Question
After writing a progam for each question, I need to check if all methods are working with TestNameClass.java, which contains a main method. 1.I'm struggling
After writing a progam for each question, I need to check if all methods are working with TestNameClass.java, which contains a main method.
1. I'm struggling to program a class called Name that represents a person's name. The class should have fields representing the person's first name, last name, and middle initial. (Your class should contain only fields for now.)
2. Add the following method to the Name class. Hint: it should be similar to the method - public String getNormalOrder()
public String getReverseOrder ( )
Returns the person's name in reverse order, with the last name preceding the first name and middle initial. For example, if the first name is "John", the middle initial is "Q", and the last name is "Public", returns "Public, John Q.".
3. I need help writing a toString method for the Name class that returns a String such as "John Q. Public".
4. Add a constructor to the Name class that accepts a first name, middle initial, and last name as parameters and initializes the Name object's state with those values.
5. Make its fields private and add methods called getMiddleInitial, and getLastName to your Name class. Hint: see the method - public String getFirstName()
6. Add methods called setMiddleInitial, and setLastName to your Name class. Give the parameters the same names as your class variables, and use the this keyword in your solution. Hint: see the method - public void setFirstName(String first)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Name.java
//
public class Name {
private String first, middle, last;
public Name(String first, String middle, String last) {
this.first = first;
this.middle = middle;
this.last = last;
}
// get normal order
public String getNormalOrder() {
return first + " " + middle + " " + last;
}
// get reverse order
public String getReverseOrder() {
return last + ", " + first + " " + middle;
}
//get first name
public String getFirstName() {
return first;
}
// get last name
public String getLastName() {
return last;
}
// get middle name
public String getMiddleName() {
return middle;
}
// set first name
public void setFirstName(String first) {
this.first = first;
}
// set last name
public void setLastName(String last) {
this.last = last;
}
// set middle initial
public void setMiddleName(String middle) {
this.middle = middle;
}
// toString method
public String toString() {
return first + " " + middle + " " + last;
}
}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
TestNameClass.java
//
public class TestNameClass {
public static void main(String args[]) {
Name n1 = new Name("James", "M.", "Bond");
Name n2 = new Name("Rebecca", "J.", "Smith");
System.out.println(n1);
System.out.println(n2);
System.out.println(n1.getNormalOrder());
System.out.println(n2.getReverseOrder());
n1.setFirstName("Jenny");
n1.setMiddleName("L.");
n1.setLastName("Lopez");
System.out.println(n1.getFirstName() + " " + n1.getMiddleName()
+ " " + n1.getLastName());
System.out.println(n2.getFirstName() + " " + n2.getMiddleName()
+ " " + n2.getLastName());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Below is the Java code for the Name class with the requested methods public class Name private String firstName private String middleInitial private String lastName Question 4 Constructor public NameS...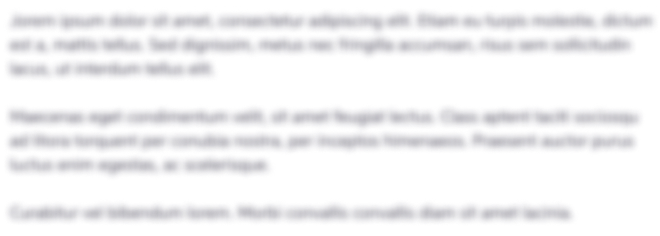
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started