Question
Add the following methods to this class and test them in a demo class called HeapDemo. T findMin() that returns the key with the smallest
Add the following methods to this class and test them in a demo class called HeapDemo.
T findMin() that returns the key with the smallest priority. For instance, if the heap is 90 80 60 20 70 10 15 5 9 50 the method returns 5.
T dequeueMin() that returns the key with the smallest priority and also deletes it. You can modify the sifting up operation to do this. For instance, if the heap is: 90 80 60 20 70 10 15 5 9 50 you would first find the smallest priority item using findMin(), then remove the last item (50)and put it in the place of 5. 90 80 60 20 70 10 15 50 9 Next sift 50 up until it finds the right spot. 90 80 60 50 70 10 15 20 9 If there are multiple keys with the minimum value, T dequeueMin() just removes one item.
import java.util.ArrayList; public class Heap> { ArrayList heapList; public Heap() { heapList = new ArrayList (); } public int size() { return heapList.size(); } public boolean isEmpty() { return heapList.isEmpty(); } public void clear() { heapList.clear(); } public void enumerate() { System.out.println(heapList); } public void add(T item) { heapList.add(item); int index = heapList.size()-1; int pindex = (index-1)/2; T parent = heapList.get(pindex); while (index>0 && item.compareTo(parent)>0) { heapList.set(index, parent); heapList.set(pindex, item); index = pindex; pindex = (index-1)/2; parent = heapList.get(pindex); } } public T deleteMax() { if (isEmpty()) { System.out.println("Heap is empty"); return null; } else { T ret = heapList.get(0); //get the item in the root. This is the largest item. T item = heapList.remove(heapList.size()-1); //remove the last item. if (heapList.size()==0) return ret; //if there was only one item in the heap to begin with, we are done. heapList.set(0, item); //otherwise, proceed. Put the item in the root. int index, lIndex, rIndex, maxIndex; T maxChild; boolean found=false; index = 0; lIndex = index*2+1; rIndex = index*2+2; while (!found) { if (lIndex 0) { maxChild = heapList.get(lIndex); maxIndex = lIndex; } else { maxChild = heapList.get(rIndex); maxIndex = rIndex; } //sift down if necesssary if (item.compareTo(maxChild)<0) { heapList.set(maxIndex, item); heapList.set(index, maxChild); index = maxIndex; } else found = true; } else if (lIndex < size()) //case 2: item to be sifted down has only left child //note: item to be sifted down cannot have only right child - it will violate the complete binary tree property { if (item.compareTo(heapList.get(lIndex))<0) { heapList.set(index, heapList.get(lIndex)); heapList.set(lIndex,item); index = lIndex; } else found = true; } else //case 3: item to be sifted down has no children found = true; lIndex = index*2+1; rIndex = index*2+2; } return ret; } }
public T findmin(){
//INPUT CODE HERE
}
public T dequeueMin(){
//INPUT CODE HERE
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
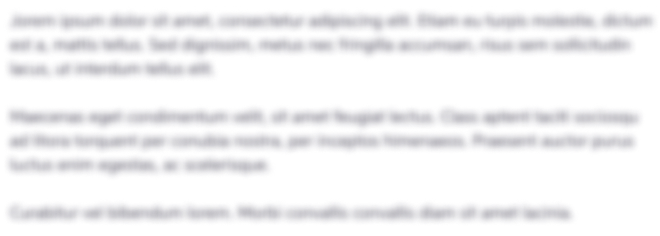
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started