Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Add a class named InvItem to this project, and add the properties, method, and constructors that are shown in the table above. Add code to
Add a class named InvItem to this project, and add the properties, method, and constructors that are shown in the table above. Add code to implement the New Item form Display the code for the New Item form and declare a classlevel InvItem variable named invItem with an initial value of null. Add a public method named GetNewItem that displays the form as a dialog box and returns an InvItem object. Add code to the btnSaveClick event handler that creates a new InvItem object and closes the form if the data is valid. Add code to implement the Inventory Maintenance form Display the code for the Inventory Maintenance form and declare a classlevel List variable named invItems with an initial value of null. Add a statement to the frmInvMaintLoad event handler that uses the GetItems method of the InvItemDB class to load the items list. Add code to the FillItemListBox method that adds the items in the list to the Items list box. Use the GetDisplayText method of the InvItem class to format the item data. Add code to the btnAddClick event handler that creates a new instance of the New Item form and executes the GetNewItem method of that form. If the InvItem object thats returned by this method is not null, this event handler should add the new item to the list, call the SaveItems method of the InvItemDB class to save the list, and then refresh the Items list box. Test the application to be sure this event handler works. Add code to the btnDeleteClick event handler that removes the selected item from the list, calls the SaveItems method of the InvItemDB class to save the list, and refreshes the Items list box. Be sure to confirm the delete operation. Then, test the application to be sure this event handler works. InvItemDBcs using System; using System.Collections.Generic; using System.Text; using System.Xml; namespace InventoryMaintenance public static class InvItemDB private const string Path @"InventoryItems.xml; public static List GetItems create the list List items new List; create the XmlReaderSettings object XmlReaderSettings settings new XmlReaderSettings; settings.IgnoreWhitespace true; settings.IgnoreComments true; create the XmlReader object XmlReader xmlIn XmlReader.CreatePath settings; read past all nodes to the first Book node if xmlInReadToDescendantItem create one Product object for each Product node do InvItem item new InvItem; xmlIn.ReadStartElementItem; item.ItemNo xmlIn.ReadElementContentAsInt; item.Description xmlIn.ReadElementContentAsString; item.Price xmlIn.ReadElementContentAsDecimal; items.Additem; while xmlInReadToNextSiblingItem; close the XmlReader object xmlIn.Close; return items; public static void SaveItemsList items create the XmlWriterSettings object XmlWriterSettings settings new XmlWriterSettings; settings.Indent true; settings.IndentChars ; create the XmlWriter object XmlWriter xmlOut XmlWriter.CreatePath settings; write the start of the document xmlOut.WriteStartDocument; xmlOut.WriteStartElementItems; write each product object to the xml file foreach InvItem item in items xmlOut.WriteStartElementItem; xmlOut.WriteElementStringItemNo Convert.ToStringitemItemNo; xmlOut.WriteElementStringDescription item.Description; xmlOut.WriteElementStringPrice Convert.ToStringitemPrice; xmlOut.WriteEndElement; write the end tag for the root element xmlOut.WriteEndElement; close the xmlWriter object xmlOut.Close; Programcs using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using System.Windows.Forms; namespace InventoryMaintenance static class Program
Add a class named InvItem to this project, and add the properties, method, and constructors that are shown in the table above.
Add code to implement the New Item form
Display the code for the New Item form and declare a classlevel InvItem variable named invItem with an initial value of null.
Add a public method named GetNewItem that displays the form as a dialog box and returns an InvItem object.
Add code to the btnSaveClick event handler that creates a new InvItem object and closes the form if the data is valid. Add code to implement the Inventory Maintenance form
Display the code for the Inventory Maintenance form and declare a classlevel List variable named invItems with an initial value of null.
Add a statement to the frmInvMaintLoad event handler that uses the GetItems method of the InvItemDB class to load the items list.
Add code to the FillItemListBox method that adds the items in the list to the Items list box. Use the GetDisplayText method of the InvItem class to format the item data.
Add code to the btnAddClick event handler that creates a new instance of the New Item form and executes the GetNewItem method of that form. If the InvItem object thats returned by this method is not null, this event handler should add the new item to the list, call the SaveItems method of the InvItemDB class to save the list, and then refresh the Items list box. Test the application to be sure this event handler works.
Add code to the btnDeleteClick event handler that removes the selected item from the list, calls the SaveItems method of the InvItemDB class to save the list, and refreshes the Items list box. Be sure to confirm the delete operation. Then, test the application to be sure this event handler works.
InvItemDBcs
using System;
using System.Collections.Generic;
using System.Text;
using System.Xml;
namespace InventoryMaintenance
public static class InvItemDB
private const string Path @"InventoryItems.xml;
public static List GetItems
create the list
List items new List;
create the XmlReaderSettings object
XmlReaderSettings settings new XmlReaderSettings;
settings.IgnoreWhitespace true;
settings.IgnoreComments true;
create the XmlReader object
XmlReader xmlIn XmlReader.CreatePath settings;
read past all nodes to the first Book node
if xmlInReadToDescendantItem
create one Product object for each Product node
do
InvItem item new InvItem;
xmlIn.ReadStartElementItem;
item.ItemNo xmlIn.ReadElementContentAsInt;
item.Description xmlIn.ReadElementContentAsString;
item.Price xmlIn.ReadElementContentAsDecimal;
items.Additem;
while xmlInReadToNextSiblingItem;
close the XmlReader object
xmlIn.Close;
return items;
public static void SaveItemsList items
create the XmlWriterSettings object
XmlWriterSettings settings new XmlWriterSettings;
settings.Indent true;
settings.IndentChars ;
create the XmlWriter object
XmlWriter xmlOut XmlWriter.CreatePath settings;
write the start of the document
xmlOut.WriteStartDocument;
xmlOut.WriteStartElementItems;
write each product object to the xml file
foreach InvItem item in items
xmlOut.WriteStartElementItem;
xmlOut.WriteElementStringItemNo Convert.ToStringitemItemNo;
xmlOut.WriteElementStringDescription item.Description;
xmlOut.WriteElementStringPrice Convert.ToStringitemPrice;
xmlOut.WriteEndElement;
write the end tag for the root element
xmlOut.WriteEndElement;
close the xmlWriter object
xmlOut.Close;
Programcs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace InventoryMaintenance
static class Program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
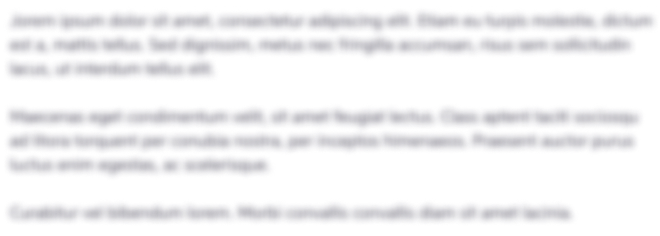
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started