Question
Add the addToHead(int data) method which is similar to addToTail(int data). I tried working around on this so you'll see the commented out method at
Add the addToHead(int data) method which is similar to addToTail(int data).
I tried working around on this so you'll see the commented out method at the bottom of the DList.java. Thanks!
********Class DList*********
package dlist; // add "add to head" public class DList { DNode head; DNode tail; int size; // how many nodes in the list public DList() { head = null; tail = null; size = 0; } /* Add a new node after the specified node */ public void addAfter(DNode node, int data) { DNode newNode = new DNode(data,node,node.getNext()); //newNode.setNext(node.getNext()); //newNode.setPrev(node); node.getNext().setPrev(newNode); node.setNext(newNode); } /* Add a node to the tail of the DList */ public DNode addToTail(int data) { /* case 1: List is empty */ if (head==null) { DNode node = new DNode(data,null,null); head = node; tail = node; size++; return node; } else // list is not empty { DNode node = new DNode(data,tail,null); DNode previousNode = tail; previousNode.setNext(node); tail = node; return node; } } /* public DNode addToHead (int data) { // Not sure if this node is the head or not // Starting from this node, go 'prev' till we reach head DNode currentHead = this; while (currentHead.prev != null) currentHead = currentHead.prev;
// Get a brand new node with prev=null, next= the current head of the list
// This uses the existing constructor DNode newNode = new DNode (data, null, currentHead); // Finally make the old head point its prev to the new node currentHead.prev = newNode; } */ }
********Class DListTest*********
package dlist;
public class DListTest { public static void main(String[] args) { // TODO Auto-generated method stub DList dlist = new DList(); // create an empty DList dlist.addToTail(5); printList(dlist.head); dlist.addToTail(10); printList(dlist.head); DNode node = dlist.addToTail(15); printList(dlist.head); dlist.addToTail(20); dlist.addAfter(node, 12); printList(dlist.head); printListBackwards(dlist.tail); }
public static void printList(DNode node) { // traverse list from head to tail for (DNode cursor = node; cursor != null; cursor = cursor.getNext()) { System.out.printf("%d ", cursor.getData()); } System.out.println(); }
public static void printListBackwards(DNode node) { // traverse list from tail to head for (DNode cursor = node; cursor != null; cursor = cursor.getPrev()) { System.out.printf("%d ", cursor.getData()); } System.out.println(); }
}
********Class DNode*********
package dlist;
public class DNode { private int data; private DNode prev; // points to the previous node in the DList private DNode next; // points to the next node in the DList public DNode(int data, DNode prev, DNode next) { this.setData(data); this.prev = prev; this.next = next; } public DNode getPrev() { return this.prev; } public void setPrev(DNode prev) { this.prev = prev; } public void setNext(DNode next) { this.next = next; } public DNode getNext() { return this.next; }
public int getData() { return data; }
public void setData(int data) { this.data = data; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
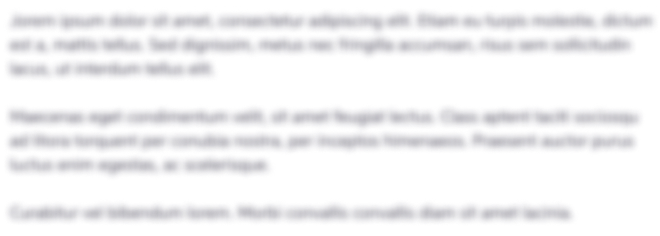
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started