Question
C++ coding! File account.hh: #ifndef ACCOUNT_HH #define ACCOUNT_HH #include class Account { public: // Constructor Account(const std::string& owner, bool has_credit = false); // More methods
C++ coding! File account.hh: #ifndef ACCOUNT_HH #define ACCOUNT_HH #includeclass Account { public: // Constructor Account(const std::string& owner, bool has_credit = false); // More methods // Method to set the credit limit void set_credit_limit(double limit); // Method to save money void save_money(double amount); // Method to take money bool take_money(double amount); // Method to transfer money bool transfer_to(Account& receiver, double amount); // Method to print account information void print(); private: // Generates IBAN (based on running_number_ below). // Allows no more than 99 accounts. void generate_iban(); // Used for generating IBAN. // Static keyword means that the value of running_number_ is the same for // all Account objects. // In other words, running_number_ is a class-wide attribute, there is // no own copies of it for each object of the class. static int running_number_; // More attributes/methods }; #endif // ACCOUNT_HH
file account.ccp:
#include "account.hh" #includeAccount::Account(const std::string& owner, bool has_credit): { generate_iban(); } // Setting initial value for the static attribute running_number_ int Account::running_number_ = 0; void Account::generate_iban() { ++running_number_; std::string suffix = ""; if(running_number_ < 10) { suffix.append("0"); } else if(running_number_ > 99) { std::cout << "Too many accounts" << std::endl; } suffix.append(std::to_string(running_number_)); iban_ = "FI00 1234 "; iban_.append(suffix); }
file main.cpp:
#include "account.hh" #include#include using namespace std; // Prints three accounts given as parameters. // If we knew containers, we could use one to store accounts in it, // and print the content of the container. // In this way, we could print any number of accounts. void print_three_accounts(const Account& a1, const Account& a2, const Account& a3) { cout << endl; cout << "Printing all three accounts:" << endl; a1.print(); a2.print(); a3.print(); cout << endl; } int main() { cout << "1: Creating three accounts" << endl; Account current_account("Tupu"); Account savings_account ("Hupu"); Account credit_card_account("Lupu", true); // Setting credit limit for a credit card account credit_card_account.set_credit_limit(100); // Trying to set credit limit for a non-credit card account current_account.set_credit_limit(100); // Saving money in all three accounts current_account.save_money(100); savings_account.save_money(200); credit_card_account.save_money(300); print_three_accounts(current_account, savings_account, credit_card_account); cout << "2: Taking money succesfully:" << endl; current_account.take_money(50); print_three_accounts(current_account, savings_account, credit_card_account); cout << "3: Taking money unsuccesfully:" << endl; current_account.take_money(100); print_three_accounts(current_account, savings_account, credit_card_account); cout << "4: Taking money succesfully from a credit card account:" << endl; credit_card_account.take_money(310); print_three_accounts(current_account, savings_account, credit_card_account); cout << "5: Taking money unsuccesfully from a credit card account:" << endl; credit_card_account.take_money(100); print_three_accounts(current_account, savings_account, credit_card_account); cout << "6: Transferring money succesfully: " << endl; savings_account.transfer_to(credit_card_account, 50); print_three_accounts(current_account, savings_account, credit_card_account); cout << "7: Transferring money unsuccesfully: " << endl; savings_account.transfer_to(current_account, 200); print_three_accounts(current_account, savings_account, credit_card_account); cout << "8: Transferring money succesfully from a credit card account:" << endl; credit_card_account.transfer_to(savings_account, 60); print_three_accounts(current_account, savings_account, credit_card_account); cout << "9: Transferring money unsuccesfully from a credit card account:" << endl; credit_card_account.transfer_to(savings_account, 100); print_three_accounts(current_account, savings_account, credit_card_account); return 0; }
Instructions:
(Function print_three_accounts in file main.cpp is not rational, but at this phase we do not know how to implement a function printing any number of bank accounts. The purpose of this function is to diminish the amount of repeated code and to make main function more clear.)
In this exercise, you will implement a program to handle different bank accounts. A bank account has a number (IBAN), owner, and balance. If a bank account has a credit card, it also has a credit limit. (In a credit card account, the balance can be negative as much as the credit limit is.)
You can save money in a bank account, you take money from it, and you can transfer money from it to another account. You can print information about a bank account in the form: owner : IBAN : balance. For a credit card account, you can set a credit limit.
In the code template, the class Account has a static attribute running_number_. A static attribute is not specific for an object (as usual attributes), for a class. In other words, its value is the same for all objects of the class, and if an object of the class changes the value, the new value is updated for all the other objects of the class. Such an attribute can be used to told the number of the objects of the class, and this is actually the case also in this program. The attribute in question is used in generating account numbers. For the first account created, the number is FI00 1234 01, for the second one it is FI00 1234 02, and so on. The Account class already has the method generate_iban, and thus, you need not care about these things any more. The call of the method generate_iban is already written in the code, as well as the initialization of the attribute running_number_.
The code template has a main program (in the file main.cpp). There you can see how the methods of Account have been called. In the class Account, implement all methods that have been called from the main program. Note that the Account class include the information about a single bank account.
In the main program, all methods have been called like void methods, i.e. methods without a return value. If you feel it useful to pass success/fail information for the caller, you can give a truth-value (bool) as the return value of such method. Now, the function can be called in both ways: as a method returning bool, or as a void method (if the caller is not interested in the return value).
After you have completed the class Account, the program will work with the given main program in the following way:
1: Creating three accounts Cannot set credit limit: the account has no credit card Printing all three accounts: Tupu : FI00 1234 01 : 100 euros Hupu : FI00 1234 02 : 200 euros Lupu : FI00 1234 03 : 300 euros 2: Taking money succesfully: 50 euros taken: new balance of FI00 1234 01 is 50 euros Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 200 euros Lupu : FI00 1234 03 : 300 euros 3: Taking money unsuccesfully: Cannot take money: balance underflow Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 200 euros Lupu : FI00 1234 03 : 300 euros 4: Taking money succesfully from a credit card account: 310 euros taken: new balance of FI00 1234 03 is -10 euros Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 200 euros Lupu : FI00 1234 03 : -10 euros 5: Taking money unsuccesfully from a credit card account: Cannot take money: credit limit overflow Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 200 euros Lupu : FI00 1234 03 : -10 euros 6: Transferring money succesfully: 50 euros taken: new balance of FI00 1234 02 is 150 euros Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 150 euros Lupu : FI00 1234 03 : 40 euros 7: Transferring money unsuccesfully: Cannot take money: balance underflow Transfer from FI00 1234 02 failed Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 150 euros Lupu : FI00 1234 03 : 40 euros 8: Transferring money succesfully from a credit card account: 60 euros taken: new balance of FI00 1234 03 is -20 euros Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 210 euros Lupu : FI00 1234 03 : -20 euros 9: Transferring money unsuccesfully from a credit card account: Cannot take money: credit limit overflow Transfer from FI00 1234 03 failed Printing all three accounts: Tupu : FI00 1234 01 : 50 euros Hupu : FI00 1234 02 : 210 euros Lupu : FI00 1234 03 : -20 euros
Step by Step Solution
There are 3 Steps involved in it
Step: 1
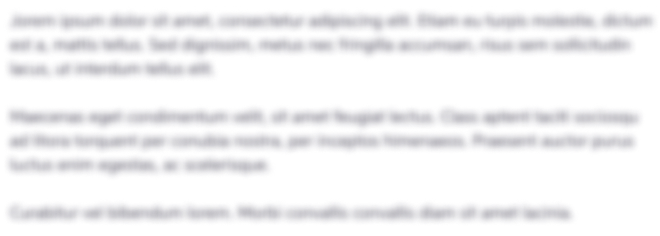
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started