Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment 5: Given: 02/04/21 Due: 02/19/21 Purpose: Create a Java class from scratch. Create a class in Java like the ur_Robot class in Karel++. Description:
Assignment 5:
Given: 02/04/21 Due: 02/19/21 Purpose: Create a Java class from scratch. Create a class in Java like the ur_Robot class in Karel++. Description: Karel++ is a simple programming language that illustrates Object-oriented programming concepts. Your job is to create Java code to mimic the Karel++ language. -- This is a major goal of Object-oriented -- -- programming: -- -- "Make the language closely match the problem- -- -- space you are working in." -- So if your ur_Robot class is well-designed, it should mimic Karel++ ur_Robots well. Look at the Karel++ overview posted on Otto for a brief summary of what these Karel++ robots are. { If you are interested in a complete description of Karel++ find a copy of the Karel++ book by Bergin. Used copies are available from Amazon and from Alibris for under $10. } Allow the five primitive instructions/messages to be sent to an ur_Robot and have its state adjusted properly. Karel++ - like ur_Robot definition: class ur_Robot { void move( ); <- These must be present void turnLeft( ); ...as in Karel++. void pickBeeper( ); void putBeeper( ); void turnOff( ); } Write a program that creates at least one of these robots and exercises it by giving it the five primitive instructions. Display its state before and after it receives the instructions to illustrate that it is functioning. Introduce some print()/display() method(s) so that a robot can display it's current state when requested. There will be no world for the program to function in or run against. So you do not need to worry about errors hitting walls (except world boundaries, which are always present) or picking up beepers. A robot should be sensitive to beepers in its bag and to the fact that it cannot put down beepers that it does not have. Note: ---- There are several things that will need to be different from Karel++ in this Java version of an ur_Robot class: 1) a "task" will be a main() 2) the four directions might not be represented by the words 'East','West','North','South', but could be 3) there will be no world (for now) 4) no graphics (for now) - we just want a model for ur_Robot - graphics will come later Use reasonable datatypes wherever possible. (Build some if you need them.) Start small. Ex: Build a robot that can move( ). - Get something working soon! Then refine it and expand it - Don't be afraid to redesign (It's how you improve yourself and your code.) - Have a previous working model to retreat to at all times! - Use the knowledge and topics we have learned. Submit your source code to otto. * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * Ex: The following code is an example of what a Java program -- might look like with a Java ur_Robot class . (Note that it does look a lot like an actual Karel++ program. ) . . . public static void main(String args[]) { ur_Robot karel = new ur_Robot(1,1,3,0); karel.move(); karel.move(); karel.turnLeft(); karel.move(); karel.pickBeeper(); karel.pickBeeper(); karel.pickBeeper(); karel.putBeeper(); karel.turnOff(); } OR// public static void main(String args[]) { ur_Robot karel = new ur_Robot(1,1,3,0); System.out.println("Initial position:"); karel.display(); System.out.println(karel); karel.move(); karel.move(); System.out.println(); System.out.println("After 2 moves:"); karel.display(); karel.turnLeft(); karel.move(); System.out.println(); System.out.println("After a turnLeft and a move:"); karel.display(); karel.pickBeeper(); karel.pickBeeper(); karel.pickBeeper(); karel.putBeeper(); karel.turnOff(); System.out.println(); System.out.print("After some beeper activity "); System.out.println("and a turnOff:"); karel.display(); } . . . /* Output: Initial position: Robot: Location: 1 st, 1 ave Direction: East Beepers in bag: 0 On/Off: on ===================================== St: 1, Ave: 1, Dir: East, Bag: 0, on After 2 moves: Robot: Location: 1 st, 3 ave Direction: East Beepers in bag: 0 On/Off: on ===================================== After a turnLeft and a move: Robot: Location: 2 st, 3 ave Direction: North Beepers in bag: 0 On/Off: on ===================================== After some beeper activity and a turnOff: Robot: Location: 2 st, 3 ave Direction: North Beepers in bag: 2 On/Off: off ===================================== */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
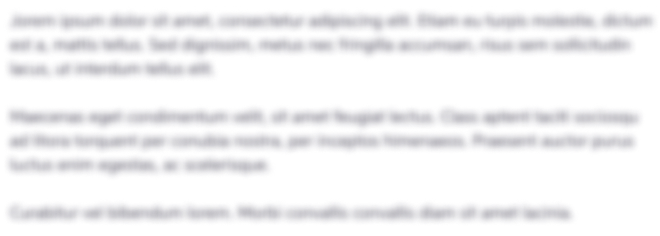
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started