Question
A. Modify your bag data structure such that in each element of array you store the head of a linked list (Instead of integer or
A.
Modify your bag data structure such that in each element of array you store the head of a linked list (Instead of integer or complex).
Modify the insert function to insert the value X in the linked list at the index X%capacity. How you modify the remove and find functions.
B.
Prevent from inserting two same integers in the bag.
C.
Modify your node to have an integer member variable "key". When you want to insert in the bag, you need to insert both key and value, however only key will be compared, the same thing applies for remove and find functions.
Data structure
That is the bag that needs modifying.
Thank you.
// Class Complex
// Data types are real of type int and imag of type int
//
// Complex()
// Precondition : Default Constructor to initialize objects
//
// Complex(int , int)
// Precondition : Parametarized Constructor to initialize objects
//
// GETTER METHODS
//
// int getReal()
// Precondition : Return the real part of the Complex number
//
// int getImag()
// Precondition : Return the imag part of the Complex number
//
// SETTER METHODS
//
// void setReal(int)
// Precondition : set the real part of the Complex number to the given arguement
//
// void getImag(int)
// Precondition : set the imag part of the Complex number to the given arguement
//
#ifndef MY_COMPLEX
#define MY_COMPLEX
#include
#include
using namespace std;
namespace myComplex
{
class Complex
{
int real;
int imag;
public:
Complex();
Complex(int, int);
int getReal();
int getImag();
void setReal(int);
void setImag(int);
};
}
#endif
-----------------Complex.cpp------------------------
#include "Complex.h"
using namespace myComplex;
Complex::Complex()
{
this->real = 0;
this->imag = 0;
}
Complex::Complex(int real, int imag)
{
this->real = real;
this->imag = imag;
}
int Complex::getReal()
{
return real;
}
int Complex::getImag()
{
return imag;
}
void Complex::setReal(int real)
{
this->real = real;
}
void Complex::setImag(int imag)
{
this->imag = imag;
}
----------------------Bag.h--------------------
// Class Bag
// Data types are arr of type Complex and index of type int
//
// Bag()
// Precondition : Default Constructor to initialize objects
//
// BAG(int)
// Precondition : Parametarized Constructor to initialize objects
//
// NORMAL METHODS
//
// bool search(Complex)
// Precondition : Return true if the given element is found
//
#ifndef MY_BAG
#define MY_BAG
#include "Complex.cpp"
namespace myBag
{
class Bag
{
// array of type Complex
Complex *arr;
// store the index of the last element in the array
int index;
public:
// constructor
Bag();
// constructor
Bag(int);
// method to add Complex object to Bag
void add(int , int);
// method to search for a complex number in the bag
bool search(Complex);
};
}
#endif
----------------Bag.cpp------------------------
#include "Bag.h"
using namespace myBag;
Bag::Bag()
{
this->arr = new Complex[10];
this->index = 0;
}
Bag::Bag(int size)
{
this->arr = new Complex[size];
this->index = 0;
}
void Bag::add(int real, int imag)
{
arr[index].setReal(real);
arr[index].setImag(imag);
//cout< index++; } bool Bag::search(Complex ob) { int i; for( i = 0 ; i < index ; i ++) { // if element is found if(ob.getReal() == this->arr[i].getReal() && ob.getImag() == this->arr[i].getImag()) return true; } // if element is not found return false; } ---------------main.cpp------------------ #include "Bag.cpp" int main() { Bag ob(50); int i; for(i=0;i<50;i++) { int real = rand() % 10 + 1; int imag = rand() % 10 + 1; ob.add(real, imag); } int r = rand() % 10 + 1; int im = rand() % 10 + 1; Complex temp(r, im); cout<<"Element to be searched : "<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
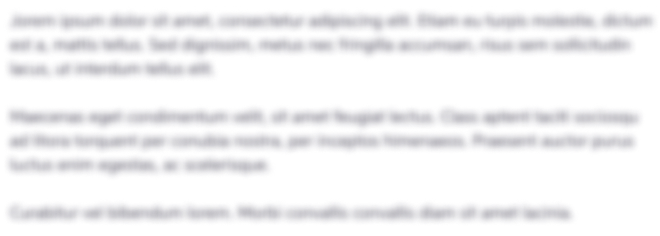
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started