Question
Based on these files, need assistance with the pokedex.cpp file. It will use input and output file from the pokedex.txt file given and string summary
Based on these files, need assistance with the pokedex.cpp file. It will use input and output file from the pokedex.txt file given and string summary from pokemon.cpp. It also uses an array constructor. only use these libraries: #include#include #include #include #include and very basic syntax,statements, I'm still learning c++. pokedex.h file: Not to be changed #ifndef POKEDEX_H #define POKEDEX_H #include #include "pokemon.h" using namespace std; class Pokedex { public: // A file containing a Pokedex should contain a line // for each Pokemon in the Pokedex. This line // should be the summary string of the Pokemon. // See pokemon.txt for an example. // Constructs an empty pokedex. Pokedex(); // Constructs a Pokedex with the Pokemon found in // the specified file. Pokedex(string filename); // Writes the Pokedex to the file. void save(string filename); // Adds a pokemon to the pokedex. void add(Pokemon* p); // Removes a pokemon from the pokedex. void remove(Pokemon* p); // Returns a (pointer to a) pokemon in the pokedex with the given name. // If none exists, returns nullptr. // // Hint: loop through all of A, searching for a Pokemon with // the given name. Return the first one found. Pokemon* lookup_by_name(string name); // Returns a (pointer to a) pokemon in the pokedex with the given name. // If none exists, returns nullptr. // // Hint: look in A[ndex]. Pokemon* lookup_by_Ndex(int ndex); // Returns the number of pokemon in the pokedex. int size(); private: // The pokedex is represented as an array of Pokemon pointers. // // Hint: // 1. Initialize the values in A to nullptr. // 2. When adding a Pokemon('s pointer), store the pointer in // the array index equal to the Pokemon's Ndex. // 3. When removing a Pokemon('s pointer), do so by setting the // corresponding array index equal to nullptr. Pokemon* A[1000]; }; #endif
pokemon.cpp file: Not to be changed
#include "pokemon2.h"
#include "pokedex.h"
#include
#include
using namespace std;
// A summary string is a single string that contains all of a Pokemon's information.
// A Pokemon with one type and Ndex 1 has a summary string of the form:
// "Name, #001, type1,"
// Similarly, a Pokemon with two types and Ndex 2 has a summary string of the form:
// "Name, #002, type1, type2,"
// Initializes a Pokemon from a summary string
Pokemon::Pokemon(string summary)
{
string name;
int index[3];
string type1;
string type2;
int count = 0;
int comma = 0;
while (summary[count] != '\0')
{
if (summary[count] == ',')
{
++comma;
if (comma == 1)
{
for (int i = 0; i < count; ++i)
{
name += summary[i];
}
index[0] = summary[count + 3] - 48;
index[1] = summary[count + 4] - 48;
index[2] = summary[count + 5] - 48;
}
if (comma == 2)
{
for (int i = count + 2; summary[i] != ','; ++i)
{
type1 += summary[i];
}
}
if (comma == 3 && summary[count + 1] == ' ')
{
for (int i = count + 2; summary[i] != ','; ++i)
{
type2 += summary[i];
}
}
}
++count;
}
if (comma == 3)
{
this->type_count = 1;
}
else
{
this->type_count = 2;
}
this->types[0] = string_to_type(type1);
this->types[1] = string_to_type(type2);
this->_name = name;
this->_ndex = index[0] * 100 + index[1] * 10 + index[2];
}
string Pokemon::summary()
{
string summary;
string separate = ", ";
string num = "#";
string name = this->_name;
string index;
string type1;
string type2;
type1 = type_to_string(this->types[0]);
if (is_multitype())
{
type2 = type_to_string(this->types[1]);
type2 += ",";
}
type1 += ",";
if (this->_ndex < 100)
{
index = "0" + to_string(this->_ndex);
if (this->_ndex < 10)
{
index = "00" + to_string(this->_ndex);
}
}
else
{
index = to_string(this->_ndex);
}
summary = name + separate + num + index + separate + type1;
if (is_multitype())
{
summary += " " + type2;
}
return summary;
}
// Same as hwPKM1
Pokemon::Pokemon(string name, int ndex, Type type1)
{
this->_name = name;
this->_ndex = ndex;
this->types[0] = type1;
this->types[1] = type1;
this->type_count = 1;
}
Pokemon::Pokemon(string name, int ndex, Type type1, Type type2)
{
this->_name = name;
this->_ndex = ndex;
this->types[0] = type1;
this->types[1] = type2;
this->type_count = 2;
}
string Pokemon::name()
{
return _name;
}
int Pokemon::Ndex()
{
return _ndex;
}
Pokemon::Type Pokemon :: type1()
{
return types[0];
}
bool Pokemon::is_multitype()
{
if (type_count == 1)
{
return false;
}
return true;
}
Pokemon::Type Pokemon :: type2()
{
return types[1];
}
float Pokemon::damage_multiplier(Type attack_type)
{
float array[4][4] = { { 1.0,1.0,1.0,1.0 },{ 2.0,1.0,0.5,0.5 },{ 1.0,2.0,1.0,1.0 },{ 1.0,1.0,1.0,0.5 } };
float multiplier = array[attack_type][types[0]];
if (is_multitype() == true)
{
multiplier = multiplier * array[attack_type][types[1]];
}
return multiplier;
}
// Returns a string corresponding to the type. Examples:
// 1. type_to_string(Pokemon::Poison) returns "Poison".
// 2. type_to_string(Pokemon::Normal) returns "Normal".
string type_to_string(Pokemon::Type t)
{
if (t == Pokemon::Normal)
{
return "Normal";
}
if (t == Pokemon::Fighting)
{
return "Fighting";
}
if (t == Pokemon::Flying)
{
return "flying";
}
if (t == Pokemon::Poison)
{
return "Poison";
}
}
// Returns the type corresponding to a string. Examples:
// 1. string_to_type("Poison") returns Pokemon::Poison.
// 2. string_to_type("Normal") returns Pokemon::Normal.
// 3. Allowed to return anything if given string doesn't
// correspond to a Pokemon type.
Pokemon::Type string_to_type(string s)
{
if (s == "Normal")
{
return Pokemon::Normal;
}
if (s == "Fighting")
{
return Pokemon::Fighting;
}
if (s == "Flying")
{
return Pokemon::Flying;
}
if (s == "Poison")
{
return Pokemon::Poison;
}
}
pokedex.txt file: not to be changed just used for input/output file and read/write to implement pokedex.cpp
Aipom, #190, Normal, Ambipom, #424, Normal, Arbok, #024, Poison, Arceus, #493, Normal, Audino, #531, Normal, Bidoof, #399, Normal, Blissey, #242, Normal, Bouffalant, #626, Normal, Braviary, #628, Normal, Flying, Buneary, #427, Normal, Bunnelby, #659, Normal, Castform, #351, Normal, Chansey, #113, Normal, Chatot, #441, Normal, Flying, Cinccino, #573, Normal, Conkeldurr, #534, Fighting, Croagunk, #453, Poison, Fighting, Crobat, #169, Poison, Flying, Delcatty, #301, Normal, Ditto, #132, Normal, Dodrio, #085, Normal, Flying, Doduo, #084, Normal, Flying, Dunsparce, #206, Normal, Eevee, #133, Normal, Ekans, #023, Poison, Exploud, #295, Normal, Farfetch'd, #083, Normal, Flying, Fearow, #022, Normal, Flying, Fletchling, #661, Normal, Flying, Furfrou, #676, Normal, Furret, #162, Normal, Garbodor, #569, Poison, Glameow, #431, Normal, Golbat, #042, Poison, Flying, Grimer, #088, Poison, Gulpin, #316, Poison, Gurdurr, #533, Fighting, Happiny, #440, Normal, Hariyama, #297, Fighting, Hawlucha, #701, Fighting, Flying, Herdier, #507, Normal, Hitmonchan, #107, Fighting, Hitmonlee, #106, Fighting, Hitmontop, #237, Fighting, Hoothoot, #163, Flying, Normal, Kangaskhan, #115, Normal, Kecleon, #352, Normal, Koffing, #109, Poison, Lickilicky, #463, Normal, Lickitung, #108, Normal, Lillipup, #506, Normal, Linoone, #264, Normal, Lopunny, #428, Normal, Loudred, #294, Normal, Machamp, #068, Fighting, Machoke, #067, Fighting, Machop, #066, Fighting, Makuhita, #296, Fighting, Mankey, #056, Fighting, Meowth, #052, Normal, Mienfoo, #619, Fighting, Mienshao, #620, Fighting, Miltank, #241, Normal, Minccino, #572, Normal, Muk, #089, Poison, Munchlax, #446, Normal, Nidoran, #029, Poison, Nidoran, #032, Poison, Nidorina, #030, Poison, Nidorino, #033, Poison, Noctowl, #164, Normal, Flying, Pancham, #674, Fighting, Patrat, #504, Normal, Persian, #053, Normal, Pidgeot, #018, Normal, Flying, Pidgeotto, #017, Normal, Flying, Pidgey, #016, Normal, Flying, Pidove, #519, Normal, Flying, Porygon, #137, Normal, Porygon-Z, #474, Normal, Porygon2, #233, Normal, Primeape, #057, Fighting, Purugly, #432, Normal, Raticate, #020, Normal, Rattata, #019, Normal, Regigigas, #486, Normal, Riolu, #447, Fighting, Rufflet, #627, Normal, Flying, Sawk, #539, Fighting, Sentret, #161, Normal, Seviper, #336, Poison, Skitty, #300, Normal, Slaking, #289, Normal, Slakoth, #287, Normal, Smeargle, #235, Normal, Snorlax, #143, Normal, Spearow, #021, Normal, Flying, Spinda, #327, Normal, Stantler, #234, Normal, Staraptor, #398, Normal, Flying, Staravia, #397, Normal, Flying, Starly, #396, Normal, Flying, Stoutland, #508, Normal, Swablu, #333, Normal, Flying, Swalot, #317, Poison, Swellow, #277, Normal, Flying, Taillow, #276, Normal, Flying, Tauros, #128, Normal, Teddiursa, #216, Normal, Throh, #538, Fighting, Timburr, #532, Fighting, Tornadus, #641, Flying, Toxicroak, #454, Poison, Fighting, Tranquill, #520, Normal, Flying, Trubbish, #568, Poison, Tyrogue, #236, Fighting, Unfezant, #521, Normal, Flying, Ursaring, #217, Normal, Vigoroth, #288, Normal, Watchog, #505, Normal, Weezing, #110, Poison, Whismur, #293, Normal, Zangoose, #335, Normal, Zigzagoon, #263, Normal, Zubat, #041, Poison, Flying,
Step by Step Solution
There are 3 Steps involved in it
Step: 1
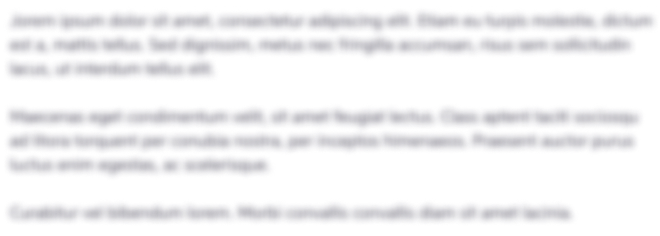
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started