Question
C++ Complete the declaration of the following IMMUTABLE class named Fraction. Each instance of the class contains a rational number represented as a fraction containing
C++
Complete the declaration of the following IMMUTABLE class named Fraction. Each instance of the class contains a rational number represented as a fraction containing an integer numerator over an integer denominator. The instance methods implement common arithmetic operations on fractions. Since the class is immutable it has no modifying methods. All arithmetic operations return new Fraction objects as their results without modifying the target nor the argument Fraction.
Your job consists of completing the following 5 tasks:
Complete the empty percentage instance method Complete the empty addInverseOf instance method Complete the empty squareRoot instance method Complete the empty divide instance method Complete the empty powerOf instance method
#include
1. Finish the percentage instance method.
Test | Result |
Fraction f1(2, 10); cout << f1.percentage() << endl; | 20 |
Fraction f1(59, 73); cout << f1.percentage() << endl; | 80 |
Fraction f1(19, 38); cout << f1.percentage() << endl; | 50 |
Fraction f1(12, 16); cout << f1.percentage() << endl; | 75 |
#include "Fraction.h" /* * Return the percentage equivalent of the target fraction. * * HINT: Check the percentage formula. Use the floor method, * you may review how floor works by viewing the C++ Library/API. * For example: https://en.cppreference.com/w/cpp/numeric/math * * Note that the tests expect and display values that are whole numbers. * * NOTE: The display of the results are already handled by the test cases. * You just need to return the int result. */ int Fraction::percentage() { // ADD YOUR CODE/SOLUTION BELOW return -1; // DUMMY RETURN, USE FLOOR. }
2. Finish the addInverseOf instance method.
Test | Result |
Fraction f1(1, 2); Fraction f2(5, 9); Fraction result = f1.addInverseOf(f2); cout << "The new numerator is " << result.getNumerator() << endl; cout << "The new denominator is " << result.getDenominator() << endl; | The new numerator is 23 The new denominator is 10 |
Fraction f1(21, 13); Fraction f2(8, 7); Fraction result = f1.addInverseOf(f2); cout << "The new numerator is " << result.getNumerator() << endl; cout << "The new denominator is " << result.getDenominator() << endl; | The new numerator is 259 The new denominator is 104 |
Fraction f1(3, 172); Fraction f2(19, 144); Fraction result = f1.addInverseOf(f2); cout << "The new numerator is " << result.getNumerator() << endl; cout << "The new denominator is " << result.getDenominator() << endl; | The new numerator is 24825 The new denominator is 3268 |
#include "Fraction.h"
/* * Return a NEW fraction representing the addition of the target fraction and the inverse * of the argument fraction. The resulting fraction may not be expressed in minimal terms. * You must create a NEW fraction object and use getNumerator & getDenominator. * * NOTE: The display of the results are already handled by the test cases. * You just need to return the new Fraction. */ Fraction Fraction::addInverseOf(Fraction f2) { return f2; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
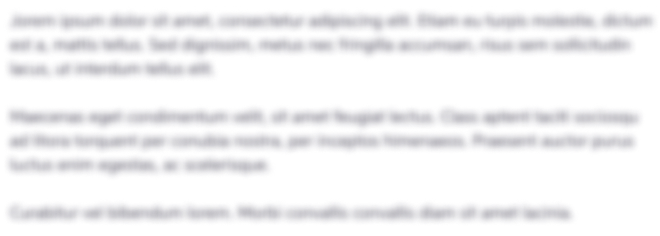
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started