Question
Attached Files: File LinkedListType.h (1.415 KB) #ifndef LinkedList_H #define LinkedList_H #include #include using namespace std; //Definition of the node struct nodeType { int info; nodeType
Attached Files: File LinkedListType.h (1.415 KB)
#ifndef LinkedList_H
#define LinkedList_H
#include #include using namespace std;
//Definition of the node struct nodeType { int info;
nodeType *link;
};
class linkedListType { public: linkedListType();
//default constructor void removeFromFront();
//Removing the node from the head of
// the linked list void removeAfterMe(nodeType * afterMePtr);
//Remove the Node after a Given Node from a Linked List void insertAtFront(int dataVal);
//Insert a Node at the Front of a Linked List void insertAfterMe(nodeType *afterMePtr, int dataVal);
//Insert a Node after a Given Node in a Linked List void printList(ostream &outs);
//Output the Data Parts of the Nodes of a Linked List(List Traversal) void destroyList();
//Destroy a Linked List void appendNode(int dataVal);
//Append a Node to a Linked List nodeType * searchValue(int target);
//Searching a Linked List for a node with target value
// returns a pointer to the first found node with the target
//Initializes the list to an empty state.
//Postcondition: first = NULL, count = 0;
~linkedListType(); //destructor
//Deletes all the nodes from the list.
//Postcondition: The list object is destroyed. protected: int count;
//variable to store the number of list elements // nodeType *head;
//pointer to the first node of the list };
#endif File main.cpp (1.136 KB)
#include #include "linkedListType.h"
using namespace std;
int main() {
linkedListType list1, list2;
int num;
bool found = false;
cout << "Enter numbers ending with -999" << endl;
cin >> num;
while (num != -999) { list1.appendNode(num);
list2.insertAtFront(num);
cin >> num;
}
cout << endl; cout << "List1: ";
list1.printList(cout);
cout << "List2: ";
list2.printList(cout);
cout << "Enter a number to be searched for in list1:" << endl;
cin >> num;
nodeType * ptr;
ptr = list1.searchValue(num);
// print the search results if (ptr) cout << "Found! ";
else cout << "Not Found! ";
cout << "Enter a number to be inserted after " << num << " in list1:" << endl;
cin >> num;
list1.insertAfterMe(ptr, 345);
cout << "Enter a number to be searched for in list2:" << endl;
cin >> num; ptr = list2.searchValue(num);
// print the search results if (ptr) cout << "Found! ";
else cout << "Not Found! ";
list2.removeAfterMe(ptr);
cout << "List1: ";
list1.printList(cout);
cout << "List2: ";
list2.printList(cout);
system("PAUSE");
return 0;
}
You need to create "main.cpp", "LinkedListType.h", and "LinkedListType.cpp" to implement LinkedListType class with all the member functions
Note: Important! Some of the functions we covered in the class have "IntegerNode * head" as its arguments. But, in the class definitions, we need to omit it from arguments since "head" is a protected class member variable.
Input:
12 45 23 24 76 23 9 124 234 87 43 -34 12 -45 123 126 29 -999
Enter a number to be searched for in list1:
76
Enter a number to be inserted after 76 in list1:
-36
Enter a number to be searched for in list2:
234
Hint: the destructor may be implemented as follows:
linkedListType::~linkedListType() {
destroyList();
}//end destructor
Step by Step Solution
There are 3 Steps involved in it
Step: 1
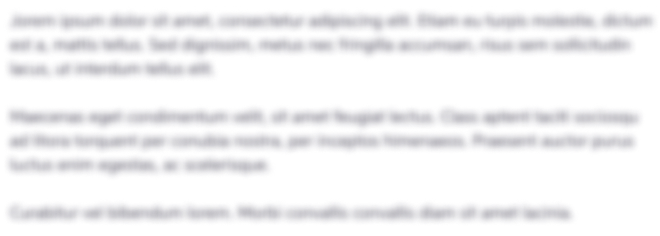
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started