Question
C++ coding-Follow the directions given All programs necessary are given below #ifndef CLASS_POINT_H #define CLASS_POINT_H /* ================================================================================ Program Name: Point class Program Description: This program
C++ coding-Follow the directions given
All programs necessary are given below
#ifndef CLASS_POINT_H #define CLASS_POINT_H /* ================================================================================
Program Name: Point class Program Description: This program defines and test class Point.
Your job: 1. Understand and run the given program without any change. 2. Combine the given three files into one .cpp file. You don't need to add any statements but you need to delete a few ones.
Notes: If a member function is defined within the class declaration, it's defined as "inline" by default. The general rule is that if a function has only one statement, it can be defined within the class. Otherwise it should be defined outside of the class after main().
=============================================================================== */ #include
using namespace std;
/* =================================================================== class Point declaration including some inline function definitions. =================================================================== */ class Point { public: Point() : x(0.0), y(0.0) {} // default constructor Point(const Point &p) : x(p.x), y(p.y) {}// copy constructor
Point(double new_x, double new_y) : x(new_x), y(new_y) {} // prefered. //Point(double new_x, double new_y) { x = new_x; y = new_y; }
// Accessors double getX() const { return x; }; double getY() const { return y; }; void print() const; // in format of (x, y) // Mutators void resetX(double new_x) { x = new_x; }; void resetY(double new_y) { y = new_y; }; void resetXY(double new_x, double new_y );
// Utilities Point add(const Point &p2) const; // addition of two points Point sub(const Point &p2) const; // difference of two points void move(double a, double b); // move current point to the added new position double distanceTo(const Point &p2) const; // distance between two points
private: double x; double y; };
/** This is a regular function. It accesses private members of Point class via the class's public members. */ double distanceOf2Points_v2(const Point& p1, const Point& p2);
#endif
/* ================================================================================
Program Name: Point class Program Description: This program defines and test class Point.
Your job: 1. Understand and run the given program without any change. 2. Combine the given three files into one .cpp file. You don't need to add any statements but you need to delete a few ones.
Notes: If a member function is defined within the class declaration, it's defined as "inline" by defautl. The general rule is that if a function has only one statement, it can be defined within the class. Otherwise it should be defined outside of the class after main().
=============================================================================== */ #include
/* ============================================ Definitions of some Point member functions ============================================ */ void Point::print() const { cout << "(" << x << ", " << y << ")" << endl; }
void Point::resetXY(double new_x, double new_y) { x = new_x; y = new_y; }
Point Point::add(const Point &p2) const { return Point(x + p2.x, y + p2.y); }
Point Point::sub(const Point &p2) const { return Point(x - p2.x, y - p2.y); }
void Point::move(double a, double b) { x += a; y += b; }
double Point::distanceTo(const Point &p2) const { double xDiff = x - p2.x; double yDiff = y - p2.y;
return sqrt(xDiff * xDiff + yDiff * yDiff); }
/** This a regular function. It accesses private members of Point class via the class's public members. */ double distanceOf2Points_v2(const Point& p1, const Point& p2) { double xDiff = p1.getX() - p2.getX(); double yDiff = p1.getY() - p2.getY();
return sqrt(xDiff * xDiff + yDiff * yDiff); }
/* ======================================================================
Program Name: Class Circle Program Description: This program declares and defined class Circle. Two data members and the following member functions should be included: Circle() {...} // default constructor Circle(double r) {...} // with radius r Circle(double r, Point p) {...} // with radius r and center p void setRadius(...) {...} double getArea(...) {...} // PI*radius^2 double getCircumference(...) {...} // Circumference = 2*PI*radius void printInfo() {...} ; bool isLargerThan(...) {...} void moveCenter(double x, double y) {...}
Instructions: 1. Imitate the sample program s10_class_rectangle.cpp to implement the Circle class described above and write testing code in the main() function. 2. Insert your code below wherever is marked "Your code below...".
Notes: 1. Usually, if a member function has only one statement, just put it in the class declaration. Otherwise, this member function should be defined outside of the class declaration after main(). 2. Create a dir h5_class_circle. You must copy h5_pointClass.h and h5_pointClass.cpp in order to compile your .cpp file.
======================================================================== */ #include
using namespace std;
class Circle { public: Circle() : radius(1.0) {} // default constructor.
// <<<<< Your code below...>>>>>>
private: double radius; Point center; static const double PI; // a static constant member };
const double Circle::PI = 3.1415926535;
int main () { // Print header. cout << "================================================================== "; cout << "This program will create a circle class and test it. "; cout << "================================================================== ";
/** =========================================================================== Imitate the code of s10_class_rectangle.cpp to test your class definition. <<<<<< Your code below...>>>>> =========================================================================== */
cout << endl;
return 0; }
/* =========================================================================== Define those member functions which are not defined within the class declaration. // <<<<<< Your code below...>>>>> =========================================================================== */
/* =============================================================================
Program Name: Sample Program 10 Program Description: The class has two private data members: width and length. Its public member functions include the following: Rectangular(...) // constructor void setSides(...) {...} void printSides() {...} double getArea(...) {...} double getPerimeter(...) {...} bool isSameArea(...) {...} void printInfo()const {...}
Notes: In the main() function, some objects of class Rectangle are created and are used to test the class definition and its member funtions. =============================================================================== */ #include
using namespace std;
class Rectangle { public: Rectangle() // default constructor //: width(1.0), length(1.0) {} // preferred { width = length = 1.0; }
Rectangle(double w, double l) // another constructor with parameters //: width (w), length(l) {} // preferred { width = w; length = l; }
void setSides(double w, double l) { width = w; length = l; } void printInfo() const; // defined after main() double getArea() const { return (width * length); } double getPerimeter() const { return (2 * width + 2 * length); } bool isLargerThan(Rectangle rect) const { return (getArea() > rect.getArea()); }
private: double width; double length; };
int main () { cout << "======================================================================= "; cout << "This program constructs and tests the Rectangle class. User is asked "; cout << "to enter width and length from keyboard once. "; cout << "======================================================================= ";
cout << fixed << setprecision( 2 );
cout << "==> Construct rect0 with the default constructor... "; Rectangle rect0; cout << "rect0: "; rect0.printInfo(); cout << " ";
cout << "==> Constuct rect1 of 10.00 x 20.00 with the 2nd constructor... "; Rectangle rect1(10,20); cout << "rect1: "; rect1.printInfo(); cout << " ";
cout << "==> After resetting sides of rect1 to 5.0 x 8.0... "; rect1.setSides(5, 8); // re-set the width and length cout << "rect1: "; rect1.printInfo(); cout << " "; double w, l; cout << "==> Input width and length for a rectangule... "; cout << "(seperated by a space) ==> "; cin >> w >> l;
while (cin.fail()) { cin.clear(); // clear tags of cin cin.ignore (256, ' '); cout << " Not valid numbers! Please try again... "; cout << "==> Input width and length for a rectangule... "; cout << "(seperated by a space) ==> "; cin >> w >> l; }
cout << " ==> Construct rect2 with the values from keyboard... "; Rectangle rect2(w,l); // use constructor to create an object. cout << "rect2: "; rect2.printInfo(); cout << " ";
cout << "==> Compare if rect1 and rect2 are the same large... "; if (rect1.isLargerThan(rect2)) cout << "rect1 is larger than rect2! "; else cout << "rect1 is NOT larger than rect2! ";
cout << endl;
return 0; }
void Rectangle::printInfo() const { cout << " Sides: " << width << " x " << length << "; "; cout << "Area: " << getArea() << "; Perimeter: " << getPerimeter() << endl; if ( fabs(width - length) <= 2 * DBL_EPSILON) cout << "This is a square! "; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
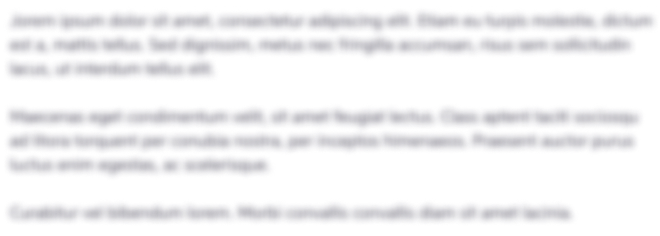
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started