Question
ADT queue: Circular array-based implementation. @file ArrayQueue.h */ #ifndef _ARRAY_QUEUE #define _ARRAY_QUEUE #include QueueInterface.h #include PrecondViolatedExcep.h const int MAX_QUEUE = 50; template < class ItemType>
ADT queue: Circular array-based implementation.
@file ArrayQueue.h */
#ifndef _ARRAY_QUEUE
#define _ARRAY_QUEUE
#include "QueueInterface.h"
#include "PrecondViolatedExcep.h"
const int MAX_QUEUE = 50;
template< class ItemType>
class ArrayQueue : public QueueInterface
{
private:
ItemType items[MAX_QUEUE]; // Array of queue items
int front; // Index to front of queue
int back; // Index to back of queue
int count; // Number of items currently in the queue
public:
ArrayQueue();
// Copy constructor and destructor supplied by compiler
bool isEmpty() const;
bool enqueue( const ItemType& newEntry);
bool dequeue();
/** @throw PrecondViolatedExcep if queue is empty. */
ItemType peekFront() const throw(PrecondViolatedExcep);
}; // end ArrayQueue
#include "ArrayQueue.cpp"
#endif
Use the following driver program with ArrayQueue structure from above.
#include
using namespace::std;
#include"ArrayQueue.h"
int main(){
ArrayQueue < int > myQueue1;
myQueue1.enqueue(56);
myQueue1.enqueue(25);
myQueue1.enqueue(32);
myQueue1.enqueue(76);
myQueue1.enqueue(35);
myQueue1.enqueue(40);
myQueue1.display();
myQueue1.dequeue();
myQueue1.display();
myQueue1.dequeue();
myQueue1.display();
myQueue1.enqueue(10);
myQueue1.enqueue(20);
myQueue1.display();
ArrayQueue< int > myQueue2(myQueue1);
myQueue2.display();
return 0;
}
Use ArrayQueue structure with const int MAX_QUEUE = 5.
Added the following member function display at the original structure.
template
void ArrayQueue::display() const{
int i,k;
i = front;
k = 0;
while(k < count){
cout << items[i] << ",";
i = (i+1) % MAX_QUEUE;
k++;
}//end while
cout << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
It looks like youve provided the implementation of an ADT Abstract Data Type queue using a circular arraybased structure along with a driver program t...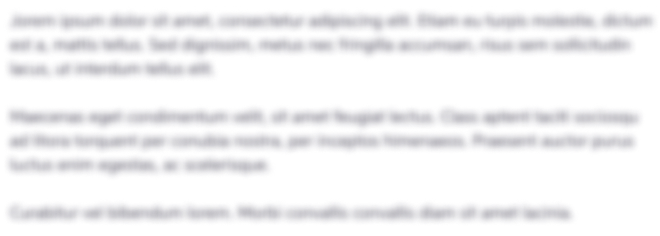
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started