not exist. So no need to check for existing course names, like we do when we add new student. // NOTE: Make note of whether you add the course to the head of 'courses' list or the tail. You will need that info when you implement lastCourse() // (Sample solution has courses added to the tail of 'courses'. You are free to add new course to head or tail of 'courses' list.) void addCourse(char* studentNameInput, char* courseInput) { struct studentList* tempList = list; // work on a copy of 'list' } // HW8 Q2: lastCourse (15 points) // This function returns the name of the last (most recently) added course of a student. // Parse the list to locate the student. No need to check if the student name exists in the list. That is done in executeAction(). // Then parse the course names to return the most recently added course. // NOTE: Last course does not necessarily mean the last course in the 'courses' list. It means the most recently added course. // If you are adding courses to the head of the list in addCourse(), then you should return that course accordingly. char* lastCourse(char* studentNameInput) { struct studentList* tempList = list; // work on a copy of 'list' return NULL; // comment out this line when implementing the function // it is placed here to avoid compile error in empty function } // HW8 Q3: displayStudentAndCourseList (10 points) // This function displays every detail of each student, including courses. // Parse through the linked list and print the student details (student name, roll no., courses) one after the other. See expected output screenshots in homework question file. // Notice that 'list' is passed to the function as argument. // NOTE: You may re-use HW7 Q2 displayStudentList(list) code here. void displayStudentAndCourseList(struct studentList* tempList) { } // HW8 Q4: modify removeStudent (10 points) // In HW7, removeStudent() is supposed to remove student details like student name and roll number. // Modify that function to remove courses of the student too. // When the student is located in the 'list', after removing the student name and number of copies, parse the 'courses' list of that student // and remove the courses.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
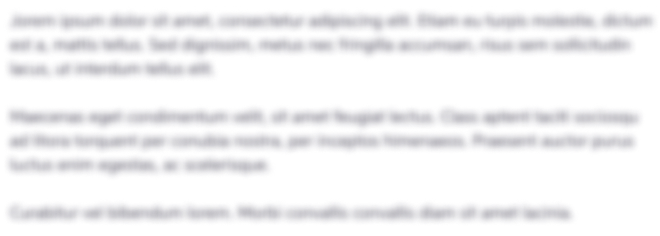
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started