Question
Before starting with part 1, we will introduce two functions These two member functions are available in istream: setstate(); peek(); setstate() manually sets the status
Before starting with part 1, we will introduce two functions
These two member functions are available in istream:
setstate(); peek();
- setstate() manually sets the status of the istream object to the desired state. In our case we will be calling setstate as follows to manually set any object of istream (like cin) to a fail state:
// Examples: // assuming there is a funciton called readData as follows istream& readData(istream& istr){ type variable{}; istr >> variable; if(/*logic for variable being invalid*/){ istr.setstate(ios::failbit); // this manually sets istr to failure // if the variable is readable but not acceptable } return istr; } // Or: int getAge(){ int age{}; cin >> age; if(age < 19){ cin.setstate(ios::failbit); // this sets cin to fail if the age is bellow 19 } return age; } void someLogic(){ cout << "Enter your name: "; int age = getAge(); if(cin.fail()){ cout << "You are not old enough to consume alcoholic drinks in Ontario!" < // Example char next; int value; cout <<"Enter a number: "; next = cin.peek(); if(next < '0' || next >'9'){ // checking if the next character in keyboard is not a digit cout << "You did not enter a number!"; } else{ cin >> value; } cin.ignore(1000, ' '); // flush the invalid value or everything after the number. Part 1 - lab (50%), The HealthCard class Your task for this part of your workshop is to complete the implementation of a class called HeathCard. This class encapsulates some basic health-card information of a person in Ontario using the following attributes: class HealthCard { char* m_name{}; long long m_number; char m_vCode[3]; char m_sNumber[10]; public: ... }; Validation These values are validated (considered valid) as follows: The HeathCard class is to validate and store the above information via initialization and data entry from istream. Also, the HeathCard class must comply with the rule of three. (i.e. the implementation of copy constructor, copy assignment and destructor) Although the name of the HealthCard is dynamically held, we can assume that the maximum length for a name is 55 characters. This value should be kept in a constant global variable so it can be changed at compile-time if needed. Finally, A HeathCard object should reveal its status (of being valid or invalid) via a Boolean type conversion overload (a true outcome means the object is valid and a false outcome means it is invalid). To accomplish the above and have an organized and modular code, implement these private methods to help you with the implementation of the whole logic: (you can add more if you like to) Private Methodsbool validID(const char* name, long long number, const char vCode[] , const char sNumber[]) const; Returns true is the four parts of the ID card are valid. (see Validation) void setEmpty(); Sets the HeathCard object to a recognizable empty (invalid) state by setting m_name to nullptr; void allocateAndCopy(const char* name); void HealthCard::extractChar(istream& istr, char ch) const; "peek()" and see if the next character in the keyboard buffer is the same as the ch argument ostream& printIDInfo(ostream& ostr)const; Inserts the three parts related to the main card number, version code and stock number of the health card information into the istr object in the following format: 1234567890-AB, XY7652341 and then returns the istr object reference void set(const char* name, long long number, const char vCode[], const char sNumber[]); Validates the arguments, reallocates memory for the name and sets the object attributes to their corresponding values. Constructors The HeathCard can either get created with no values (default constructor) into a safe empty state or use all four values. Instead of overloading the constructor you can use one constructor with the default values for the four parameters, (i.e nullptr, 0, {}, {} ) and remember to reuse the set function for the latter case. Note that since the m_name attribute is initialized in the class definition to be nullptr, there is no need to worry about setting it to nullptr before calling the set function. Rule of threeCopy Constructor HeathCard(const HeathCard& hc); Copy Assignment operator overload HeathCard& operator=(const HeathCard& hc); Destructor deletes the memory pointed by m_name. Boolean type conversion operator Returns true if m_name is not nullptr, else it will return false ostream& print(ostream& ostr, bool toFile = true) const; If the current object is in a valid state it inserts the values of the card information in two different formats based on the value of the toFile argument: istream& read(istream& istr); Reads the Contact information in following format: Example: Luke Skywalker,1231231234-XL,AF1234567 implementation insertion operator overload ostream& operator<<(ostream& ostr, const Contact& hc); if hc is valid it will print it using the print function on the screen and not on File, (i.e. onFile is false). Otherwise, it will print "Invalid Card Number". In the end, it will return the ostr reference. extraction operator overload istream& operator>>(istream& istr, Contact& hc) returns the read method of the hc argument. The tester program Tester output Files to Submit HealthCard.cpp HealthCard.h HealthCardInfo.csv main.cpp
const int MaxNameLength = 55;
// Workshop #6: // Version: 1.0 // File name: main.cpp // Date: 2021/12/02 // Author: Wail Mardini // Description: // This file tests the lab section of your workshop /////////////////////////////////////////////////// #include
Validation Test Fred Soley........................................1234567890-AB, WQ1234567 Invalid Health Card Record Invalid Health Card Record Invalid Health Card Record Fred Soley........................................1234567890-AB, WQ1234567 Invalid Health Card Record Invalid Health Card Record Invalid Health Card Record Data entry test. Enter the test data using copy and paste to save time: Enter the following: >Person Name,1231231234-XL,AF1234567 >Person Name,1231231234-XL,AF1234567 HealthCard Content: Person Name.......................................1231231234-XL, AF1234567 Enter the following: >Person Name,1231231234-XL,AF123456 >Person Name,1231231234-XL,AF123456 HealthCard Content: Invalid Health Card Record Enter the following: >Person Name,1231231234-L,AF1234567 >Person Name,1231231234-L,AF1234567 HealthCard Content: Invalid Health Card Record Enter the following: >Person Name,1231231234-,AF1234567 >Person Name,1231231234-,AF1234567 HealthCard Content: Invalid Health Card Record Enter the following: >Person Name,131231234-XL,AF1234567 >Person Name,131231234-XL,AF1234567 HealthCard Content: Invalid Health Card Record Enter the following: >Person Name 1231231234-XL,AF1234567 >Person Name 1231231234-XL,AF1234567 HealthCard Content: Invalid Health Card Record Invalid Health Card Record 2Person Name2.....................................1231231234-XL, AF1234567 Invalid Health Card Record 4Person Name3.....................................1231231234-XL, AF1234567 Invalid Health Card Record Invalid Health Card Record Invalid Health Card Record 8Person Name7.....................................1231231234-XL, AF1234567 Invalid Health Card Record Invalid Health Card Record 11Person Name10...................................1231231234-XL, AF1234567 12Person Name11...................................1231231234-XL, AF1234567 13Person Name12...................................1231231234-XL, AF1234567 14Person Name13...................................1231231234-XL, AF1234567 15Person Name.....................................1231231234-XL, AF1234567 Invalid Health Card Record 17Person Name.....................................1231231234-XL, AF1234567 18Person Name.....................................1231231234-XL, AF1234567 Invalid Health Card Record 20P...............................................1231231234-XL, AF1234567 21Person Name.....................................1231231234-XL, AF1234567 22Person Name.....................................1231231234-XL, AF1234567 Invalid Health Card Record 24Person Name.....................................1231231234-XL, AF1234567 25Person Name.....................................1231231234-XL, AF1234567 All records were read successfully! Contents of goodInfo.csv ---------------------------------------------------------------- 2Person Name2,1231231234-XL, AF1234567 4Person Name3,1231231234-XL, AF1234567 8Person Name7,1231231234-XL, AF1234567 11Person Name10,1231231234-XL, AF1234567 12Person Name11,1231231234-XL, AF1234567 13Person Name12,1231231234-XL, AF1234567 14Person Name13,1231231234-XL, AF1234567 15Person Name,1231231234-XL, AF1234567 17Person Name,1231231234-XL, AF1234567 18Person Name,1231231234-XL, AF1234567 20P,1231231234-XL, AF1234567 21Person Name,1231231234-XL, AF1234567 22Person Name,1231231234-XL, AF1234567 24Person Name,1231231234-XL, AF1234567 25Person Name,1231231234-XL, AF1234567
HealthCard.h HealthCard.cpp main.cpp HealthCardInfo.csv
#define _CRT_SECURE_NO_WARNINGS #include
#ifndef SDDS_HEALTHCARD_H #define SDDS_HEALTHCARD_H namespace sdds { const int MaxNameLength = 55; class HealthCard { char* m_name{}; long long m_number; char m_vCode[3]; char m_sNumber[10]; public: }; } #endif // !SDDS_HealthCard_H
1Person Name1Person Name1Person Name1Person Name1Person Name1Person Name1Person Name1,1231231234-XL,AF1234567 2Person Name2, 1231231234-XL,AF1234567 , 1231231234-XL,AF1234567 4Person Name3,1231231234-XL,AF1234567 5Person Name4,1231231234-,AF1234567 6Person Name5,123123234-XL,AF1234567 7Person Name6,-XL,AF134567 8Person Name7,1231231234-XL,AF1234567 9Person Name8,1231231234-,AF1234567 10Person Name9,1231231234-XL,AF123456 11Person Name10,1231231234-XL,AF1234567 12Person Name11,1231231234-XL,AF1234567 13Person Name12,1231231234-XL,AF1234567 14Person Name13,1231231234-XL,AF1234567 15Person Name,1231231234-XL,AF1234567 16Person Name,1231231234-XL,AF234567 17Person Name,1231231234-XL,AF1234567 18Person Name, 1231231234-XL,AF1234567 19Person Name,123121234-XL,AF1234567 20P,1231231234-XL,AF1234567 21Person Name,1231231234-XL,AF1234567 22Person Name,1231231234-XL,AF1234567 23Person Name,1231231234-L,AF1234567 24Person Name,1231231234-XL,AF1234567 25Person Name,1231231234-XL,AF1234567
// Workshop #6: // Version: 1.0 // File name: main.cpp // Date: 2021/12/02 // Author: Wail Mardini // Description: // This file tests the lab section of your workshop /////////////////////////////////////////////////// #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
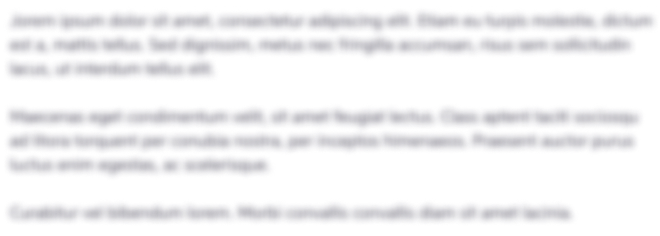
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started