Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Before we start coding up our game of blackjack, let's first code up our playing cards. In this task you need to develop the following:
Before we start coding up our game of blackjack, let's first code up our playing cards. In this task you need to develop the following:
A Suit enum, for representing clubs, spades, diamonds and hearts.
A Card class, for storing a single playing card like the ace of spades
A Deck class, for storing a complete deck of cards.
We have provided a Runner class with a main method that will be executed when you click the Run button. You are welcome to change this method however you like. This file is not used in testing and is provided purely to allow you to interact with and explore your own code.
Background
As you almost certainly already know, a deck of playing cards consists of cards, evenly split between four suits clubs spades, diamonds and hearts There are cards within each suit: the numeric cards through and the face cards Jack, Queen, King and Ace. These cards are ranked with Ace being the lowest, followed by through and then the Jack, Queen and King.
Specification
The Suit enum must provide:
The values CLUBS, SPADES, DIAMONDS and HEARTS
A static getChar method which takes a Suit, and returns either or as a char depending on the given suit.
The Card class must provide:
A constructor that accepts two parameters: the suit that the card belongs to as a Suit and an int value representing the rank of the card within the suit. You can also assume that the rank will be a number between corresponding to the ace and corresponding to the king
A getSuit method, which takes no parameters and returns the suit of the card as a Suit
A getRank method, which takes no parameters and returns the rank of the card as an int
A toString method, which takes no parameters and returns a String representing both the rank and suit of the card. For numeric cards, this string should simply contain both the rank and suit without any other punctuation eg For face cards, the rank should be represented as a single capital letter eg A J Q K
The Deck class must provide:
A constructor that accepts no parameters, and populates the deck with all playing cards no jokers The playing cards should initially be in a sequence where the suits are in the order and within each suit the cards should be ordered from ace through to king.
A getSize method that takes no parameters and returns the number of cards remaining in the deck. When the deck is first constructed, this should equal As cards are removed from the deck this number should decrease.
A deal method that takes no parameters and returns the first card from the deck, removing it from the deck and reducing the size of the deck by one. For a new unshuffled deck, the first call to this method should return the ace of clubs, followed by the two of clubs, and so on
A shuffle method that takes no parameters and returns no values. After calling this method the order of cards in the deck should be randomised, such that it is not possible to predict which card will be returned when calling deal. After calling this method the deck should still contain the same cards and thus the same total number of cards but they should be in a different order.
All of methods described above should be public and unless otherwise stated not static. You should not define any additional public methods or properties other than those explicitly stated above. You are welcome to add any private properties and methods that you feel are necessaryhelpful
Examples
Here are some examples of the output of the runner if you leave it unchanged, and implement the classes correctly:
Bobby was dealt the following cards: J
Billy was dealt the following cards: J A
Sally was dealt the following cards: A
After dealing, there are cards remaining in the deck
The output of your program may differ due to the randomness of shuffling the deck.
Hints
Expand
Feel free to use the following array to help convert from a Suit to an appropriate character ie to print it out :
char SUITS ;
Expand
Feel free to use the following array to help convert from an int rank to an appropriate character ie to print it out :
char RANKS AJQK ;
Expand
Feel free to use any of the collection classes within your Deck class, if that makes sense to you.
Expand
For the Deck.shuffle method, you will find it very useful to import either java.util.Arrays or java.util.Collections. Both of these utility classes provide a shuffle method that takes either an array or a collection respectively and randomises the order of items within it
Step by Step Solution
There are 3 Steps involved in it
Step: 1
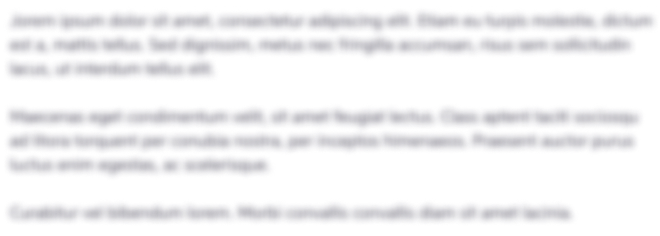
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started