Question
Below is my code, and this MyProgram is my tester for the game. I am having these errors which i listed below the MyProgram class,
Below is my code, and this MyProgram is my tester for the game. I am having these errors which i listed below the MyProgram class, please fix. Thanks.
import java.io.IOException;
import java.util.Scanner;
public class MyProgram
{
public static void main(String[] args)
{
Scanner kb = new Scanner(System.in);
// Creates the board.
private Board board = new Board();
// Array of players.
private Player[] players = new Player[2];
// Creates a turn.
private Turn turn;
int[] count = new int[2];
final static int BLACK = 1;
final static int WHITE = 0;
public static void main(String[] args) throws IOException
{
new MyProgram().startGame();
}
/**
* Starts the game by finding first players possible moves
*/
public void startGame() throws IOException
{
int who = this.initPlayers();
this.turn = new Turn((who + 1) % 2);
// Asks players for their names.
for (int i = 0; i < 2; i++)
{
System.out.print("Player " + (i + 1) + " ");
players[i].setNames();
}
// Indicates player's turn.
System.out.println(players[0].getName() + " moves");
this.players[turn.getTurn()].findCanSelect();
board.display(); // Displays board.
// Finds possible moves when game is not over.
while (!board.gameOver())
{
// Count of possible moves.
int count = 0;
// Searches the entire board.
for (int row = 0; row < Board.num; row++)
{
for (int col = 0; col < Board.num; col++)
{
//Finds valid moves.
if (board.findLegalMove(new Move(row, col), turn.getTurn()) == true)
{
count++; // Adds a possible move to the count.
}
}
}
// When no possible moves are available.
if (count == 0)
{
turn.change(); // Switches to other players turn.
board.display(); // Prints the updated board.
board.scoreDisplay(); //Prints updated score.
count = 0; // Reset all the possible moves to 0.
}
else
{
int row = this.getRow();
int col = this.getCol();
// Creates a new move.
Move move = new Move(row, col);
// If a valid move.
if (board.canSelect(move))
{
// Place and replace chip.
this.players[turn.getTurn()].placeChip(row, col);
turn.change(); // Then changes the turn to the other player.
}
// Finds the possible moves.
this.players[turn.getTurn()].findCanSelect();
board.display(); // Displays updated board with possible moves.
// Indicates whose turn it is.
System.out.println(players[turn.getTurn()].getName() + " moves");
}
}
System.out.println("Game over!");
}
/**
* Creates two players.
*/
private int initPlayers()
{
Turn a = new Turn(); // Temporary turn.
this.players[0] = new Player("name 1", a.getTurn(), this.board); // Player 1
a.change(); // Changes to player 2.
this.players[1] = new Player("name 2", a.getTurn(), this.board); // Player 2
return 1;
}
/**
* Gets the row for move.
*/
private int getRow()
{
System.out.print("Select a row: ");
Integer value = -1;
value = kb.nextInt();
return value; // Returns the value selected.
}
/**
* Gets the col for move.
*/
private int getCol()
{
System.out.print("Select a column: ");
Integer value = -1;
value = kb.nextInt();
return value; // Returns value selected.
}
}
}
Errors:
-
MyProgram.java: Line 11: illegal start of expression
-
MyProgram.java: Line 14: illegal start of expression
-
MyProgram.java: Line 17: illegal start of expression
-
MyProgram.java: Line 22: illegal start of expression
-
MyProgram.java: Line 22: illegal start of expression
-
MyProgram.java: Line 22: You forgot a
;
, probably at the end -
MyProgram.java: Line 22: You forgot a
.class
, probably at the end -
MyProgram.java: Line 22: You forgot a
;
, probably at the end -
MyProgram.java: Line 22: illegal start of expression
-
MyProgram.java: Line 22: You forgot a
;
, probably at the end -
MyProgram.java: Line 30: illegal start of expression
-
MyProgram.java: Line 30: illegal start of expression
-
MyProgram.java: Line 30: You forgot a
;
, probably at the end -
MyProgram.java: Line 30: Not a statement.
-
MyProgram.java: Line 30: You forgot a
;
, probably at the end -
MyProgram.java: Line 105: illegal start of expression
-
MyProgram.java: Line 105: You forgot a
;
, probably at the end -
MyProgram.java: Line 118: illegal start of expression
-
MyProgram.java: Line 118: You forgot a
;
, probably at the end -
MyProgram.java: Line 129: illegal start of expression
-
MyProgram.java: Line 129: You forgot a
;
, probably at the end
---------------------------------------------------------------------------------------------------------
public class Cell
{
// Displays 'B' for the black disk player.
public static final char BLACK = 'B';
// Displays 'W' for the white disk player.
public static final char WHITE = 'W';
// Displays '*' for the possible moves available.
public static final char CANSELECT = '*';
// If the cell is empty or not.
public boolean empty;
// If the cell can be selected or not.
public boolean canselect;
// empty = -1 , white = 0 , black = 1
public int value;
/**
* Class constructor. Cell is empty by default.
*/
public Cell()
{
this.empty = true;
this.value = -1;
}
/**
* Checks if cell is empty or not.
*/
public boolean isEmpty()
{
return this.empty;
}
/**
* Returns player's number.
*/
public int getPlayer()
{
return this.value;
}
/**
* Places a chip in the cell.
*/
public void placeChip(int player)
{
this.empty = false;
this.value = player;
}
/**
* Changes the colour value of chip (0,1).
*/
public void changeChip()
{
placeChip((value + 1) % 2);
}
/**
* Makes cell possible to select/choose.
*/
public void setSelect()
{
this.canselect = true;
}
/**
* Makes the cell selectable.
*/
public boolean canSelect()
{
return this.canselect;
}
/**
* Makes the cell not selectable.
*/
public void unselect()
{
this.canselect = false;
}
/**
* Displays the cell so that valid moves are *, black is B, and white is W.
*/
public void display()
{
// If the cell is empty.
if (this.isEmpty())
{
// If the cell can be selected.
if (this.canselect)
{
System.out.print("[ " + CANSELECT + " ]"); // Print "*."
}
// Prints a empty space.
else
{
System.out.print("[ " + " " + " ]");
}
}
else
{
char content = BLACK;
if (this.value == 0)
{
content = WHITE;
System.out.print("[ " + content + " ]"); // For black "B" & for white "W."
}
}
}
}
------------------------------------------------------------------------------------------------------------------------------------------------------------
import java.util.ArrayList;
public class Board
{
// 8 rows by 8 columns board.
public static final int num = 8;
// Matrix
private Cell[][] cell = new Cell[num][num];
// Counter for both players moves.
int[] counter = new int[2];
// Game over.
boolean gameOver;
final static int BLACK = 1;
final static int WHITE = 0;
public final Move up = new Move(0, -1); // Up
public final Move down = new Move(0, 1); // Down
public final Move left = new Move(-1, 0); // Left
public final Move right = new Move(1, 0); // Right
public final Move upLeft = new Move(-1, -1); // Up and left
public final Move upRight = new Move(1, -1); // Up and right
public final Move downLeft = new Move(-1, 1); // Down and left
public final Move downRight = new Move(1, 1); // Down and right
// Array of possible directions.
final Move directions[] = { up, down, left, right, upLeft, upRight, downLeft, downRight };
public Board()
{
// Goes through the whole board.
for (int row = 0; row < num; row++)
{
// Sets all the cells to be empty.
for (int col = 0; col < num; col++)
{
this.cell[row][col] = new Cell();
}
}
// 1 for black.
this.cell[3][4].placeChip(1);
this.cell[4][3].placeChip(1);
// 0 for white .
this.cell[3][3].placeChip(0);
this.cell[4][4].placeChip(0);
// Start counter at 2 for each player.
counter[0] = 2; // White player
counter[1] = 2; // Black player
// Allows moves at start of game.
gameOver = false;
}
/**
* Gets the cell clicked.
* Row selected.
* Col selected.
*/
public Cell getCell(int row, int col)
{
return cell[row][col];
}
/**
* Gets the row of the selected cell.
*/
public int getNumRows()
{
return cell.length;
}
/**
* Gets the col of the selected cell.
*/
public int getNumCols()
{
return cell[0].length;
}
/**
* Displays the board with row and col numbers.
*/
public void display()
{
for (int i = 0; i < num; i++)
{
System.out.print(i + " ");
for (int j = 0; j < num; j++)
{
this.cell[i][j].display();
}
System.out.println(" ");
}
System.out.print(" ");
for (int j = 0; j < num; j++)
{
System.out.print(" " + j + " ");
}
System.out.println();
}
/**
* Displays the winner of the game.
*/
public void winner()
{
if (getChipsCount(0)
{
System.out.println("Black Won!");
}
else if(getChipsCount(1)
{
System.out.println("White Won!");
}
}
/**
* Displays the scores of both players.
*/
public void scoreDisplay()
{
System.out.println("White score: " + getChipsCount(0));
System.out.println("Black score: " + getChipsCount(1));
System.out.println();
}
/**
* Finds all the valid and available moves of current player.
*/
public boolean findLegalMove(Move move, int player)
{
// No legal moves found by default.
boolean result = false;
// Finds the opponent.
int opponent = (player + 1) % 2;
// Player playing.
int cPlayer = player;
int i = move.getI(); // Gets the position of i axis.
int j = move.getJ(); // Gets the position of j axis.
// Checks if the cell is empty.
if (cell[i][j].isEmpty() == false)
{
return false; // If the cell is not empty, no moves will be available.
}
else
{
// Gets all the directions.
for (int k = 0; k < directions.length; k++)
{
Move direction = directions[k];
int iDir = direction.getI(); //Gets i and j axis.
int jDir = direction.getJ();
int jump = 2; // Jumps one chip.
try {
if (cell[i + iDir][j + jDir].getPlayer() == opponent)
{
while ((j + (jump * jDir)) > -1 && (j + (jump * jDir)) < 8 && (i + (jump * iDir)) < 8 && (i + (jump * iDir)) > -1)
{
// If cell cannot be empty.
if (!cell[i + jump * iDir][j + jump * jDir].isEmpty())
{
if (cell[i + jump * iDir][j + jump * jDir].getPlayer() == cPlayer)
{
return true;
}
else if (cell[i + jump * iDir][j + jump * jDir].isEmpty())
{
break;
}
}
else
{
break;
}
jump++; // Jumps another extra chip.
}
}
}
catch (Exception e)
{
}
}
}
// If true, move found. If false, no moves found.
return result;
}
/**
* Places the chip on the board
*/
public void placeChip(int colour, int row, int col)
{
this.cell[row][col].placeChip(colour);
}
/**
* An array list of all valid moves available.
*/
public ArrayList validMoves(int colour)
{
ArrayList allValidMoves = new ArrayList();
for (int i = 0; i < cell.length; i++)
{
for (int j = 0; j < cell.length; j++)
{
cell[i][j].unselect();
Move testMove = new Move(i, j);
boolean valid = findLegalMove(testMove, colour);
if (valid)
{
allValidMoves.add(testMove);
}
}
}
return allValidMoves;
}
/**
* Allows the cell to be selected.
*/
public void setCanSelect(Move move)
{
this.cell[move.getI()][move.getJ()].setSelect();
}
/**
* Allows the cell to be selected
*/
public boolean canSelect(Move move)
{
return this.cell[move.getI()][move.getJ()].canSelect();
}
/**
* Replaces the chip(s) in between the players old and current chip.
*/
public void replaceChip(Move move, int player)
{
int opponent = (player + 1) % 2;
int playing = player;
int i = move.getI();
int j = move.getJ();
for (int movement = 0; movement < directions.length; movement++)
{
Move direction = directions[movement];
int iDir = direction.getI();
int jDir = direction.getJ();
boolean possible = false;
// Checks for the opponent in the all directions.
if ((j + jDir) > -1 && (j + jDir) < num && (i + iDir) < num && (i + iDir) > -1)
{
if (cell[i + iDir][j + jDir].getPlayer() == opponent)
{
possible = true;
}
}
if (possible == true)
{
int jump = 2;
while ((j + (jump * jDir)) > -1 && (j + (jump * jDir)) < 8 && (i + (jump * iDir)) < 8 && (i + (jump * iDir)) > -1)
{
// Thecell cannot be emptied.
if (!cell[i + jump * iDir][j + jump * jDir].isEmpty())
{
if (cell[i + jump * iDir][j + jump * jDir].getPlayer() == playing)
{
for (int k = 1; k < jump; k++)
{
cell[i + k * iDir][j + k * jDir].changeChip();
}
break;
}
jump++;
}
}
}
}
}
/**
* Updates the number/counter/disks of chips on the board per player.
*/
public int getChipsCount(int colour)
{
int score = 0;
for (int i = 0; i < num; i++)
{
for (int j = 0; j < num; j++)
{
if (cell[i][j].getPlayer() == colour)
{
score++;
}
}
}
return score;
}
/**
* Checks if all the 64 chips have been placed on board which then means the game is over.
*/
public boolean gameOver()
{
int count = 0;
// If cells are full, game over.
if (counter[0] + counter[1] == 64)
{
return true;
}
else
{
for (int j = 0; j < num; j++)
{
for (int i = 0; i < num; i++)
{
if (findLegalMove(new Move(i, j), 0) == true)
{
count++;
}
if (findLegalMove(new Move(i, j), 1) == true)
{
count++;
}
}
}
// If there is no legal moves left then the game will be over.
if (count == 0)
{
return true;
}
}
return false;
}
}
----------------------------------------------------------------------------------------------------------------------------------------------------
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
public class Player
{
Scanner kb = new Scanner(System.in);
// Player's name.
private String name;
// Colour of the chips.
private int colour;
// Board
private Board board;
/**
* Creates a player.
*/
public Player(String name, int colour, Board board)
{
this.name = name;
this.colour = colour;
this.board = board;
}
/**
* Places the piece for the chosen player at the chosen location. Replaces the piece in between
* the old piece and the new piece to the players colour.
*/
public void placeChip(int row, int col)
{
this.board.placeChip(this.colour, row, col);
Move move = new Move(row, col);
this.board.replaceChip(move, this.colour);
}
/**
* Sets the players name to a variable.
*/
public void setNames() throws IOException
{
System.out.println("What is your name: ");
String line = kb.nextLine();
this.name = line;
}
/**
* Gets the name of the player.
*/
public String getName()
{
return this.name;
}
/**
* Gets the colour number of the player.
*/
public int getcolour()
{
return this.colour;
}
/**
* Finds valid moves, and allows them to be selected.
*/
public void findCanSelect()
{
ArrayList moves = board.validMoves(this.colour);
for (Move move : moves)
{
board.setCanSelect(move);
}
}
}
-------------------------------------------------------------------------------------------------------------------------------------
public class Turn
{
// The players number.
private int value;
/**
* Creates a turn starting with a random player.
*/
public Turn()
{
this.value = (int) (Math.random() * 2.0d);
}
/**
* Creates a turn starting with the selected first player.
*/
public Turn(int v)
{
this.value = v;
}
/**
* Returns the player whose turn it is.
*/
public int getTurn()
{
return this.value;
}
/**
* Changes the turn to the other player.
*/
public void change()
{
this.value = (this.value + 1) % 2;
}
}
-------------------------------------------------------------------------------------------------------
class Move
{
// Position on i and j axis.
int i;
int j;
/**
* Creates the directions on the i and j axis.
*/
public Move(int i, int j)
{
this.i = i;
this.j = j;
}
/**
* Gets the location of the position on the i axis.
*/
public int getI()
{
return i;
}
/**
* Gets the location of the position on j axis.
*/
public int getJ()
{
return j;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
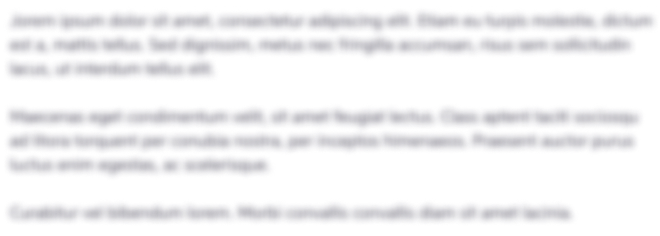
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started