Question
/** A class to keep track of a single appointment. */ public class Appointment { private String description; private int year; private int month; private
/** A class to keep track of a single appointment. */ public class Appointment { private String description; private int year; private int month; private int day;
/** Initializes appointment for a given date. @param year the year @param month the month @param day the day @param description the text description of the appointment */ public Appointment(int year, int month, int day, String description) { this.year = year; this.month = month; this.day = day; this.description = description; }
/** Returns the year of the appointment. @return the year */ public int getYear() { return year; }
/** Returns the month of the appointment. @return the month */ public int getMonth() { return month; }
/** Returns the day of the appointment. @return the day */ public int getDay() { return day; }
/** Determines if the appointment is on the date given. @param year the year to check @param month the month to check @param day the day to check @return true if the appointment matches all three parameters */ public boolean occursOn(int year, int month, int day) { return (year == this.year) && (month == this.month) && (day == this.day); }
/** Converts appointment to string description. */ public String toString() { return description; } }
/** Monthly appointment. */ public class Monthly extends Appointment { /** Initializes appointment for a given date. @param year the year @param month the month @param day the day @param description the text description of the appointment */ public Monthly(int year, int month, int day, String description) { //-----------Start below here. To do: approximate lines of code = 1 // Initialize the inherited variables - make sure of the super() keyword //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. }
/** Determines if the appointment occurs on the same day of the month. @param year the year @param month the month @param day the day @return true if day matches the appointment date and is later than the appointment date stored in this object */ public boolean occursOn(int year, int month, int day) { //-----------Start below here. To do: approximate lines of code = 5 // Override the occursOn() method. Check to see if the appointment occurs on the // same day of the month and is later than the appointment date stored in this object return (year == this.getYear()) && (month == this.getMonth()) && (day >= this.getDay()); //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } }
/** Daily appointment. */ public class Daily extends Appointment { /** Initializes appointment for a given date. @param year the year @param month the month @param day the day @param description the text description of the appointment */ public Daily(int year, int month, int day, String description) { //-----------Start below here. To do: approximate lines of code = 1 // Initialize the inherited variables - make use of the super() keyword super(year,month,day,description); //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. }
/** Determines if the date is later than the appointment date @param year the year @param month the month @param day the day @return true if base appointment is earlier than the appointment date */ public boolean occursOn(int year, int month, int day) { //-----------Start below here. To do: approximate lines of code = 9 // Override occursOn. Checks the given date parameters year, month, day to see if it is a later // date than the appointment date stored in this object. Return true if so, false otherwise //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } }
import java.util.Scanner;
/** Demonstration of the appointment classes */ public class AppointmentTester {
public static void main(String[] args) { Appointment[] appointments = new Appointment[2]; appointments[0] = new Daily(2000, 8, 13, "Brush your teeth"); appointments[1] = new Monthly(2003, 5, 20, "Visit grandma");
String dates0 = "2000 9 13 2000 6 13 2001 8 13 2000 8 14"; String dates1 = "2003 4 20 2003 7 20 2004 5 20 2002 5 20"; Scanner in = new Scanner(dates0); int year, month, day;
while (in.hasNextInt()) { year = in.nextInt(); month = in.nextInt(); day = in.nextInt();
if (appointments[0].occursOn(year, month, day)) { System.out.println(appointments[0]); } } System.out.println("Expected: Brush your teeth Brush your teeth Brush your teeth"); in = new Scanner(dates1); while (in.hasNextInt()) { year = in.nextInt(); month = in.nextInt(); day = in.nextInt(); if (appointments[1].occursOn(year, month, day)) System.out.println(appointments[1]); } System.out.println("Expected: Visit grandma Visit grandma"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
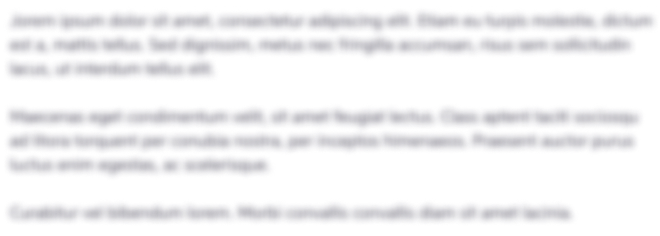
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started