Question
C++ coding-separate the code into 3 separate files, 2 .cpp and 1 .h, without any changes to the code #include #include #include #include #include using
C++ coding-separate the code into 3 separate files, 2 .cpp and 1 .h, without any changes to the code
#include
using namespace std;
/** ==================================================== Declaration of Class Point including some inline function definitions. ==================================================== */ class Point { /** This is a friend function of Point class. */ friend double distanceOf2Points_v1(Point p1, Point p2);
// Overloading "<<" operator. friend ostream& operator<<(ostream &output, const Point &p);
// Overloading "+" operator. friend Point operator+(const Point &p1, const Point &p2);
public: // Constructors Point() : x(0.0), y(0.0) {} // default constructor Point(double new_x, double new_y) : x(new_x), y(new_y) {} Point(const Point &p) : x(p.x), y(p.y) {} // copy constructor
// Accessors double getX() const { return x; }; double getY() const { return y; }; void print() const; // in format of (x, y) void print(string p) const; // in format of "p(x, y)"
// Mutators void resetX(double new_x) { x = new_x; }; void resetY(double new_y) { y = new_y; }; void resetXY(double new_x = 0.0, double new_y = 0.0);
// Utilities Point add(const Point &p2) const; // addition of two points Point sub(const Point &p2) const; // difference of two points void move1(double a, double b); // move without returning current object. Point& move2(double a, double b); // move and return current object. double distanceTo(const Point &p2) const; // distance between two points
// Overloading of operators Point operator+(const Point &p2); Point operator-(const Point &p2); bool operator==(const Point &p2) const;
private: double x; double y; };
/** This is a regular function. */ double distanceOf2Points_v2(const Point &p1, const Point &p2);
int main() { cout << "========================================== "; cout << "This program defines and tests the class Point. "; cout << "========================================== ";
cout << fixed << setprecision(2);
cout << "==> Test contructors, p1 with default construction, "; cout << "p2(4.0, 2.0) with regular constructor and "; cout << "p3 with copy constructor ... "; Point p1; cout << "p1"; p1.print(); Point p2(4.0, 2.0); p2.print("p2"); Point p3(p2); cout << "p3" << p3 << endl; // testing overloading <<. cout << " ";
cout << "==> Test getX() and getY() ... "; cout << "x-coordinate of p2 is : " << p2.getX() << endl; cout << "y-coordinate of p2 is : " << p2.getY() << endl; cout << " ";
cout << "==> Reset p1 with p1.reSetXY(5.0, 5.0) ... "; p1.resetXY(5.0, 5.0); p1.print("p1"); cout << " ";
cout << "==> After p4 = p1.add(p2) ... "; Point p4 = p1.add(p2); p4.print("p4"); cout << " ";
cout << "==> After p4 = p1.sub(p2) ... "; p4 = p1.sub(p2); p4.print("p4"); cout << " ";
cout << "==> Move p3 by (3, 3) with move1() ... "; p3.move1(3, 3); p3.print("p3"); cout << " ==> Move p3 by (3, 4) with move2() ... "; p3.move2(3, 4).print("p3"); cout << " ";
cout << "==> Find distance between p3 and p4 by member function: " << p3.distanceTo(p4) << endl; cout << "==> Find distance between p3 and p4 by friend function: " << distanceOf2Points_v1(p3, p4) << endl; cout << "==> Find distance between p3 and p4 by regular function: " << distanceOf2Points_v2(p3, p4) << endl;
cout << " ==> Test overloaded operators ... "; cout << "p1" << p1 << " + " << "p2" << p2 << " = " << p1 + p2 << endl; cout << "p1" << p1 << " - " << "p2" << p2 << " = " << p1 - p2 << endl; cout << "p1" << p1 << " and " << "p2" << p2 << " are same? "; cout << ((p1 == p2) ? "Yes!" : "No!") << " "; Point p5(p4); cout << "p4" << p4 << " and " << "p5" << p5 << " are same? "; cout << ((p4 == p5) ? "Yes!" : "No!") << " ";
return 0; }
/** ==================================== Definitions of some Point member functions ==================================== */ inline void Point::print() const { cout << "(" << x << ", " << y << ")" << endl; }
void Point::print(string p) const { cout << p << "(" << x << ", " << y << ")" << endl; }
void Point::resetXY(double new_x, double new_y) { x = new_x; y = new_y; }
inline Point Point::add(const Point &p2) const { return Point(x + p2.x, y + p2.y); }
inline Point Point::sub(const Point &p2) const { return Point(x - p2.x, y - p2.y); }
void Point::move1(double a, double b) { x += a; y += b; }
Point& Point::move2(double a, double b) { x += a; y += b;
return (*this); }
double Point::distanceTo(const Point &p2) const { double xDiff = x - p2.x; double yDiff = y - p2.y;
return sqrt(xDiff * xDiff + yDiff * yDiff); }
/** This is a friend function of Point class. */ double distanceOf2Points_v1(Point p1, Point p2) { double xDiff = p1.x - p2.x; double yDiff = p1.y - p2.y;
return sqrt(xDiff * xDiff + yDiff * yDiff); }
/** This is a regular function. */ double distanceOf2Points_v2(const Point &p1, const Point &p2) { double xDiff = p1.getX() - p2.getX(); double yDiff = p1.getY() - p2.getY();
return sqrt(xDiff * xDiff + yDiff * yDiff); }
ostream& operator<<(ostream &output, const Point &p) { output << "(" << p.x << ", " << p.y << ")"; return output; }
Point operator+(const Point &p1, const Point &p2) { return Point(p1.x + p2.x, p1.y + p2.y); }
Point Point::operator+(const Point &p2) { return Point(x + p2.x, y + p2.y); }
Point Point::operator-(const Point &p2) { return Point(x - p2.x, y - p2.y); }
bool Point::operator==(const Point &p2) const { return (fabs(x - p2.x) < 2 * DBL_EPSILON && fabs(y - p2.y) < 2 * DBL_EPSILON); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
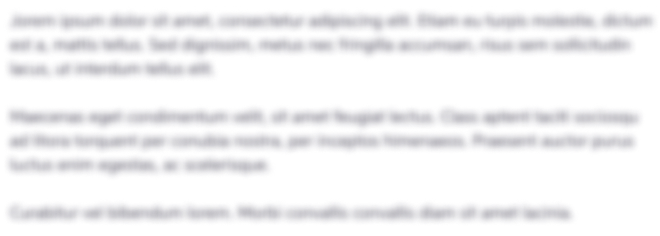
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started