Question
ArrayQueue.java public class ArrayQueue implements QueueInterface { // Do not add new instance variables. private T[] backingArray; private int front; private int size; /** *
ArrayQueue.java
public class ArrayQueue implements QueueInterface {
// Do not add new instance variables. private T[] backingArray; private int front; private int size;
/** * Constructs a new ArrayQueue. */ public ArrayQueue() {
}
/** * Dequeue from the front of the queue. * * Do not shrink the backing array. * If the queue becomes empty as a result of this call, you should * explicitly reset front to 0. * * You should replace any spots that you dequeue from with null. Failure to * do so can result in a loss of points. * * See the homework pdf for more information on implementation details. * * @see QueueInterface#dequeue() */
/** * Dequeue from the front of the queue. * * This method should be implemented in O(1) time. * * @return the data from the front of the queue * @throws java.util.NoSuchElementException if the queue is empty */
@Override public T dequeue() {
}
/** * Add the given data to the queue. * * If sufficient space is not available in the backing array, you should * regrow it to double the current length. If a regrow is necessary, * you should copy elements to the front of the new array and reset * front to 0. * * @see QueueInterface#enqueue(T) */
/** * Add the given data to the queue. * * This method should be implemented in (if array-backed, amortized) O(1) * time. * * @param data the data to add * @throws IllegalArgumentException if data is null */
@Override public void enqueue(T data) {
}
@Override public T peek() {
}
@Override public boolean isEmpty() { // DO NOT MODIFY THIS METHOD! return size == 0; }
@Override public int size() { // DO NOT MODIFY THIS METHOD! return size; }
/** * Returns the backing array of this queue. * Normally, you would not do this, but we need it for grading your work. * * DO NOT USE THIS METHOD IN YOUR CODE. * * @return the backing array */ public Object[] getBackingArray() { // DO NOT MODIFY THIS METHOD! return backingArray; } }
.
.
.
.
.
public interface QueueInterface{ /** * The initial capacity of a queue with fixed-size backing storage. */ public static final int INITIAL_CAPACITY = 11; /** * Dequeue from the front of the queue. * * This method should be implemented in O(1) time. * * @return the data from the front of the queue * @throws java.util.NoSuchElementException if the queue is empty */ T dequeue(); /** * Add the given data to the queue. * * This method should be implemented in (if array-backed, amortized) O(1) * time. * * @param data the data to add * @throws IllegalArgumentException if data is null */ void enqueue(T data); /** * Retrieves the next data to be dequeued without removing it. * * This method should be implemented in O(1) time. * * @return the next data or null if the queue is empty */ T peek(); /** * Return true if this queue contains no elements, false otherwise. * * This method should be implemented in O(1) time. * * @return true if the queue is empty; false otherwise */ boolean isEmpty(); /** * Return the size of the queue. * * This method should be implemented in O(1) time. * * @return number of items in the queue */ int size(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
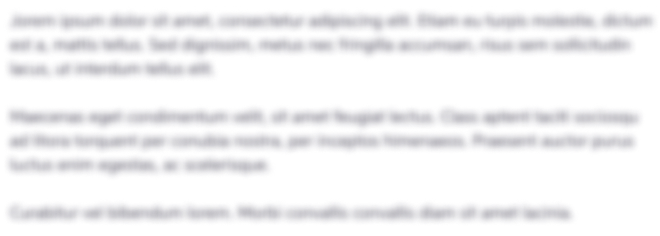
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started