Question
C++ doubly Linked Lists - Complete the program below that keeps track of a telephone directory sorted by first name. Use the comments below to
C++
doubly Linked Lists - Complete the program below that keeps track of a telephone directory sorted by first name. Use the comments below to implement the routines that are not implemented.
#include
#include
using namespace std;
class DLL;
class Node
{
friend class DLL;
private:
string firstName;
string lastName;
string phoneNumber;
Node * nextRecord;
Node * previousRecord;
};
class DLL
{
private:
Node * head;
public:
DLL();
DLL(const DLL&); //optional
~DLL();
void insert(string, string, string);
void print();
void searchByName(string, string);
void remove(string, string);
};
//--------------------------------------------
//the default constructor
DLL::DLL()
{
}
//--------------------------------------------
//the copy constructor: optional
DLL::DLL(const DLL& source)
{
}
//--------------------------------------------
//the destructor
DLL::~DLL()
{
}
//--------------------------------------------
//print the linked list
void DLL::print ()
{
}
//--------------------------------------------
//search for a particular person in the list and print the
//corresponding phone number
void DLL::searchByName (string fName, string lName)
{
}
//--------------------------------------------
//create a node and insert it in the list in order (list
//should be sorted by the persons first name)
void DLL::insert (string fName, string lName, string phone)
{
}
//--------------------------------------------
//remove a person's record from the list
void DLL::remove (string fName, string lName)
{
}
//--------------------------------------------
int main ()
{
DLL list1;
list1.insert ("Mayssaa", "Najjar", "878-635-1234");
list1.insert ("Jim", "Meyer", "337-465-2345");
list1.insert ("Joe", "Didier", "352-654-1983");
list1.insert ("Yara", "Sarkis", "858-694-1787");
list1.insert ("Nancy", "Garcia", "617-227-5454");
list1.print();
list1.searchByName ("Nancy", "Garcia");
list1.remove ("Mayssaa", "Najjar");
list1.remove ("Yara", "Sarkis");
list1.remove ("Jim", "Meyer");
list1.print();
//this part is optional
DLL list2(list1);
list2.print();
return 0;
}
#includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
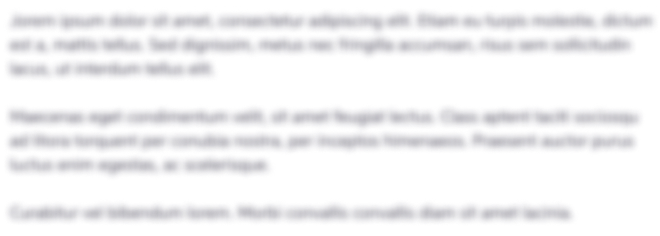
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started