Question
Arrays, Functions, Parameters, Statistics, Simple Classes Create a Form app P3Functions. Copy the following buggy method, and locate and fix the bug(s) such that it
Arrays, Functions, Parameters, Statistics, Simple Classes
- Create a Form app
P3Functions. Copy the following buggy method, and locate and fix the bug(s) such that it does what is described in the block comment;
//swapNumbers david beard Oct19
//FIND AND FIX THE BUG(S)
//swaps values in x and y.
public void swapNumbers(float x, float y)
{
int foo = x;
x = y;
y = foo;
}
For #2 through #10, create buttons and textboxes in the above form app to test the functions you create.
- Write a function called minIndex that accepts an integer array and returns the position of the minimum value.
- Write a function that accepts an integer array and return the second to the last occurrence of a target in the array. Return -1 if there are less than 2 values in the array.
- Write a function mean1DDoubleArray that accepts a 1dimensional array of doubles and determines the mean of all the values in the array. Your mean function should call a function that determines the sum.
- Implement the bubble sort as provided in code samples and notes for this assignment that sorts an array of integers. Provide appropriate code in your tester to show that it works correctly. Try it with array length 10, 100, 1000, and 10000 that are randomly filled with integers. What happens? (put your answers into the block comments for the bubble sort code.
- Create a function that accepts an array of integers a and returns the standard deviation of the values in a. The standard deviation is a statistical measure of the average distance each value in an array is from the mean . To calculate the standard deviation, determine the mean using a second function mean1DArrayOfInts. Then sum the square of the difference of each value in the array a and that mean. The standard deviation is the square root of that sum divided by the number of elements in the array. Note the discussion of standard deviation in the notes for this module.
- Create a function frequencyCount that given a value target, returns how many times target occurs in the array a.
- Create a method that returns the mode of the value in the array. The mode is the most frequently occurring value in the array . To make things easier, if two or more different values have the same frequency count, we will simply return the smallest. You may or may not find it easier to write the mode function if it calls frequencyCount.
- Create a function that accepts a 2 dimensional array of integers a and returns the number of rows that contain that integer.
- Create a function that accepts a 2 dimensional array of integers a and returns the sum of all the values on the outside rows and columns (do not add the corners twice.)
- Create a C# project
FlowOfControl in your project folder. Create a new class (under the project tab) named FlowOfControl be sure the first letter is capitalized as this is the naming convention for classes. Place the following code inside that class. It will replace the code that is already there:
/********************************************
* FlowOfControl - david beard
* used to show the flow of control from tester
* to class constructor and methods
* the output will be as follows:
* ***************************************/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace classFlowOfControl_v1
{
class FlowOfControl
{
/// constructor
/// we normally do not put writes in the class, but it helps us understand
public FlowOfControl()
{
Console.WriteLine("FLowOfControl:constructor");
}
///
/// foo - does nothing but show flow of control to method in a Class
/// note use of tripple / to create method block comments
/// foo (fu, phu, etc) is a generic meaningless name for things in code
/// professional code often has goo, foo, boo, zoo, soo, too, etc
/// this method is of type void as it does not return any value.
///
public void foo()
{
Console.WriteLine("FLowOfControl:foo");
}
}
}
In the solutions window, (use the gadget under file to display the solutions window if you can see it) rename program.cs to flowOfControlTester.cs. Place the following code in your flowOfControlTester. It will replace the code that is already there:
/**********************************************
* flow of contro9l tester - david beard
* output will be as follows:
* in tester - writeLine #1
* FLowOfControl:constructor
* in tester - writeLine #2
* FLowOfControl:foo
* in tester - writeLine #3
* ************************************************/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace classFlowOfControl_v1
{
class flowOfControlTester
{
static void Main(string[] args)
{
Console.WriteLine("in tester - writeLine #1");
// this method creates an instance of the FlowOfControl Class
// the constructor is executed so the writeLine will be executed.
FlowOfControl zoo = new FlowOfControl();
Console.WriteLine("in tester - writeLine #2");
// this statement executes the foo method of the zoo instance of the FlowOfControl class
// it executes the writeLine inside foo.
zoo.foo();
Console.WriteLine("in tester - writeLine #3");
}
}
}
Run your program and make sure its output is the same as what is described in the block comment.
Rubric: 9 points each. Style: -20. Block comments for each method and for the class including your name, the date, and one great sentence without ands and ors. Use great variable names. If you borrow code from someone else, include the appropriate attributions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
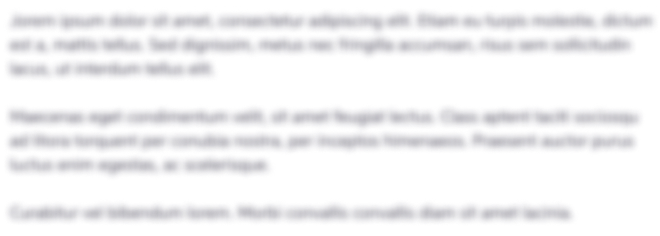
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started