Question
C++ Description Project 09 introduces the student to the use of two-dimensional arrays. In this project you will write a C++ program that computes the
C++
Description
Project 09 introduces the student to the use of two-dimensional arrays. In this project you will write a C++ program that computes the Boolean algebra results of OR, AND and XOR of two two-dimensional arrays of binary digits.
To complete this task your program must open an input file containing the number of rows and columns for two two-dimensional arrays and the binary data for the two arrays. After reading the binary data into the appropriate arrays, output their contents as shown in the sample solution.
Next, calculate the Boolean algebra results of OR, AND and XOR on the two arrays. Use three arrays to hold the results of the Boolean algebra computations. After calculating the three result arrays, output them as shown in the sample solution. Be sure to look at the sample solution for the format of the output of the arrays the input and result arrays are printed side by side. The project slides will have more information on this form of the output.
Lastly, the program is to output the integer value of each row of the result arrays (OR, AND and XOR) as shown in the sample solution. This output is side by side in field widths of 8 left justified. To determine the integer value of a row in the array, a binary to decimal conversion is performed as described later.
In addition to the function main(), your solution must include several non-trivial functions to receive full credit
The first number in the input file is the number of rows of the arrays, and the second number is the number of columns (maximum size for the array is 10 rows and 10 columns). Each of these numbers has a comment to the right of it. This extraneous information must be skipped before reading the next value (look at the provided input files for further clarification). After the row and column values, there are integer values of 1 and 0 corresponding to the two arrays that need to be read. These values appear in the format of the array and the numbers are separated by spaces
Requirements
No global variables are allowed
This program will require at least 5 functions in addition to main some possible functions to consider and what parameters may be needed are given below:
1) OpenInputFile 1 parameter - the input file stream. This function does all the work to successfully open an input file for reading
2) ReadData 5 possible parameters this function can read in the row and column numbers from the input file and both input arrays. It would also require the input file stream.
3) Calculate results arrays Could use one function to find results for OR, XOR and AND or write 3 separate functions for each result. In any case, need the 2 input arrays, the output array or arrays and the number of rows and number of columns in the arrays. This function finds the results of OR, XOR and AND of the two input arrays.
4)Print out the input arrays this function would require 4 parameters both input arrays and the number of rows and columns this function performs the output for the input arrays
5) Print out the output arrays 5 parameters the row and column values and the result arrays for OR, XOR and AND. This function writes out the output information for the result arrays
6) Print out the decimal values This function takes the same 5 parameters as the function that prints out the result arrays. This function will print out the decimal value for each row of the three results arrays. This function could call another function that calculates the integer value for a given row of an array. To do this, a loop is necessary to go through all possible rows of the result arrays
7) Calculate integer value of a row this function has 3 parameters the array to process, the row number of the array to process and the number of columns. It will calculate the integer equivalent of the specified row for the array passed in
Input file:
10 // number of rows 10 // number of columns 0 0 1 1 0 1 1 1 1 1 1 1 0 0 1 0 0 0 0 0 0 1 1 0 0 1 1 1 1 1 1 0 0 1 1 0 0 0 0 0 1 1 0 0 0 1 1 1 1 1 1 1 1 1 1 0 0 1 1 0 0 0 0 0 0 1 1 0 0 1 1 1 1 1 1 0 1 1 0 0 0 0 0 0 0 1 0 0 1 1 1 1 1 1 1 1 1 0 0 0 1 1 1 1 1 0 0 1 1 0 0 0 0 0 0 1 1 0 0 1 1 1 1 1 1 0 1 1 0 0 0 0 0 0 0 1 0 0 1 1 1 1 1 1 1 1 1 0 0 0 0 0 1 1 0 1 1 1 1 1 1 1 0 0 1 0 0 0 0 0 0 1 1 0 0 1 1 1 1 1 1 0 0 1 1 0 0 0 0 0 1 1 0 0 0 1 1 1 1 1
Answer Output code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
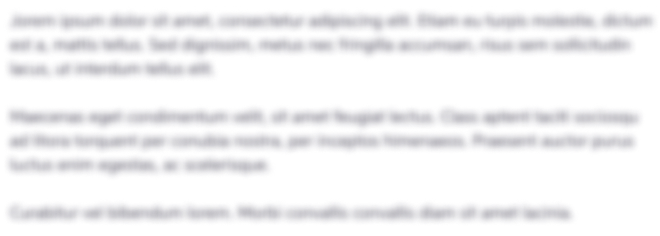
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started