Question
Your task is to create a priority queue class called PriQueue that is derived from the ArrayList class. Your class should be constructed as a
Your task is to create a priority queue class called PriQueue that is derived from the ArrayList class. Your class should be constructed as a Generic class so that the type of data the queue operates on can easily be changed:
PriQueue
PriQueue
Your priority queue should be sorted by priority based on a value from 1 to 10. If a number is assigned that is outside the range of 1 to 10 you should give the element a value of 5. What this means is that each time an element is added to the queue it should be put in order by priority. If multiple elements are given the same priority their position relative to each other is unimportant as long as they are prioritized relative to other elements in the queue.
Your PriQueue should have the following functionality:
- enqueue - Add an element to the queue
- dequeue - Remove element from the front of the queue
- peek - Return value at front of queue do not remove it
- size - Returns the number of items in the queue.
Specifics
You should create a classcalled QElem that will hold both the priority of the element and the data to be put in the queue. The classshould also be of a Generic type so that the value it holds can be of any type. The priority variable type should be an int.
The PriQueue class should be derived from ArrayList and should also be a Generic class so that it can operate on any type. The enquemethod should be adding a QElem so that the data and the priority are coupled. This needs to be done so that the data and priority are related for sorting. Here is an example:
PriQueue
que.enqueue("Hello", 3); que.enqueue("Goodbye", 9); // You are passing a string and an int but you should store a QElemclass.
String s = que.dequeue();
The String s at this point should hold "Goodbye" even though it was put in last because it has a higher priority.
Sorting your queue. You are to create your own sort functionality. Pick any sort that you would like but the bubble sort might be the easiest to implement. If you cannot find an example of a bubble sort, I will provide a link for you.
NOTE:
Your PriQueue class should be derived from the ArrayList class that is part of java.util.ArrayList
here is Pair code
package generics;
import java.util.Vector;
public class Pair
F first;
S second;
// Constructor
public Pair(F first, S second) {
this.first = first;
this.second = second;
}
// getter method of first
public F getFirst() {
return first;
}
// setter method of first
public void setFirst(F first) {
this.first = first;
}
// getter method of second
public S getSecond() {
return second;
}
// setter method of second
public void setSecond(S second) {
this.second = second;
}
// Format of printing a pair object
@Override
public String toString() {
return "
}
}
PairLIst.java code
package generics;
import java.util.Vector;
public class PairList
// method to add a pair to the vector
void addPair(T first, T second) {
add(new Pair(first, second));
}
// method to get first element given second
T getFirst(T second) {
for (Pair p : this) {
if (p.getSecond().equals(second)) {
return (T) p.getFirst();
}
}
return null;
}
// method to get second element given first
T getSecond(T first) {
for (Pair p : this) {
if (p.getFirst().equals(first)) {
return (T) p.getSecond();
}
}
return null;
}
// method to delete a pair
void deletePair(T first, T second) {
for (int i = 0; i < size(); i++) {
Pair p = get(i);
if (p.getFirst().equals(first) && p.getSecond().equals(second)) {
remove(p);
}
}
}
// method to print the vector of pairs.
void printList() {
for (Pair p : this) {
System.out.println(p);
}
}
}
and last test.code
package generics;
public class test {
public static void main(String[] args) {
PairList
coordinates.addPair(10, 10);
coordinates.addPair(20, 25);
coordinates.addPair(50, 35);
coordinates.addPair(40, 65);
coordinates.addPair(60, 30);
System.out.println("List of Pairs:");
coordinates.printList();
System.out.println("First of 25 = " + coordinates.getFirst(25));
System.out.println("Second of 65 = " + coordinates.getFirst(65));
coordinates.deletePair(50, 35);
System.out.println("Pairs after delete of <50, 35>");
coordinates.printList();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
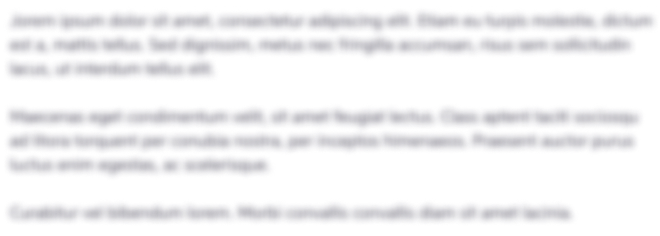
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started