Question
A Programming Exercise in Java . Please ensure your solutions work for JDK 11 ! Part 1 1. Look at the documentation on the String
A Programming Exercise in Java. Please ensure your solutions work for JDK 11!
Part 1
1. Look at the documentation on the String class.
2. Create a single public class JavaStrings which includes the three methods specified below. Your solution should use String methods.
3. You may include additional methods (e.g., main() or helper methods), but no methods beyond those specified need to be included, unless the requested methods depend on your new methods.
4. You may use additional methods included in the String class beyond those specified.
5. An example main() and output is provided below
These are the specified methods
Example Main and Output
Sample main (same as screenshot, included so you don't need to type it out)
public class JavaStrings {
public static void main(String args[]) {
JavaStrings myObject = new JavaStrings();
// Print out examples from addTogether.
String oneExample = "12 4 6789";
String twoExample = "abcdef gh";
int theLength = myObject.addTogether(oneExample,twoExample);
System.out.println(theLength);
// Length is unchanged by adding whitespace to front and back
oneExample = " " + oneExample + " ";
twoExample = "\t" + twoExample;
theLength = myObject.addTogether(oneExample,twoExample);
System.out.println(theLength);
// Print out example of idProcessing
String personFirst = "Dorothy";
String personLast = "Gale";
String petName = "Toto";
int petBirth = 1899;
String theID = myObject.idProcessing(personFirst,personLast,
petName,petBirth);
System.out.println(theID);
// Print out examples from secretCode
String ingredientOne = "tomato";
String ingredientTwo = "WATER";
String theCode = myObject.secretCode(ingredientOne);
System.out.println(theCode);
theCode = myObject.secretCode(ingredientTwo);
System.out.println(theCode);
}
}
Provides a method addTogether - Accepts two Strings as arguments - The method should trim the leading and trailing whitespaces from each String and add them together - Returns the length of the trimmed, concatenated String Provides a method idProcessing - A veterinary clinic needs to create unique identifiers for its animal clients. The identifier will consist of the owner's first and last initials, the first initial of the animal's name, and the pet's year of birth (e.g. EMD2010) - Write a method that will: + Accept three Strings and an integer as arguments (first name, last name, pet name, year) Returns the desired identifier as a String Provides a method secretCode - A famous pizzeria wants to encode its secret recipe. All ingredients are referred to using code words. Vowels (a, e, i, o, u) are replaced with a "z" and only the first three letters are used (e.g. tomato = tzmztz = tzm) - Write a method that will: + Accept a String as an argument Return the corresponding secret code String You may assume that all ingredients are at least three letters long Tip: Be sure to check the documentation for methods that might be helpful. Part 2 1. Consider your full solution to Part 1. 2. Add a private member variable SECRET_CODE_REGEX which is a Pattern object. 3. Create an associated static getter method getSecretCodeRegex. 4. Revise your solution so that the method secretCode uses SECRET_CODE_REGEX instead of String methods to perform the task. 5. The example main and output is the same as part 1 6. Provide your complete Java program that meets all the requirements from BOTH parts 1 and 2. Tip: Be sure to check the documentation for methods that might be helpful. Important: JDK 15 introduced text blocks which allow you to ignore the need to escape regular expressions in strings. Beware, if you are using a later version of Java, a regex may work on your machine, but not work in assessment, if you are not careful about escaping backslashes. Your code must be written with JDK 11 as the target! public class JavaStrings { public static void main(String args[]) { JavaStrings myObject = new JavaStrings(); // Print out examples from addTogether. String oneExample = "12 4 6789"; String twoExample = "abcdef gh"; int theLength = myObject.addTogether (oneExample, twoExample); System.out.println(theLength); = // Length is unchanged by adding whitespace to front and back oneExample = + one Example + " "; twoExample = "\t" + twoExample; theLength = myObject.addTogether (oneExample, twoExample); System.out.println(theLength); // Print out example of idProcessing String personFirst = "Dorothy"; String personlast = "Gale"; String petName = "Toto"; int petBirth = 1899; String theID = myObject.idProcessing(personFirst personLast, petName, petBirth); System.out.println(theID); // Print out examples from secretCode String ingredientOne = "tomato"; String ingredientTwo = "WATER"; String theCode = myObject. secretCode(ingredientOne); System.out.println(theCode); theCode = myObject. secretCode(ingredientTwo); System.out.println(theCode); = } } Output 18 2 18 Nm DGT1899 4. tzm 5 WZTStep by Step Solution
There are 3 Steps involved in it
Step: 1
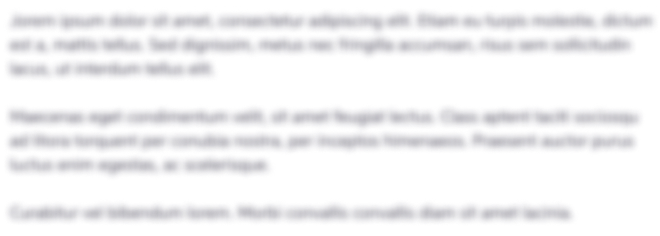
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started