Question
Below is the BASE implementation of the MIPSzy's processor design in Verilog code. Extend the base implementation to support MIPS instructions including sub, j, slt,
Below is the BASE implementation of the MIPSzy's processor design in Verilog code. Extend the base implementation to support MIPS instructions including sub, j, slt, beq, jal, jr. I started the CTRL, (mipszy_ctrl.v), by adding sub, jal, beq, and slt
Help, MUCH appreciated?!
Verilog for 32-bit adder. (adder_32.v) ________________________ `timescale 1ns / 1ps
module adder_32(A, B, S); input [31:0] A, B; output reg [31:0] S;
always @(A, B) begin S = A + B; end endmodule
Verilog for 32-bit incrementer that increments by 4. (inc4_32.v) ________________________ `timescale 1ns / 1ps
module inc4_32(A, S); input [31:0] A; output reg [31:0] S;
always @(A) begin S = A + 4; end endmodule
Verilog for 1024x32 memory used for instruction and data memories. (mem_1024x32.v) ________________________ `timescale 1ns / 1ps module mem_1024x32(clk, ra, rd, re, wa, wd, we); input clk; input [9:0] ra, wa; input re, we; output reg [31:0] rd; input [31:0] wd;
reg [31:0] memory [0:1023];
always @(posedge clk) begin if (we) begin memory[wa] = wd; end end
always @* begin if (re) begin rd = memory[ra]; end else begin rd = 0; end end endmodule
Verilog for MIPSzy's control logic component CTRL. (mipszy_ctrl.v) ________________________ `timescale 1ns / 1ps
module mipszy_ctrl(ir31_26, ir5_0, rf_wd_s, rf_wa_s, rf_we, rf_r1e, rf_r2e, add2_s, dm_we, dm_re);
input [5:0] ir31_26, ir5_0; output reg rf_wd_s, rf_wa_s, rf_we, rf_r1e, rf_r2e; output reg add2_s; output reg dm_we, dm_re;
always @* begin if (ir31_26 == 'b100011) begin // lw rf_wd_s = 0; rf_wa_s = 1; rf_we = 1; rf_r1e = 1; rf_r2e = 0; add2_s = 0; dm_we = 0; dm_re = 1; end else if (ir31_26 == 'b101011) begin // sw rf_wd_s = 0; rf_wa_s = 0; rf_we = 0; rf_r1e = 1; rf_r2e = 1; add2_s = 0; dm_we = 1; dm_re = 0; end else if (ir31_26 == 'b001000) begin // addi rf_wd_s = 1; rf_wa_s = 1; rf_we = 1; rf_r1e = 1; rf_r2e = 0; add2_s = 0; dm_we = 0; dm_re = 0; end else if (ir31_26 == 'b000000 && ir5_0 == 'b100000) begin // add rf_wd_s = 1; rf_wa_s = 0; rf_we = 1; rf_r1e = 1; rf_r2e = 1; add2_s = 1; dm_we = 0; dm_re = 0; end else if ( (ir31_26 == 0b000000) && (ir5_0 == 0b100010) ) begin // sub rf_r1e = 1; rf_r2e = 1; add_add2_s = 1; rf_wd_s = 1; rf_wa_s = 0; rf_we = 1; add_sub = 1; end else if (ir31_2e6ls=e= i0fb0(0i0r0311_)2{6 == 0b000011) begin // jal rf_wd_s1s0 = 0b10; rf_wa_s1s0 = 0b10; we = 1; pc_s = 1; end else if (ir31_26 == 0b000100) begin // beq rf_r1e = 1; rf_r2e = 1; add_add2_s = 1; if (alu_eq == 1) begin pc_s = 1; // Branch (pass target address) end else begin pc_s = 0; // No branch (just pass PC + 4) end end else if ((ir32_26 == 0b000000) && (ir5_0 == 0b101010)) begin // slt rf_r1e = 1; rf_r2e = 1; add_add2_s = 1; alu_less = 1; rf_wd_s = 1; rf_wa_s = 0; rf_we = 1; end //still missing j, and jr else begin rf_wd_s = 0; rf_wa_s = 0; rf_we = 0; rf_r1e = 0; rf_r2e = 0; add2_s = 0; dm_we = 0; dm_re = 0; end end endmodule
Verilog for MIPSzy's top-level structure. (MIPSzy.v) ________________________ `timescale 1ns / 1ps
module MIPSzy(clk, rst); input clk, rst;
// Ctrl wires wire rf_wd_s, rf_wa_s, rf_we, rf_r1e, rf_r2e; wire add2_s; wire dm_we, dm_re;
// PC wires wire [31:0] pc_out, pc_inc4;
// IM/IR wires wire [31:0] ir; wire [5:0] ir31_26, ir5_0; wire [4:0] ir20_16, ir15_11, ir25_21; wire [15:0] ir15_0;
// SW wires wire [31:0] ir15_0_se;
// RF wires wire [4:0] rf_wa; wire [31:0] rf_wd; wire [31:0] rf_r1d, rf_r2d;
// Adder wires wire [31:0] add2, add1, add_sum;
// DM wires wire [31:0] dm_a; wire [31:0] dm_wd; wire [31:0] dm_rd;
// internal connections assign dm_a = rf_r1d; assign dm_wd = rf_r2d; assign add1 = rf_r1d;
assign ir31_26 = ir[31:26]; assign ir5_0 = ir[5:0]; assign ir20_16 = ir[20:16]; assign ir15_11 = ir[15:11]; assign ir25_21 = ir[25:21]; assign ir15_0 = ir[15:0];
// component instantiations mipszy_ctrl CTRL(ir31_26, ir5_0, rf_wd_s, rf_wa_s, rf_we, rf_r1e, rf_r2e, add2_s, dm_we, dm_re); register_32 PC(clk, rst, 1'b1, pc_inc4, pc_out); inc4_32 PC_inc(pc_out, pc_inc4); mux2x1_32 RF_wd_mux(add_sum, dm_rd, rf_wd_s, rf_wd); mux2x1_32 RF_wa_mux(ir20_16, ir15_11, rf_wa_s, rf_wa); regfile_32x32 RF(clk, rst, ir25_21, rf_r1d, rf_r1e, ir20_16, rf_r2d, rf_r2e, rf_wa, rf_wd, rf_we); signext_16_32 SE(ir15_0, ir15_0_se); mux2x1_32 ADD_mux(rf_r2d, ir15_0_se, add2_s, add2); adder_32 ADD(add1, add2, add_sum); mem_1024x32 IM(clk, pc_out[11:2], ir, 1'b1, 1'b0, 1'b0, 1'b0); mem_1024x32 DM(clk, dm_a[11:2], dm_rd, dm_re, dm_a[11:2], dm_wd, dm_we); endmodule
Verilog for 32-bit 2x1 mux. (mux2x1_32.v) ________________________ `timescale 1ns / 1ps module mux2x1_32(I1, I0, s, D); input [31:0] I1, I0; input s; output reg [31:0] D;
always @(I1, I0, s) begin if (!s) begin D = I0; end else begin D = I1; end end endmodule
Verilog for 32x32 register file with 2 read ports and 1 write port. (regfile_32x32.v) ________________________ `timescale 1ns / 1ps
module regfile_32x32(clk, rst, r1a, r1d, r1e, r2a, r2d, r2e, wa, wd, we); input clk, rst; input [4:0] r1a, r2a, wa; input r1e, r2e, we; output reg [31:0] r1d, r2d; input [31:0] wd;
integer i; reg [31:0] registers [0:31];
// Write procedure always @(posedge clk) begin if (rst) begin for (i = 0; i < 32; i = i+1) begin registers[i] = 0; end end else if (we) begin registers[wa] = wd; end end
// Read ports procedure always @* begin // Read port 1 if (r1e) begin r1d = registers[r1a]; end else begin r1d = 0; end
// Read port 2 if (r2e) begin r2d = registers[r2a]; end else begin r2d = 0; end end endmodule
Verilog for 32-bit register used for PC. (register_32.v?)
________________________ `timescale 1ns / 1ps
module register_32(clk, rst, ld, D, Q); input clk, rst; input ld; input [31:0] D; output reg [31:0] Q;
always @(posedge clk) begin if (rst) begin Q = 0; end else if (ld) begin Q = D; end end endmodule
Verilog for 16-bit to 32-bit sign extension. (signext_16_32.v) ________________________ `timescale 1ns / 1ps
module signext_16_32(I, O); input signed [15:0] I; output reg [31:0] O;
always @(I) begin O = I; end endmodule
Step by Step Solution
There are 3 Steps involved in it
Step: 1
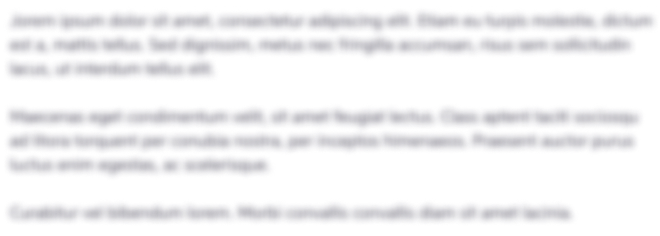
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started