Question
C++ coding! file date.hh: #ifndef DATE_HH #define DATE_HH class Date { public: // Default constructor. // Uses the default value 1 for day, month, and
C++ coding!
file date.hh:
#ifndef DATE_HH #define DATE_HH
class Date { public: // Default constructor. // Uses the default value 1 for day, month, and year. Date();
// Constructor. // If any of the given parameter is out of sensible limits // default value 1 used instead. Date(unsigned int day, unsigned int month, unsigned int year);
// Destructor ~Date();
// Advances the date with given amount of days. // Can't be anvanced by negative amounts. void advance(unsigned int days);
// Prints the date (dd.mm.yyyy). void print() const;
private: unsigned int day_; unsigned int month_; unsigned int year_;
// Returns true if the year of the date is a leap year, // otherwise returns false. bool is_leap_year() const; };
#endif // DATE_HH
file date.cpp:
#include "date.hh" #include
unsigned int const month_sizes[12] = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
Date::Date(): day_(1), month_(1), year_(1) { }
Date::Date(unsigned int day, unsigned int month, unsigned int year): day_(day), month_(month), year_(year) { if( month_ > 12 || month_ < 1 ) { month_ = 1; } if ( day_ > month_sizes[month_ - 1] || ( month_ == 2 && is_leap_year() && day > month_sizes[month - 1] + 1 ) ) { day_ = 1; } }
Date::~Date() { }
void Date::advance(unsigned int days) { day_ += days; while ( day_ > month_sizes[month_ - 1] ) { if ( month_ == 2 && day_ == 29 ) { return; } day_ -= month_sizes[month_ - 1]; if ( month_ == 2 && is_leap_year() ) { --day_; } ++month_;
if ( month_ > 12 ) { month_ -= 12; ++year_; } } }
void Date::print() const { if(day_ < 10) { std::cout << "0"; } std::cout << day_ << "."; if(month_ < 10) { std::cout << "0"; } std::cout << month_ << "."; if(year_ < 10) { std::cout << "0"; } std::cout << year_ << std::endl; }
bool Date::is_leap_year() const { return (year_ % 4 == 0) && (!(year_ % 100 == 0) || (year_ % 400 == 0)); } file main.cpp:
#include "date.hh" #include "book.hh" #include
using namespace std;
int main() { // Creating a date Date today(5, 5, 2020);
// Creating a book Book book1("Kivi", "Seitseman veljesta"); book1.print();
// Loaning a book book1.loan(today);
// Two weeks later today.advance(14);
// Trying to loan a loaned book book1.loan(today); book1.print();
// Renewing a book book1.renew(); book1.print();
// Returning the book book1.give_back();
// Trying to renew an available book book1.renew(); book1.print();
// Loaning again (two weeks later) book1.loan(today); book1.print();
return 0; } Instructions:
The final program has two simple classes: Date and Book. The former one describes a date, and it is already completed. Each date has three numeric values: day, month, and year. These numbers get their values, when a date object is created. A date can be printed as dd.mm.yyyy. A date can be advanced with the method advance. The class Date has a private method is_leap_year, telling if the year of the date is a leap year or not. The method is needed in advancing the date. It is not meant to be used outside of the class, and thus, it is declared in the private part.
Your task is to implement the class Book, which describes a library book. Each book has a title and an author, as well as the information if the book has been loaned or not. If a book is loaned, it has a loaning date and a returning date (due date).
A book can be loaned (unless it is already loaned), it can also be renewed (if it has been previously loaned) and returned. If a book can be loaned, the loaning method creates a loaning date (given as a parameter) and a due date that is 28 days after the loaning date. If a book can be renewed, the renewing method moves the due date 28 days later. (Note that here renewing works in a way, different from that of a real library. In a real library, the new due date is counted by starting from the renewing date, but here it is counted by starting from the old due date.)
The information about a book can be printed in the form: author : title. If the book is not loaned, the printing method prints the text available. If the book is loaned, the printing method prints the dates when the book has been loaned and when it should be returned. More precise printing form can be seen in the example below.
The code template has a main program (in the file main.cpp). There you can see how the methods of Book have been called. Implement the class Book which includes the information about a single book, and all the methods that are called in the main program. In the main program, there is only one book object, but there could be more of them.
There is no need to change the class Date, but you need it in implementing the class Book. Moreover, you can study it to see how a header and implementation files of a class look like.
After you have implemented the class Book, the program will work with the given main program in the following way:
Kivi : Seitseman veljesta - available Already loaned: cannot be loaned Kivi : Seitseman veljesta - loaned: 05.05.2020 - to be returned: 02.06.2020 Kivi : Seitseman veljesta - loaned: 05.05.2020 - to be returned: 30.06.2020 Not loaned: cannot be renewed Kivi : Seitseman veljesta - available Kivi : Seitseman veljesta - loaned: 19.05.2020 - to be returned: 16.06.2020
Step by Step Solution
There are 3 Steps involved in it
Step: 1
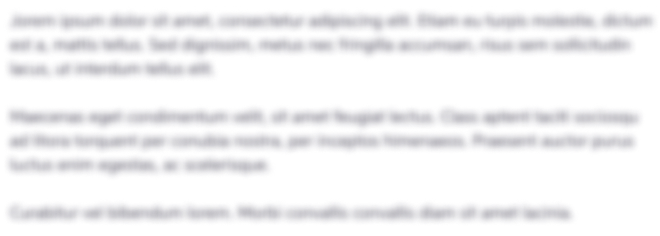
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started