Question
C++ A rational number is of the form a/b, where a and b are integers with b 0. Develop and test a class for processing
C++ A rational number is of the form a/b, where a and b are integers with b 0. Develop and test a class for processing rational numbers. The class should have a numerator data member and a denominator data member. It should read and display all rational numbers in the format a/b; for output, just display a if the denominator is 1. The following examples illustrate the operations that should be provided.
Operator | Example | Result |
Addition | 3/9 + 1/7 | 10/21 |
Subtraction | 3/9*1/7 | 4/21 |
Multiplication | 3/8 * 1/6 | 1/16 |
Division | 3/8 / 1/6 | 9/4 |
Invert | 2/4 I | 4/2 |
Mixed fraction | 8/3 M | 2 and 2/3 |
Reduce | 18/24 R | 3/4 |
Less than | 1/6 < 3/8 | True |
Less than or equal to | 1/6 <= 3/8 | True |
Greater Than | 1/6 > 3/8 | False |
Greater than or equal | 1/6 >= 3/8 | False |
Equal to | 3/8 == 9/24 | True |
Program Plan: Rnumber.h Create the class Rnumber Declare the required member function as public Declare the required member variables as private Declare operator overloading function for various operations Rnumber.cpp Definition of Reduce() function in which it checks if the numerator and denominator value is less than zero Definition of Mixed number() with two parameter gcd() function in which it stores the numerator by denominator value. Function definition for lcd() function definition for operator overloading (+=) function definition for operator overloading (+) function definition for operator overloading (-=) function definition for operator overloading
function definition for operator overloading (/=)(-)
function definition for operator overloading (/)
function definition for operator overloading (==) function definition for operator overloading (!=) function definition for operator overloading (<=) function definition for operator overloading (>) function definition for operator overloading (>=) function definition for operator overloading (<) operator overloading for '<<' operator overloading for '>>'
Rnumber.cpp Definition of Reduce() function in which it checks if the numerator and denominator value is less than zero Definition of Mixed number() with two parameter gcd() function in which it stores the numerator by denominator value. Function definition for lcd() function definition for operator overloading (+=) function definition for operator overloading (+) function definition for operator overloading (-=) function definition for operator overloading (-)
function definition for operator overloading (/=) function definition for operator overloading (/)
function definition for operator overloading (==) function definition for operator overloading (!=) function definition for operator overloading (<=) function definition for operator overloading (>) function definition for operator overloading (>=) function definition for operator overloading (<) operator overloading for '<<' operator overloading for '>>' Main.cpp Declaration of main help() function is declared The for loop gets executed unconditionally until if the user enter quit Switch case operation is performed for the various condition If the switch case operation is not satisfied then default operation are performed.
- The arithmetic operators must be overloaded to work with the class
- The class must have
- At least 2 private member variables, numerator and denominator
- At least 4 public member functions
- getNu(), getDe(), setNu(value), setDe(value)
You must have internal documentation within your code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
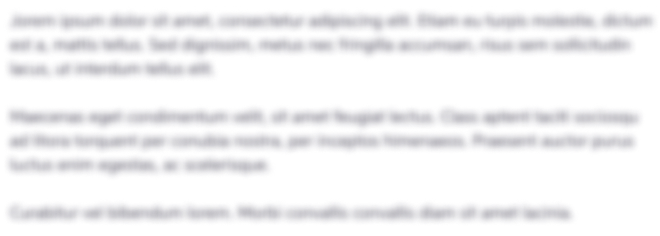
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started