Question
C# Coding; DOING SOMETHING WRONG> Instructions Danielle, Edward, and Francis are three salespeople at Holiday Homes. Write an application named HomeSales that prompts the user
C# Coding;
DOING SOMETHING WRONG>
Instructions
Danielle, Edward, and Francis are three salespeople at Holiday Homes. Write an application named HomeSales that prompts the user for a salesperson initial (D, E, or F) input as a string. Either uppercase or lowercase initials are valid. While the user does not type Z, continue by prompting for the amount of a sale. Issue the error message "Sorry - invalid salesperson" for any invalid initials entered. Keep a running total of the amounts sold by each salesperson. After the user types Z or z for an initial, display each salespersons total, a grand total for all sales, and the name of the salesperson with the highest total unless there is a tie. If there is a tie, indicate this in the program's output with the message: "There was a tie".
An example of the program is shown below:
Enter a salesperson initial >> D Enter amount of sale >> 10 Enter next salesperson intital or Z to quit >> d Enter amount of sale >> 2 Enter next salesperson intital or Z to quit >> E Enter amount of sale >> 15 Enter next salesperson intital or Z to quit >> f Enter amount of sale >> 9 Enter next salesperson intital or Z to quit >> Z Danielle sold $12.00 Edward sold $15.00 Francis sold $9.00 Total sales were $36.00 Edward sold the most
In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US")));
my code:
using System;
using static System.Console;
using System.Globalization;
class HomeSales
{
static void Main()
{
// Write your main here.
char ch = 'D';
int sum = 0, sumD = 0, sumE = 0, sumF = 0, value;
while (true)
{
Console.WriteLine("Enter the" + " intitial for salesperson" + " D, E, F and Z to terminate");
ch = Convert.ToChar(Console.ReadLine());
if (ch == 'd' || ch == 'D')
{
Console.WriteLine("Please" + " enter the sale amount");
value = Convert.ToInt32(Console.ReadLine());
sum = sum + value;
sumD = sumD + value;
}
else if (ch == 'e' || ch == 'E')
{
Console.WriteLine("Please" + "enter the sale amount");
value = Convert.ToInt32(Console.ReadLine());
sum = sum + value;
sumE = sumE + value;
}
else if (ch == 'f' || ch == 'F')
{
Console.WriteLine("Please" + " enter the sale amount");
value = Convert.ToInt32(Console.ReadLine());
sum = sum + value;
sumF = sumF + value;
}
else if (ch == 'z' || ch == 'Z')
{
break;
}
else
{
Console.WriteLine("Sorry - invalid salesperson");
}
}
if (sum > 0)
{
Console.WriteLine("Danielle sold {0}", sumD.ToString("C", CultureInfo.GetCultureInfo("en-US")));
Console.WriteLine("Edward sold {0}", sumE.ToString("C", CultureInfo.GetCultureInfo("en-US")));
Console.WriteLine("Francise sold {0}", sumF.ToString("C", CultureInfo.GetCultureInfo("en-US")));
Console.WriteLine("Total sales were {0}", sum.ToString("C", CultureInfo.GetCultureInfo("en-US")));
if (sumD > sumE && sumD > sumF)
{
Console.WriteLine("Danielle sold the most");
}
else if (sumE > sumD && sumE > sumF)
{
Console.WriteLine("Edward sold the most");
}
else if (sumF > sumD && sumF > sumE)
{
Console.WriteLine("Francis sold the most");
}
else
{
Console.WriteLine("There was a tie");
}
}
}
}
MindTap says I am doing this wrong.
Test CaseIncomplete
Program accepts both upper and lower case value for a salesperson's initial
Input
D 10 d 5 Z
Output
Enter the intitial for salesperson D, E, F and Z to terminate Please enter the sale amount Enter the intitial for salesperson D, E, F and Z to terminate Please enter the sale amount Enter the intitial for salesperson D, E, F and Z to terminate Danielle sold $15.00 Edward sold $0.00 Francise sold $0.00 Total sales were $15.00 Danielle sold the most
Results
Danielle\ssold\s*\$15\.00
Edward\ssold\s*\$0\.00
Francis\ssold\s*\$0\.00
Expected Output
Danielle\ssold\s*\$15\.00
Edward\ssold\s*\$0\.00
Francis\ssold\s*\$0\.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
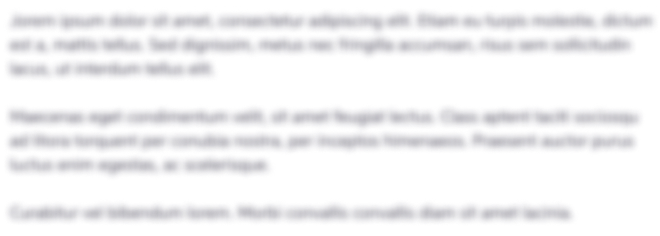
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started