Question
Bank Accounting System C++ PROGRAMING Develop a bank account transaction processing system. This software is the basis for the software that would be used by
Bank Accounting System C++ PROGRAMING
Develop a bank account transaction processing system. This software is the basis for the software that would be used by a bank to manage user accounts. When developing the interface, you should imagine the interactions between a bank teller and a customer.
There are two main programs in this assignment. 1) teller_simulation, and 2) ReportGeneration.
Teller Simulation
The first program simulates a bank teller that customers would interact with to create accounts, deposit, or withdraw funds. The following dialog is one possible set of interactions that your software system must provide interfaces for:
Teller: Hello, Let's make a new account for you. CustomerRecord: My name is "Wade Wilson", I live at "201 14th Street, Seattle Washington, 91312", and I my social security number is "SSN_9999" Teller: Thank you! Now that you are a member of our bank, can we open an account? For you? CustomerRecord: Yes please. Please create both a checking account, and a saving account associated with my SSN. Teller: You two accounts are created with zero balance. Would you like to make a deposit? CustomerRecord: Please makeDeposit of $57 in my checking account. CustomerRecord: Please makeDeposit $77 in my savings account. Teller: Would you like to make a withdrawal? CustomerRecord: Yes, makeWithdrawal of $100 from my savings account. Teller: Sorry, you have insufficient funds to perform that task, your acount was not modified.
At the beginging of each day when the bank accounting program starts, all previous customer transactions are read in from a file on disk.
At the end of the day when the program ends, the entire transaction history needs to be written to disk.
Report Generation
The second program reads a transaction history file (provided to the program as argv[1]), and generates a report of each customers account balances.
GENERAL RULES:
All users need a name with more than 5 characters in their name.
CustomerSSN's must be unique (no two customers can have the same SSN)
Only 1 bank object can be used.
ZipCode must have exactly 5 digits.
State must be exactly 2 characters (bonus, validate only valid states).
All public interface itmes must be justified. Many private attributes will not have public getters/setters.
You are allowed to use std::cerr only for error processing.
Address - Has Street - Has City - Has State - Has ZipCode Bank + Must be able to add a customer (and validate rules) + Must be able to change addresses + Must be able to verify that a customer exists (HasCustomer based on SSN). - Has many Customers - Has RoutingBankID (Can never be changed after bank is created) (RountingBankIDK always begins with the letter 'R') - Has Bank Name (Can never be changed after bank is created) - Has Address HINT: Some attributes will NOT have public geters/setters; HINT: maps need : object of type 'Bank' cannot be assigned because its copy assignment operator is implicitly deleted mapOfAllBanks[routingNumber] = myBank; ^ /Users/johnsonhj/cie/ENGR2730Private/homework/homework4/exampleSolution/Bank.h:41:21: note: copy assignment operator of 'Bank' is implicitly deleted because field 'm_RoutingBankID' has no copy assignment operator const std::string m_RoutingBankID; ^ CustomerRecord + Must be able to create a new account ledger for Checking/Savings/ or Loan acocunts. Error checking must be available to check if an account already exists. - Has Address - Has CustomerRecord Name - Has Accounts (You may assume that a customer has at most 1 each type of account, but you may *not* assume that a customer has all accounts) - Has CustomerSSN (Can never be changed after customer is created) CustomerSSN (Always begins with letter 'S') AccountLedger + Must be able to make deposits (verified that only non-negative values are requested to be deposited). Invalid request result in error message but no transaction recorded. Deposits must optionally be annotated with a memo Timestamps for new transactions need to be timestamped with "chrono" + Must be able to make withdrawls (verified that non-negative values accepted to be withdrawn), Must verify that sufficient funds are available to make the withdrawal. Invalid request result in error message but no transaction recorded. Internal to the class, the value stored will be negated to indicate a reduction in the balance. Withdrawals must optionally be annotated with a memo + Must be able to compute the balance of the account as needed. The balance MUST NOT BE STORED, it must be computed from the list of transactions. - AccountLedger Type [Checking|Savings|Loan] - List of transactions Transaction - Change value - Date/Time (use time_t which is an integer representing # of seconds past a given epoch time)
HINTS:
m_AccountMapping[type] = AccountLedger(type); This requires 3 functions to complete: m_AccountMapping[type] --> Needs AccountLedger() = --> needs operator=(....) AccountLedger(type) --> Needs AccountLedger( AccountType )
Use typedefs judiciously in this assignment.
NameType --> std::string, or ?? SSNType --> std::string or char *, or class? StreetNameType --> std::string, or ?? ZipCodeType --> (int, or string, or float, or something else)
Having a record like the following is not acceptable:
"","",""","","","""
any help and/or suggestions on how to do aspects of this would be greatly appreciated, I'm not looking for the full code, I am having alot of trouble with the class structures and interface, MUST BE IN C++.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
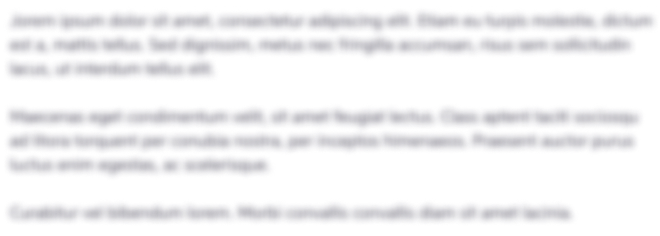
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started