Question
A skeleton code is provided for you to write a program which will calculate the Fibonacci sequence (0, 1, 1, 2, 3, 5, 8, .).
A skeleton code is provided for you to write a program which will calculate the Fibonacci sequence (0, 1, 1, 2, 3, 5, 8, .). The first n number will be shown as the output, where n will be given by the user. As the first two numbers (0 and 1) are predefined in a Fibonacci sequence, n must be >=3. Otherwise, the program will show an error message. The skeleton program uses two leaf procedures: print_number: this procedure prints an integer followed by a space character. add_two: this procedure does the addition of two integers, save the result in $s0, and return the value of $s0 to the caller. The two following restrictions are added so that you have some practice of using stack in the callee procedure. The input n must be stored in $s0 in the main program. The add_two procedure must use $s0 to save the result of the addition before returning its value to the caller.
#This is a MIPS program to print first n numbers fo the fibonacci sequence. n will be user input. #As the first two number of the sequence are given (0 and 1), n must be >=3 #Two restrictions: #$s0 must store the value of n in the main program. #$s0 must also be used as the register for storing the temporary/local result of the addition in the procedure .text main: # Print input prompt li $v0,4 # syscall code = 4 for printing a string la $a0, in_prompt # assign the prompt string to a0 for printing syscall # Read the value of n from user and save to t0 li $v0,5 # syscall code = 5 for reading an integer from user to v0 syscall ### Set s0 with the user input & if invalid (<=2) jumpt to exit_error ### ### Set proper argument register and call the procedure print_numnber to show the first two numbers of the sequence (0 & 1)### li $t0, 2 # initialize counter register t0 ### Load the first two numbers (0 & 1) to s1 & s2 ### loop: ### set the argument registers (a0 and a1) to the last two values in the sequence for addition, and then call proedure add_two### move $s1, $s2 # s1 now stores the last value in the sequence move $s2, $v0 # s2 now new value as returned from the addition procedure move $a0, $v0 # update a0 for printing the returned value jal print_number ### Increment the counter and compare it with user input. If equal, jumpt to exit.### j loop # Go to the start of the loop add_two: ### Push the value of s0 in the stack ### add $s0, $a0, $a1 # s0 now holds the result of the addition ### Set the register that will hold the reutrn value with the result of the addition ### ### Pop the value of s0 from the stack ### jr $ra # return to the caller # segement for printing an integer print_number: ### Write the syscall code for printing the integer ### # syscall for printing a space character li $v0,4 la $a0, space syscall jr $ra #return to the caller # exit block for wrong value of the input exit_error: li $v0, 4 # syscall code = 4 for printing the error message la $a0, error_string syscall li $v0,10 # syscall code = 10 for terminate the execution of the program syscall # exit block for the program exit: li $v0,10 # syscall code = 10 for terminate the execution of the program syscall .data in_prompt: .asciiz "how many numbers in the sequence you want to see (must be at least 3): " error_string: .asciiz "The number ust be greater than or equal to 3" space: .asciiz " "
Right now I have done some part of the code that if I input a number lower than 3 the program will print the error message. The problem is I cannot print out the answer after I input a value greater than 3
Here is the code I have.
#This is a MIPS program to print first n numbers fo the fibonacci sequence. n will be user input. #As the first two number of the sequence are given (0 and 1), n must be >=3 #Two restrictions: #$s0 must store the value of n in the main program. #$s0 must also be used as the register for storing the temporary/local result of the addition in the procedure
.text main: # Print input prompt li $v0,4 # syscall code = 4 for printing a string la $a0, in_prompt # assign the prompt string to a0 for printing syscall # Read the value of n from user and save to t0 li $v0,5 # syscall code = 5 for reading an integer from user to v0 syscall ### Set s0 with the user input & if invalid (<=2) jumpt to exit_error ### move $s0, $v0 # set the value of n in s0 li $t1, 2 # set the value of t1 as 2 bgt $s0, $t1, loop # jump to the loop if the value of n is bigger than 2 j exit_error ### Set proper argument register and call the procedure print_numnber to show the first two numbers of the sequence (0 & 1)### li $t0, 2 # initialize counter register t0 li $v0, 0 # set value of v0 as 0 jal print_number li $v0, 1 # set value of v0 as 1 jal print_number ### Load the first two numbers (0 & 1) to s1 & s2 ### li $s1, 0 # set value of s1 as 0 li $s2, 1 # set value of s2 as 1 loop: ### set the argument registers (a0 and a1) to the last two values in the sequence for addition, and then call proedure add_two### move $s1, $s2 # s1 now stores the last value in the sequence move $s2, $v0 # s2 now new value as returned from the addition procedure move $a0, $v0 # update a0 for printing the returned value jal print_number move $a0, $s1 # move the value of a0 to s1 move $a1, $s2 # move the value of a1 to s2 jal add_two ### Increment the counter and compare it with user input. If equal, jumpt to exit.### addi $t0, $t0, 1 # add counter beq $t0, $s0, exit # jump to exit if the value of counter is equal to n j loop # Go to the start of the loop
move $s1, $s2 # save the last value to s1 move $s2, $v0 # set s2 as the new value of addition procedure move $a0, $v0 # get the new value of v0 and print jal print_number j loop # Go to the start of the loop add_two: ### Push the value of s0 in the stack ### add $s0, $a0, $a1 # s0 now holds the result of the addition addi $sp, $sp, -4 # decrease the stack pointer sw $s0, 0($sp) # put the value of s0 into the stack ### Set the register that will hold the reutrn value with the result of the addition ### add $s0, $a0, $a1 ### Pop the value of s0 from the stack ### jr $ra # return to the caller # segement for printing an integer print_number: ### Write the syscall code for printing the integer ### # syscall for printing a space character li $v0,4 la $a0, space syscall jr $ra #return to the caller # exit block for wrong value of the input exit_error: li $v0, 4 # syscall code = 4 for printing the error message la $a0, error_string syscall li $v0,10 # syscall code = 10 for terminate the execution of the program syscall # exit block for the program exit: li $v0,10 # syscall code = 10 for terminate the execution of the program syscall .data in_prompt: .asciiz "how many numbers in the sequence you want to see (must be at least 3): " error_string: .asciiz "The number ust be greater than or equal to 3" space: .asciiz " "
Step by Step Solution
There are 3 Steps involved in it
Step: 1
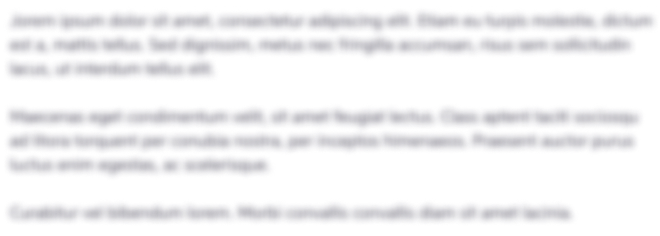
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started