Question
Assignment 1 BCS 370 Data Structures Due: 9/20/2017 @ 1:40pm You must submit all projects necessary to run this assignment. Zip the whole project directory
Assignment 1 BCS 370 Data Structures
Due: 9/20/2017 @ 1:40pm
You must submit all projects necessary to run this assignment. Zip the whole project directory of every project that is necessary to run it.
Use good style. Make sure that you properly separate code into .h/.cpp files. Make sure that you add preprocessor guards to the .h files to allow multiple #includes.
IMPORTANT Make sure you properly comment your programs. Download the commenting document from Blackboard. It is located in the Handouts folder. If you do not properly document your programs I will deduct points.
Overview
You will be writing the classes Stock and Portfolio. You will be writing unit testing code for both classes.
Part 1 Create a Stock Class
Write a class named Stock.
Stock Class Specifications
Include member variables for name (string), price (double), shares (double).
Write a default constructor.
Write a constructor that takes values for all member variables as parameters.
Write a copy constructor.
Implement Get/Set methods for all member variables.
Implement the CalculateValue function. This function should multiply the prices by the shares and return that value. Use the following function header: double CalculateValue().
Add a member overload for the assignment operator.
Add a non-member operator<< overload. Prints the values of all member variables on the given ostream.
Part 2 Create a Portfolio Class
Write a class that will store a collection of Stock. This class will be used to keep track of data for multiple Stock class instances. You MUST implement ALL of the specifications below.
Portfolio Class Specifications
Create a private member variable that is a static array of Stock. The size of the array can be whatever you want it to be.
Your class must implement all of the following functions (use the given function prototypes):
void Set(int index, Stock s) Sets the value at the given index to the given Stock instance. You should test the index to make sure that it is valid. If the index is not valid then do not set the value.
Stock Get(int index) Return the Stock located at the given index in the array.
int PriceRangeCount(double lowerBound, double upperBound) Returns the count of the number of Stocks that fall within the given range. For example, assume the following number of Stock prices: 10, 20, 15, 25, 30, 40
If lowerBound is 20 and upperBound is 30 then the returned value should be 3. Any values that fall on the boundaries should be included in the count. In this example we are getting a count of the number of stocks that have a price between $20 and $30. Remember, this function is using price and not value.
Stock MostShares() Returns the Stock in the Portfolio that has the most shares.
bool FindByName(string name, Stock &v) Returns true if the Stock with the given name is in the array and false otherwise. If the Stock is in the array you should copy it into the Stock reference parameter.
double TotalValue() Returns the sum of all Stock values (not prices) in the collection.
int Size() Returns the size of the array.
void Initialize() Initializes all of the elements of the array to reasonable default values.
string GetAuthor() Returns your name. Just hard code your name into the function.
Create a default constructor that will initialize all elements of the array to default values.
Part 4 Main Function
Main should create instances of Stock and Portfolio and contain an automated unit test for both of them.
Automated Test
Use the Automated Testing slides on Blackboard as a guide when coding this section.
Create an automated unit test in main. You need to write code to automatically test that both classes work properly. The automated test should contain code to unit test the following functions:
All Stock get/set functions.
Portfolio Get.
Portfolio Set.
Portfolio PriceRangeCount.
Portfolio MostShares.
Portfolio FindByName.
Portfolio TotalValue.
DO NOT TAKE THE TESTING CODE LIGHTLY. IT IS VERY IMPORTANT TO SHOW THAT EVERYTHING ON EACH CLASS WORKS.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
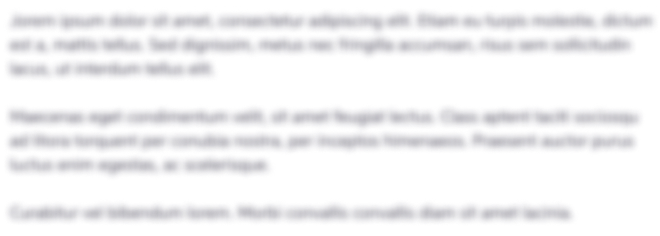
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started