Question
WRITE in C Take as input a list of numbers and reverse it. Intended approach: store the numbers in a deque (or a cardstack). Either
WRITE in C
Take as input a list of numbers and reverse it.
Intended approach: store the numbers in a deque (or a cardstack). Either extract the elements from the top and add it to a new queue, which will reverse the order, or traverse the list and switch the directions of the pointers.
Notice that simply exchanging the first and last pointers is not enough!
#include
#include
typedef struct s_card {
int cardvalue;
struct s_card *next;
struct s_card *prev;
} t_card;
typedef struct s_cardstack {
struct s_card *first;
struct s_card *last;
} t_cardstack;
t_cardstack *cardstackInit() {
t_cardstack *cardstack;
cardstack = malloc(sizeof(t_cardstack));
cardstack->first = NULL;
cardstack->last = NULL;
return cardstack;
}
int isEmpty(t_cardstack *cardstack) { return !cardstack->first; }
void pushFront(t_cardstack *cardstack, int cardvalue) {
t_card *node = malloc(sizeof(t_card));
node->cardvalue = cardvalue;
node->prev = NULL;
node->next = cardstack->first;
if (isEmpty(cardstack))
cardstack->last = node;
else
cardstack->first->prev = node;
cardstack->first = node;
}
void pushBack(t_cardstack *cardstack, int cardvalue) {
t_card *node = malloc(sizeof(t_card));
node->cardvalue = cardvalue;
node->prev = cardstack->last;
node->next = NULL;
if (isEmpty(cardstack))
cardstack->first = node;
else
cardstack->last->next = node;
cardstack->last = node;
}
int popFront(t_cardstack *cardstack) {
t_card *node;
int cardvalue;
if (isEmpty(cardstack))
return -1;
node = cardstack->first;
cardstack->first = node->next;
if (!cardstack->first)
cardstack->last = NULL;
else
cardstack->first->prev = NULL;
cardvalue = node->cardvalue;
free(node);
return cardvalue;
}
int popBack(t_cardstack *cardstack) {
t_card *node;
int cardvalue;
if (isEmpty(cardstack))
return -1;
node = cardstack->last;
cardstack->last = node->prev;
if (!cardstack->last)
cardstack->first = NULL;
else
cardstack->last->next = NULL;
cardvalue = node->cardvalue;
free(node);
return cardvalue;
}
int peekFront(t_cardstack *cardstack) {
if (isEmpty(cardstack))
return -1;
return cardstack->first->cardvalue;
}
int peekBack(t_cardstack *cardstack) {
if (isEmpty(cardstack))
return -1;
return cardstack->last->cardvalue;
}
void *fronttoback(t_cardstack *cardstack) {
if (isEmpty(cardstack))
return NULL;
t_card *currpointer = cardstack->first;
while (currpointer) {
printf("%d", currpointer->cardvalue);
currpointer = currpointer->next;
}
}
int main() {
int n;
scanf("%d", &n);
t_cardstack *firststack = cardstackInit();
for (int i = 0; i < n; i++) {
int x;
scanf("%d", &x);
pushBack(firststack, x);
}
// Method 1. Declare another stack and push elements
// out of the first stack into the other.
t_cardstack *revstack = cardstackInit();
// Method 2. Reverse the pointers within firststack.
return 0;
}
Step by Step Solution
3.42 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
include include typedef struct scard int cardvalue struct scard next struct scard prev tcard typedef ...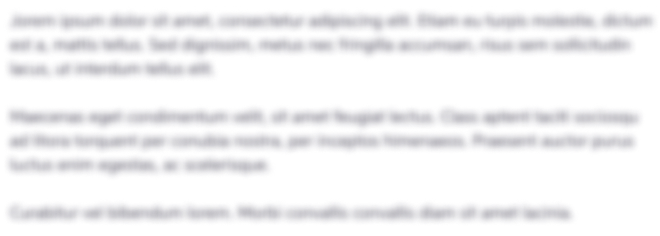
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started