Question
Add the three Java classes Applications, Rectangle and ArrayPractice as of your lab9 project to lab10 and copy over the codes from lab9 public class
Add the three Java classes Applications, Rectangle and ArrayPractice as of your lab9 project to lab10 and copy over the codes from lab9
public class Applications {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
//Declaring the ArrayPractice
ArrayPractice ap = new ArrayPractice();
ap.displayNumbers();
System.out.println(" ----------------- ");
ap.displayBoxes();
System.out.println(" ----------------- ");
ap.displayList();
//String names of my choice
String[] names={"Bob","Joe","Tim","Dan","Eli"};
System.out.println(" ------------------ ");
System.out.println(" ------------------- ");
//numbers are initialized to zeroes
// objects are initiaized to null
//ArrayPractice 2 with parametrized constructor
ArrayPractice ap2 = new ArrayPractice(50,6,names);
ap2.displayNumbers();
System.out.println(" ------------------------------ ");
ap2.displayBoxes();
System.out.println(" ------------------------------ ");
ap2.displayList();
//numbers are random numbers between -100 to 100
//length and width are random numbers between 0 to 1
// list of names are Bob, Joe, Tim, Dan, Eli as initialized above
}
}
public class ArrayPractice {
//Array declaration
private int []numbers;
private Rectangle [] boxes;
private String [] listOfNames;
int baseLength = 10;
ArrayPractice(){
numbers = new int[baseLength];
boxes = new Rectangle[baseLength];
listOfNames = new String[baseLength];
}
ArrayPractice(int k,int j,String[] a){
numbers = new int[k];
boxes = new Rectangle[j];
listOfNames = a;
loadBoxes();
loadNumbers();
}
//populates numbers array with randomly selected integer from range
private void loadNumbers() {
Random random = new Random();
int randomNumber;
for (int k = 0;k < numbers.length;k++){
randomNumber = random.nextInt(100 + 1 - (-100)) + (-100);
numbers[k] = randomNumber;
}
}
//populates the boxes array with Rectangles
private void loadBoxes() {
Random random = new Random();
int w,l;
for(int k = 0;k < boxes.length;k++)
{
w = random.nextInt();
l = random.nextInt();
boxes[k] = new Rectangle(w,l);
}
}
//prints the corresponding array entries
public void displayNumbers(){
for(int k=0;k System.out.println(numbers[k]); } } public void displayBoxes() { for(int k = 0;k < boxes.length;k++) { System.out.println(boxes[k]); } } public void displayList() { for(int k = 0;k < listOfNames.length;k++) { System.out.println(listOfNames[k]); } } } public class Rectangle { double length; private double width; public double getLength(){ return length; } public double getWidth(){ return width; } public void setLength(double length){ this.length = length; } public void setWidth(double width){ this.width = width; } public double computeArea(){ return length * width; } public double computePerimeter(){ return (length * 2) + (width * 2); } public String toString(){ return ("The length is: " + length + " and The width is: " + width); } public void displayRectangle(){ System.out.println(toString()); } public boolean equals(Rectangle other){ if (length == other.length && width == other.width){ return true; } else return false; } public Rectangle(double len, double w){ length = len; width = w; } public Rectangle(){ length = 0; width = 0; } } 2. (2pts)Add one more constructor to the Rectangle class, the so called copy constructor. This constructor also initializes the fields, but the values passed to the fields are the fields of another Rectangle object which already exists, and the other rectangle is the parameter : public Rectangle (Rectangle other){ length = other.length; width = other.width; } 3. (6pts) Add three new methods to the ArrayPractice class, each is meant to create and return a copy of an array field: copyNumbers( ), copyBoxes( ), copyList( ). Here is the specification with the code of the method that copies the array of names: //the method returns a String[ ] array, takes no parameter: public String[ ] copyList(){ //declare a local array variable and instantiate it to the length of listOfNames[ ] String[]clone = new String[listOfNames.length]; //populate clone with the copy of elements of listOfNames[ ] for(int k = 0; k< listOfNames.length; k++) clone[k] = new String(listOfNames[k]; //return clone return clone; } The copyBoxes( ) method follows the pattern above, you just must change the type, the method name and the array name (not the local variable clone). The copyNumbers( ) is also analogous, but populating the clone array is simpler. The numbers can directly be assigned to the clone entries, copy constructor does not apply for primitive types. 4. (6pts) Class Add three new accessor methods to the ArrayPractice class. However these getters do not directly return the fields, but rather a copy of the fields created by the corresponding copy method above. For instance, the getter for the numbers array is public int [ ] getNumbers(){ return copyNumbers(); } The other two getters are analogous. 5. For testing and demonstration purposes you add to the ArrayPractice class an ordinary accessor method as well, just for the boxes field, such that it directly returns boxes. Name this method getBoxesUnsafe( ). Applications class: test the code 6. In the main method of the Application class keep the list array and the ArrayPractice object instantiated by the parametrized constructor, delete the rest of the code. 7. (2pts)Call the getListOfNames( ) method, save the retuned array in a local String[ ] array named namesCopy and run a for loop to print its element to the console. Reading the output check if you see the elements of the list array defined in main. Print the bool-eans list[0]==namesCopy[0] and list[0].equals(namesCopy[0]) to the console and explain the result in a comment. 8. (4pts)Call the getBoxesUnsafe( ) and getBoxes( ) methods and save the retuned values in local variables named original and cloned, respectively. Run a for loop to print the elements of both arrays to the console, compare and comment. Print the booleans original[0]==cloned[0] and original[0].equals(cloned[0])to the console and explain the result in a comment. Change cloned[0] by the assignment cloned[0] = new Rectangle(1000,1000); Call again getBoxesUnsafe( ) and save it in original2, then print to the console original[0], original2[0] and cloned[0] and comment about the output. Change original[0] by the assignment original[0] = new Rectangle(2000,2000); Call again getBoxesUnsafe( ) and save it in original3, then print to the console original[0], original3[0] together with the boolean original[0]==original3[0] and comment about the output.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
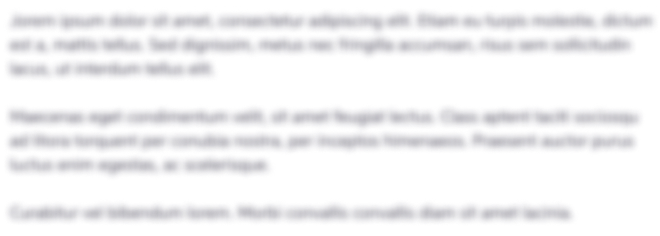
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started