Question
C++ a. Write removeLast(n). Delete the last occurrence of an item from a linked list. So if the item is 7 and the list is
C++
a. Write removeLast(n). Delete the last occurrence of an item from a linked list. So if the item is 7 and the list is [1,3,7,4,7,3,7,2], the result is [1,3,7,4,7,3,2]
b. Use linkList2.cpp Write removeAll(int n). Deletes all occurrences of an item n from a linked list. So if the item is 7 and the list1 is [1,3,7,4,7,3,2] , then list1.removeAll(7) then list1 becomes [1,3,4,3,2].
Demonstrate by displaying the list contents before and after calling the above methods. Eg:
lst1
[1,3,7,4,7,3,7,2]
lst1.removelast(7)
[1,3,7,4,7,3,2]
lst1.removeall(7)
[1,3,4,3,2]
Demonstrate: To your main create lst1 with the same values shown above.
call lst1.removelast(7)
display the contents of the list
call lst1.removeall(7)
display the contents of the list
//adds methods to search and to remove an item with a specified key value //linkList2.cpp //demonstrates linked list #include
using namespace std; //////////////////////////////////////////////////////////////// class Link { public: int iData; //data item (key) Link * pNext; //next link in list //------------------------------------------------------------- Link(int id): //constructor iData(id), pNext(NULL) {} //------------------------------------------------------------- void displayLink() //display ourself: {22, 2.99} { cout << "{" << iData << "} "; } }; //end class Link //////////////////////////////////////////////////////////////// class LinkList { private: Link * pFirst; //ptr to first link on list public: //------------------------------------------------------------- LinkList(): pFirst(NULL) //constructor {} //(no links on list yet) //------------------------------------------------------------- ~LinkList() //destructor (deletes links) { Link * pCurrent = pFirst; //start at beginning of list while (pCurrent != NULL) //until end of list, { Link * pOldCur = pCurrent; //save current link pCurrent = pCurrent -> pNext; //move to next link delete pOldCur; //delete old current } } //------------------------------------------------------------- void insertFirst(int id) { //make new link Link * pNewLink = new Link(id); pNewLink -> pNext = pFirst; //it points to old first link pFirst = pNewLink; //now first points to this } //------------------------------------------------------------- Link * find(int key) //find link with given key { //(assumes non-empty list)
Link * pCurrent = pFirst; //start at first'
while (pCurrent -> iData != key) //while no match, {
if (pCurrent -> pNext == NULL) //if end of list, return NULL; //didn't find it else //not end of list, pCurrent = pCurrent -> pNext; //go to next link } return pCurrent; //found it
} //------------------------------------------------------------- bool remove(int key) //remove link with given key { //(assumes non-empty list)
Link * pCurrent = pFirst; //search for link
Link * pPrevious = pFirst;
while (pCurrent -> iData != key) {
if (pCurrent -> pNext == NULL) return false; //didn't find it else {
pPrevious = pCurrent; //go to next link
pCurrent = pCurrent -> pNext;
} } //found it if (pCurrent == pFirst) //if first link, pFirst = pFirst -> pNext; //change first else //otherwise, pPrevious -> pNext = pCurrent -> pNext; //bypass it delete pCurrent; //delete link
return true; //successful removal
} //------------------------------------------------------------- void displayList() //display the list {
cout << "List (first-->last): ";
Link * pCurrent = pFirst; //start at beginning of list
while (pCurrent != NULL) //until end of list, {
pCurrent -> displayLink(); //print data
pCurrent = pCurrent -> pNext; //move to next link
} cout << endl;
} void removeLast(int n) { } void removeAll(int n) { } //------------------------------------------------------------- }; //end class LinkList //////////////////////////////////////////////////////////////// int main() { LinkList lst1; //make list lst1.insertFirst(2); lst1.insertFirst(7); lst1.insertFirst(3); lst1.insertFirst(7); lst1.insertFirst(4); lst1.insertFirst(7); lst1.insertFirst(3); lst1.insertFirst(1);
lst1.removeLast(7); lst1.displayList(); lst1.removeAll(7); lst1.displayList(); return 0; } //end main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
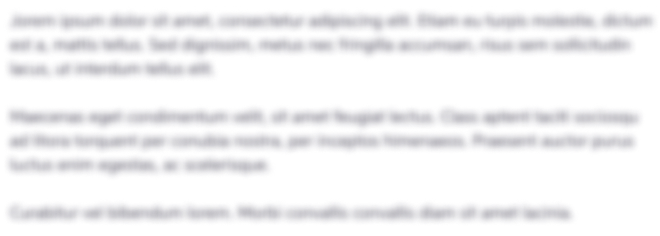
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started