Question
BANK ACCOUNT EXAMPLE #include #include #include using namespace std; class Account { private : int accountNumber; double balance; public : Account( int accountNumber, double balance
BANK ACCOUNT EXAMPLE
#include
#include
#include
using namespace std;
class Account {
private:
int accountNumber;
double balance;
public:
Account(int accountNumber, double balance = 0.0);
int getAccountNumber() const;
double getBalance() const;
void setBalance(double balance);
void credit(double amount);
void debit(double amount);
void print() const;
};
Account::Account(int no, double b) : accountNumber(no), balance(b) { }
int Account::getAccountNumber() const {
return accountNumber;
}
double Account::getBalance() const {
return balance;
}
void Account::setBalance(double b) {
balance = b;
}
void Account::credit(double amount) {
balance += amount;
}
void Account::debit(double amount) {
if (amount <= balance) {
balance -= amount;
}
else {
cout << "Amount withdrawn exceeds the current balance!" << endl;
}
}
void Account::print() const {
cout << fixed << setprecision(2);
cout << "A/C no: " << accountNumber << " Balance=$" << balance << endl;
}
int main() {
Account a1(8111, 99.99);
a1.print();
a1.credit(20);
a1.debit(10);
a1.print();
Account a2(8222);
a2.print();
a2.setBalance(100);
a2.credit(20);
a2.debit(200);
a2.print();
return 0;
}
USING C++ for a MAC
Using the bankAccount example that we studied in class, extend the solution we coded and consider the following points: a) Redefine the class bankAccount to store a bank customers account number, owners fullname, and balance. Your class should, besides many possible others, provide the followingoperations: retrieve the account number, retrieve the owners full name, retrieve the balance,deposit and withdraw money, and print account information. b) Banks offer checking accounts. Derive the class checkingAccount from the class bankAccount (designed in part (a)). A customer with a checking account does not receive interest but pays service charges if the balance falls below the minimum balance ($100). Add member variables to store this additional information. In addition to the operations inherited from the base class, this class should provide the following operations: set minimum balance, retrieve minimum balance, set service charges, retrieve service charges, verify if the balance is less than the minimum balance, etc. c) Banks offer savings accounts. Derive the class savingsAccount from the class bankAccount (designed in part (a)). A customer with a saving account typically receives interest. In addition to the operations inherited from the base class, this class should provide the following operations: set interest rate, retrieve interest rate, post interest, etc. |
d) Write a program to test your classes. Bonus points will be given if you do not hardcode inputs in your program (prompt the user for options). |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
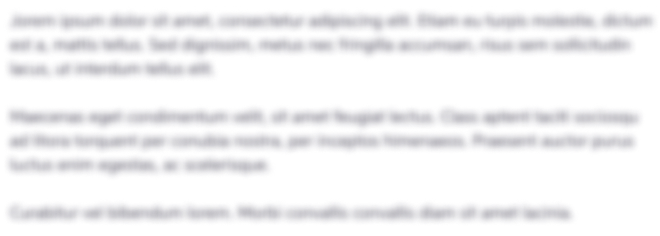
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started