Question
Assignment #6 Introduction to C Programming COP 3223 Objectives 1. To learn how to use 2 dimensional arrays to store and retrieve data to help
Assignment #6 Introduction to C Programming COP 3223
Objectives 1. To learn how to use 2 dimensional arrays to store and retrieve data to help solving problems.
Introduction: Ninja Academy Ninjas are awesome! Your friend has not stopped talking about how cool ninjas and how they would like to become a ninja. To amuse your friend, you have decided to create a series of programs about ninjas.
Problem: Stolen! (ninjamission.c) The Ninja Academy has been raided by a rival ninja school! Some of the Academys most valuable relics have been stolen: 0) The founders KATANA 1) A leather scroll CASE 2) An ornate SCROLL 3) A velvet POUCH 4) A JADE statue 5) An ancient MASK The Ninja Academy is nominating its best students to go on a mission to retrieve these items. Each you will take turns trying to steal back a relic, simulated by a randomly generated number from 0 to 6. Six of those numbers (0-5) correspond to the numbers above, while 6 corresponds to getting caught. Getting caught by the rival schools guards will force you to give back a relic youve already retrieved. If you get a number that corresponds to an item you already have, nothing happens. If you get a SCROLL (item 2), you may only pick up the item if you already have a CASE (item 1). If you get a JADE (item 4) or a MASK (item 5), you may only pick up the item if you already have a POUCH (item 3) to put them in. This is because you cannot carry the scroll with a scroll case and you cannot carry the jade stature or the mask with a velvet pouch to keep them from getting damaged. If you land on Youve been caught!, then you must choose which relic to discard. If you have no items to begin with, then you dont lose anything. If you have a SCROLL (2), then you cannot discard the CASE (1) and if you have a JADE (4) or a MASK (5), then you cannot discard the POUCH (3). The first person to retrieve all six items will be a hero of the Academy! Program Specification You must use a 2D array to solve the problem. There will be no more than 6 ninja trying to complete the mission. Sample Data Items You may wish to use these data structures to help you solve the problem. Type them into Code::Blocks (do not copy/paste). int cont = 1; int ninjas[6][6]; char ITEMS[6][20] = {"KATANA", "CASE", "SCROLL", "POUCH", "JADE", "MASK"}; The values in ITEMS are strings and may be printed with %s. For example, to print CASE we could use printf(%s, ITEMS[1]); Program Format Beginning the program Start by asking how many ninjas will be sent on the mission. Beginning of Each Turn Each turn should begin with a prompt with the following format: Ninja X, it is your turn. Enter 0 to look for an item. where X is the number given to the current ninja, starting at 1. (Note: Internally, the player numbers will start at 0, so when you print this out, just add 1 to the variable storing the player number.) Finding an Item After the random number is generated, check what it corresponds to. If a value other than 6 (Youve been caught!) is generated, a statement of the following form should be printed: Ninja X, you found a S. where S is the name of the item the number matches. If the player does not yet have the item and is allowed to obtain it, follow this with a statement of the form: You now have a S! If the player is not allowed to obtain the item because they dont yet have another necessary item, print out a statement of the form: Sorry, you cant obtain a S. If the player lands on a square for a piece they already have, then print the following: You already have that item. Landing on Youve been caught! If the player gets this number they must choose a piece to lose. First print the warning: Youve been caught! If the player has no pieces to lose, then print the following: There is no equipment for you to lose. Lucky you, sort of. If they have any items, prompt them with the question: Which piece would you like to lose? Follow this with a numbered list of items that are valid for the player to discard. (Namely, this list will NOT contain the case if the rider has a scroll and it will not contain the pouch if the rider has a mask or a jade) The numbering of the list should correspond to the numbering of each item listed on page 1. Thus, the numbering wont necessarily be sequential. For example, if the possible pieces a player can lose are the katana, case, scroll, and pouch, the print out would appear as follows: 0. KATANA 2. SCROLL 3. POUCH You may assume the user will select a valid item. Then, print out a message of the following format: You have selected the S to discard. where S is the item to discard. Final Printout for Each Turn At the end of each persons turn, print out a complete list of their items. The first line will be: Ninja X, here is what you currently have: Follow this with a blank line and then a numbered list (as previously described), with each item the player currently has. End of the Game The game ends when one person has all the items. If you are using the sample data structure cont, then you can use this as a flag for continuing the game or not. The default is 1 for continuing the game. When a person has all the items, change the flag to 0. At the end of the game, simply print a message with the following format: Congratulations Ninja X, you are the first to return with all of the stolen items! Output Sample Sample outputs will be provided on the webcourse. Deliverables One source file: ninjamission.c for your solution to the given problem submitted over WebCourses. Restrictions Although you may use other compilers, your program must compile and run using Code::Blocks. Your program should include a header comment with the following information: your name, course number, section number, assignment title, and date. Also, make sure you include comments throughout your code describing the major steps in solving the problem. Grading Details Your programs will be graded upon the following criteria: 1) Your correctness 2) Your programming style and use of white space. Even if you have a plan and your program works perfectly, if your programming style is poor or your use of white space is poor, you could get 10% or 15% deducted from your grade. 3) Compatibility You must submit C source files that can be compiled and executed in a standard C Development Environment. If your program does not compile, you will get a sizable deduction from your grade.
Here is an example of the output
How many ninjas were sent on the mission? 2 Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a MASK. Sorry, you can't obtain a MASK. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a MASK. Sorry, you can't obtain a MASK. Ninja 2, here is what you currently have: Ninja 1, it is your turn. Enter 0 to look for an item. 0 You've been caught! There is nothing for you to lose. Lucky you, sort of. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a POUCH. You now have a POUCH! Ninja 2, here is what you currently have: POUCH Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a KATANA. You now have a KATANA! Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a JADE. You now have a JADE! Ninja 2, here is what you currently have: POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a KATANA. You already have that item. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 2, here is what you currently have: POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a MASK. Sorry, you can't obtain a MASK. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 2, here is what you currently have: POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a KATANA. You already have that item. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 2, here is what you currently have: POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a JADE. Sorry, you can't obtain a JADE. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a CASE. You now have a CASE! Ninja 2, here is what you currently have: CASE POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a CASE. You already have that item. Ninja 2, here is what you currently have: CASE POUCH JADE Ninja 1, it is your turn. Enter 0 to look for an item. 0 You've been caught! Which piece would you like to lose? 0. KATANA 0 You have selected the KATANA to discard. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 You've been caught! Which piece would you like to lose? 1. CASE 4. JADE 4 You have selected the JADE to discard. Ninja 2, here is what you currently have: CASE POUCH Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a MASK. You now have a MASK! Ninja 2, here is what you currently have: CASE POUCH MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a JADE. Sorry, you can't obtain a JADE. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a CASE. You already have that item. Ninja 2, here is what you currently have: CASE POUCH MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a CASE. You already have that item. Ninja 2, here is what you currently have: CASE POUCH MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a JADE. Sorry, you can't obtain a JADE. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a JADE. You now have a JADE! Ninja 2, here is what you currently have: CASE POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a SCROLL. Sorry, you can't obtain a SCROLL. Ninja 1, here is what you currently have: Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a MASK. You already have that item. Ninja 2, here is what you currently have: CASE POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a KATANA. You now have a KATANA! Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a JADE. You already have that item. Ninja 2, here is what you currently have: CASE POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a KATANA. You already have that item. Ninja 1, here is what you currently have: KATANA Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a POUCH. You already have that item. Ninja 2, here is what you currently have: CASE POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a CASE. You now have a CASE! Ninja 1, here is what you currently have: KATANA CASE Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a SCROLL. You now have a SCROLL! Ninja 2, here is what you currently have: CASE SCROLL POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a JADE. Sorry, you can't obtain a JADE. Ninja 1, here is what you currently have: KATANA CASE Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a SCROLL. You already have that item. Ninja 2, here is what you currently have: CASE SCROLL POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 You've been caught! Which piece would you like to lose? 0. KATANA 1. CASE 0 You have selected the KATANA to discard. Ninja 1, here is what you currently have: CASE Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a MASK. You already have that item. Ninja 2, here is what you currently have: CASE SCROLL POUCH JADE MASK Ninja 1, it is your turn. Enter 0 to look for an item. 0 Ninja 1, you found a POUCH. You now have a POUCH! Ninja 1, here is what you currently have: CASE POUCH Ninja 2, it is your turn. Enter 0 to look for an item. 0 Ninja 2, you found a KATANA. You now have a KATANA! Ninja 2, here is what you currently have: KATANA CASE SCROLL POUCH JADE MASK Congratulations Ninja 2, you are the first to return with all of the stolen items!
if you can give me the correct code, I will rate you 5 stars and buy an hour or 2 of tutoring from you, thanks in advanced.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
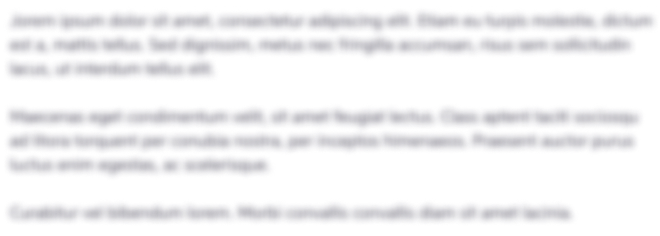
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started