Question
Below I have provided the code I have created for a super class, 2 subclasses and a Tester: public class Animal { protected int legs;
Below I have provided the code I have created for a super class, 2 subclasses and a Tester:
public class Animal { protected int legs; protected int eyes; protected String eat; protected String sound; public Animal() { int legs = 0; int eyes = 0; String eat = "nothing"; String sound = "nothing"; }//END constructor 1 with no parameters public Animal(int legs, int eyes, String eat, String sound) { legs = legs; eyes = eyes; eat = eat; sound = sound; }//END constructor 2 with (4) parameters public final void setLegs(int legs) { legs = legs; }//END setLegs(int legs) public final int getLegs() { return legs; } public final void setEyes(int eyes) { eyes = eyes; }//END setEyes(int eyes) public final int getEyes() { return eyes; }//END getEyes() public final void setEat(String eat) { eat = eat; }//END setEat(String eat) public final String getEat() { return eat; }//END getEat() public final void setSound(String sound) { sound = sound; }//END setSound(String sound) public final String getSound() { return sound; }//END getSound() //creating method printAnimal() public void printAnimal() { System.out.printf("%nFrom Animal: This animal has %d legs, %d eyes, eats nothing, sounds nothing%n", getLegs(), getEyes(), getEat(), getSound()); }//END method printAnimal() }//END class Animal
----------------------------- public class Spider extends Animal { public Spider() { super(); //invokes the parent constructor of parent class Animal }//END constructor 1 //creating constructor 2 with the (4) parameters public Spider(int legs, int eyes, String eat, String sound) { super(legs, eyes, eat, sound); //invokes the parent constructor of parent class Animal }//END constructor 2 //creating method printAnimal() @Override public void printAnimal() { System.out.printf("%nFrom Spider: legs 0, eyes 0, eats nothing, says nothing", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); System.out.printf("%nFrom Spider: legs 8, eyes 8, eats insects, sounds very quiet", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); //overrides the method with the same name in parent class Animal System.out.printf("%nFrom Spider: legs 7, eyes 0, eats nothing, sounds nothing", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); }//END method printAnimal() //creating method setSpider() with (1) incoming parameter public void setSpiderLegs(int legs) { System.out.printf("%nFrom Spider: legs 8, eyes 8, eats insects, sounds very quiet", getLegs(), getEyes(), getEat(), getSound()); super.setLegs(legs); // calls the parent class Animal method "setLegs" }//END method setSpiderLegs(int legs) }//END class Spider -------------------------- public class Lion extends Animal { public Lion(int legs, int eyes, String eat, String sound) { super(legs, eyes, eat, sound); //invokes parent constructor of parent class Animal }//END constructor Lion //creating method printAnimal() @Override public void printAnimal() { System.out.printf("%nFrom Lion: This animal has legs 4, eyes 1, eats meat, sounds 'roar' ", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); //invokes the method printAnimal() from parent class Animal System.out.printf("%nFrom Lion: This animal has legs 2, eyes 1, eats meat, sounds 'roar' ", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); System.out.printf("%nFrom Lion: This animal has legs 4, eyes 2, eats meat, sounds 'roar' ", getLegs(), getEyes(), getEat(), getSound()); super.printAnimal(); }//END method printAnimal() //creating method setLionLegs() public void setLionLegs(int legs) { System.out.printf("%nFrom Lion: This animal has legs 4, eyes 2, eats meat, sounds 'roar' ", getLegs(), getEyes(), getEat(), getSound()); super.setLegs(legs); //calls the parent class Animal "setLegs" }//END method setLionLegs(int legs) }//END class Lion ------------------------ public class AnimalTester { public static void main(String[] args) { //instantiating 2 Spider objects & 1 Lion object Spider spidy1 = new Spider(); Spider spidy2 = new Spider(8, 8, "insects", "very quiet"); Lion lion = new Lion(2, 1, "meat", "roar"); //creating the sequence of statements per instructions System.out.printf("%nGoing to print out info about spidy1%n"); spidy1.printAnimal(); System.out.printf("%nGoing to print out info about spidy2%n"); spidy2.printAnimal(); System.out.printf("%nspidy1 has 0 eyes!" + spidy1.getEyes()); System.out.printf("%nBut spidy2 has 8 eyes!%n" + spidy2.getEyes()); spidy1.setLegs(7); //sets eyes to 7 System.out.printf("%nGoing to print out info about spidy1%n"); spidy1.printAnimal(); System.out.printf("%nGoing to print out info about lion%n"); lion.printAnimal(); lion.setLegs(4); //sets eyes to 4
System.out.printf("%nGoing to print out info about lion%n"); lion.printAnimal(); lion.setEyes(2);//sets eyes to 2 System.out.printf("%nGoing to print out info about lion%n"); lion.printAnimal(); System.out.printf("%nEnd of program"); }//END main method }//END class AnimalTester ------------------------------------
Below is the output I keep getting: How do I fix it to get the correct output?
Going to print out info about spidy1
From Spider: legs 0, eyes 0, eats nothing, says nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 8, eyes 8, eats insects, sounds very quiet From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 7, eyes 0, eats nothing, sounds nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
Going to print out info about spidy2
From Spider: legs 0, eyes 0, eats nothing, says nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 8, eyes 8, eats insects, sounds very quiet From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 7, eyes 0, eats nothing, sounds nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
spidy1 has 0 eyes!0 But spidy2 has 8 eyes! 0 Going to print out info about spidy1
From Spider: legs 0, eyes 0, eats nothing, says nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 8, eyes 8, eats insects, sounds very quiet From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Spider: legs 7, eyes 0, eats nothing, sounds nothing From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
Going to print out info about lion
From Lion: This animal has legs 4, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 2, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 4, eyes 2, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
Going to print out info about lion
From Lion: This animal has legs 4, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 2, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 4, eyes 2, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
Going to print out info about lion
From Lion: This animal has legs 4, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 2, eyes 1, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
From Lion: This animal has legs 4, eyes 2, eats meat, sounds 'roar' From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds nothing
Below is the CORRECT output needed:
Going to print out info about spidy1
From Spider: legs 0, eyes 0, eat nothing, says "nothing"
From Animal: This animal has 0 legs, 0 eyes, eats nothing, sounds "nothing"
Going to print out info about spidy2
From Spider: legs 8, eyes 8, eat insects, says "very quiet"
From Animal: This animal has 8 legs, 8 eyes, eats nothing, sounds "very quiet"
spidy1 has 0 eyes!
But spidy2 has 8 eyes!
Going to print out info about spidy1
From Spider: legs 7, eyes 0, eat nothing, says "nothing"
From Animal: This animal has 7 legs, 0 eyes, eats nothing, sounds "nothing"
Going to print out info about lion
From Lion: legs 2, eyes 1, eat meat, says "roar"
From Animal: This animal has 2 legs, 1 eyes, eats meat, sounds "roar"
Going to print out info about lion
From Lion: legs 4, eyes 1, eat meat, says "roar"
From Animal: This animal has 4 legs, 1 eyes, eats meat, sounds "roar"
Going to print out info about lion
From Lion: legs 4, eyes 2, eat meat, says "roar"
From Animal: This animal has 4 legs, 2 eyes, eats meat, sounds "roar"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
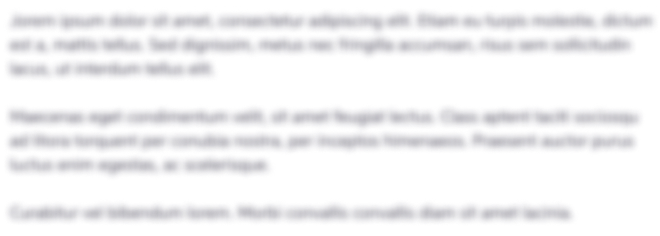
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started