Question
A password log file password_log_joe_bloggs.txt has been provided with the date/time and reason the password is invalid. Read the data from the file into a
A password log file password_log_joe_bloggs.txt has been provided with the date/time and reason the password is invalid. Read the data from the file into a list and output the list. Using the list, count the number of errors for passwords that were below the minimum length and count the number of passwords that were above the maximum length. Output the counts to the screen.
Steps:
Read the contents of the password log file and add this to a list
Output the contents of the list to the screen
Use the list to count of the number of errors for passwords that were below the minimum length and a count of the number of passwords that were above the maximum length.
Display both counts to the screen
Extra:
screen shots of the debugger window demonstrating correct errors by stepping & tracing through your code.
use:
count_pw_too_small
count_pw_too_large
modifying/re-writing the Python codebelow:
# importing module
import datetime
# constant variables declaration
PASSWORD_LOG_FILE = "password_log_joe_bloggs.txt"
MIN_PASSWORD_LENGTH = 6
MAX_PASSWORD_LENGTH = 10
# opening text file in write mode
File = open(PASSWORD_LOG_FILE, 'w')
# taking input for password
password = input("Enter password: ")
# looping until pass word length is less than 6 and greater than 10
while len(password) < 6 or len(password) > 10:
# getting current date and time
time = datetime.datetime.today()
if len(password) < 6:
reason = 'password < 6'
else:
reason = 'password > 10'
# writing into file time and reason
File.write(str(time)+"\t"+reason+" ")
# taking password again
password = input("Enter password: ")
# closing the text file
File.close()
# printing length of the password
print(" Length of the password is:",len(password))
# declaration of counting variables
letter_count = 0
digit_count = 0
# looping over password character by character
for char in password:
# if the character is alphabet then
if char.isalpha():
# incrementing the count of letter_count
letter_count += 1
# if the character is digit then
elif char.isdigit():
# incrementing the count of digit_count
digit_count += 1
# if the letter_count or digit_count is 0 then
if letter_count == 0 or digit_count == 0:
# printing the statement
print(" Weak password!")
# otherwise
else:
# printing the statement
print(" Strong Password! ")
# opening text file in read mode
File = open(PASSWORD_LOG_FILE, 'r')
# looping over text file line by line
for line in File:
# stripping the line to remove extra spaces at both ends
line = line.strip()
# if the line is not empty line then
if len(line) != 0:
# printing the line
print(line)
# closing the text file
File.close()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
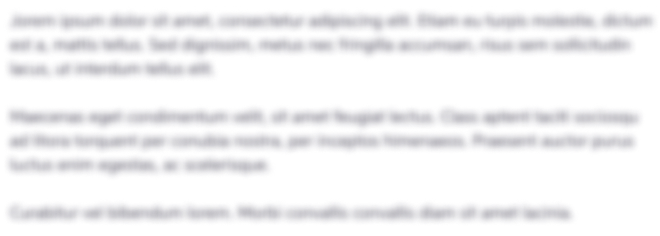
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started