Question
Assignment Question: We discussed Queue class in our lectures. In addition to what we discussed, please add the following functions to the Queue class that
Assignment Question:
We discussed Queue class in our lectures. In addition to what we discussed, please add the following functions to the Queue class that we discussed. Test it as you add each new operation. When you're done, use the program Qtester.cpp to test your class.
1. << Overload the output operator << so that a statement of the form cout << q; will display q's contents from front to back.)
2. size() //returns the number of elements in the queue
3. back() // returns the element at the back of a queue
Here is what I have so far:
"Queue.h"
#ifndef QUEUE #define QUEUE #include using namespace std; const int QUEUE_CAPACITY = 128; typedef int QueueElement; class Queue { public: Queue(); bool empty() const; void enqueue(const QueueElement & value); QueueElement front() const; void dequeue(); friend ostream & operator<<(ostream & out, const Queue & Q); int size(); QueueElement back(); private: QueueElement myArray[QUEUE_CAPACITY]; int myFront, myBack; int count; }; #endif
"Queue.cpp"
#include "Queue.h" #include using namespace std; Queue::Queue() //Purpose: Constructor //Precondition: Declared queue //Postcondition: Queue is defined as empty { myFront = 0; myBack = 0; count = 0; } bool Queue::empty() const //Purpose: Checks to see if queue empty //Precondition: Declared queue //Postcondition: Returns true if the queue is empty { return myFront == myBack; } void Queue::enqueue(const QueueElement & value) //Purpose: Adds a value to the queue //Precondition: A value to be added to the queue //Postcondition: Adds the value to the queue { if (count != QUEUE_CAPACITY) { count++; myArray[myBack] = value; myBack = (myBack + 1) % QUEUE_CAPACITY; } else cerr << "Queue is full." << endl; } QueueElement Queue::front() const //Purpose: Sets element at the top of the queue //Precondition: The queue //Postcondition: Changes the topmost element in queue to a new value { if (!empty()) return (myArray[myFront]); else cerr << "Queue is empty." << endl; return myArray[QUEUE_CAPACITY - 1]; } void Queue::dequeue() //Purpose: Removes topmost value in queue //Precondition: The queue //Postcondition: Returns the queue without its top value { if (!empty()) { count--; myFront = (myFront + 1) % QUEUE_CAPACITY; } else cerr << "Queue is empty." << endl; } ostream & operator<<(ostream &out, const Queue & Q) //Purpose: Overloaded output operator //Precondition: The queue //Postcondition: Returns the output { for (int i = Q.myFront; i != Q.myBack; i = (i + 1) % QUEUE_CAPACITY) out << Q.myArray[i] << endl; return out; } int Queue::size() //Purpose: Returns the number of elements in the queue //Precondition: The queue //Postcondition: Returns number of elements in queue { Queue q; int count = 0; while (!q.empty) { q.dequeue(); count++; } return count; } QueueElement Queue::back() //Purpose: Returns the element at the back of a queue //Precondition: The queue //Postcondition: Returns the element at the back of a queue { Queue q; QueueElement temp; while (!q.empty()) { temp = q.front(); q.dequeue(); } return temp; }
"Tester.cpp"
#include "Queue.h" #include #include using namespace std; void ShowCommands() { cout << "Use the following commands to test the Queue class:" << endl; cout << "a---add an element to the queue" << endl; cout << "d---display contents of queue" << endl; cout << "e---test whether a queue is empty" << endl; cout << "f---retrieve the item at the front of the queue" << endl; cout << "h---help--print this list of commands" << endl; cout << "r---remove item from front of the queue" << endl; cout << "s---display the size of queue" << endl; cout << "b---retrieve the item at the back of the queue" << endl; cout << "q---quit testing" << endl; } int main() { QueueElement item; char command; Queue q; ShowCommands(); do { cout << "Command? "; cin >> command; if (isupper(command)) command = tolower(command); switch (command) { case 'a': cout << "Enter item to add to queue: "; cin >> item; q.enqueue(item); cout << "--> " << item << " added "; break; case 'd': cout < "--> Queue contents: " << q << endl; break; case 'e': cout << "--> Queue " << (q.empty() ? "is" : "is not") << " empty "; break; case 'f': cout << "--> " << q.front() << " is at the front "; break; case 'h': ShowCommands(); break; case 'r': q.dequeue(); cout << "--> Front element removed "; break; case 's': cout << "--> the size of the queue is: " << q.size() << endl; break; case 'b': cout << "--> " << q.back() << " is at the back "; break; case 'q': cout << "--> End of test "; break; default: cout << "*** Illegal command: " << command << endl; } } while (command != 'q'); }
I have a few errors on the tester and am not sure why. Help is appreciated!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
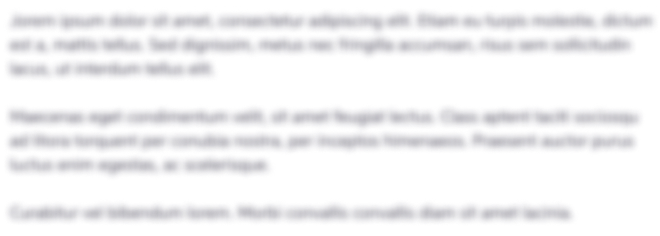
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started