Question
C++ Each programming assignments includes two parts: 1. Code Reflection: A brief explanation of the code and its purpose, and a brief discussion of your
C++ Each programming assignments includes two parts:
1. Code Reflection: A brief explanation of the code and its purpose, and a brief discussion of your experience in developing it, including any issues that you encountered while completing the exercise and what approaches you took to solve them.
2.associated pseudocode or flowchart Annotated .cpp code file(s
The following critical elements should be addressed in your project submission:
Pseudocode or Flowchart: A pseudocode or flowchart description of the code that is clear and understandable and captures accurate logic to translate to the programming language.
Annotation / Documentation: All code should also be well-commented. This is a practiced art that requires striking a balance between commenting everything, which adds a great deal of unneeded noise to the code, and commenting nothing. Well-annotated code requires you to:
A. Explain the purpose of lines or sections of your code, detailing the approach and method you took to achieve a specific task in the code
#include
#include "CSVparser.hpp"
using namespace std;
//============================================================================ // Global definitions visible to all methods and classes //============================================================================
// forward declarations double strToDouble(string str, char ch);
// define a structure to hold bid information struct Bid { string bidId; // unique identifier string title; string fund; double amount; Bid() { amount = 0.0; } };
//============================================================================ // Static methods used for testing //============================================================================
/** * Display the bid information to the console (std::out) * * @param bid struct containing the bid info */ void displayBid(Bid bid) { cout << bid.bidId << ": " << bid.title << " | " << bid.amount << " | " << bid.fund << endl; return; }
/** * Prompt user for bid information using console (std::in) * * @return Bid struct containing the bid info */ Bid getBid() { Bid bid;
cout << "Enter Id: "; cin.ignore(); getline(cin, bid.bidId);
cout << "Enter title: "; getline(cin, bid.title);
cout << "Enter fund: "; cin >> bid.fund;
cout << "Enter amount: "; cin.ignore(); string strAmount; getline(cin, strAmount); bid.amount = strToDouble(strAmount, '$');
return bid; }
/** * Load a CSV file containing bids into a container * * @param csvPath the path to the CSV file to load * @return a container holding all the bids read */ vector
// Define a vector data structure to hold a collection of bids. vector
// initialize the CSV Parser using the given path csv::Parser file = csv::Parser(csvPath);
try { // loop to read rows of a CSV file for (int i = 0; i < file.rowCount(); i++) {
// Create a data structure and add to the collection of bids Bid bid; bid.bidId = file[i][1]; bid.title = file[i][0]; bid.fund = file[i][8]; bid.amount = strToDouble(file[i][4], '$');
//cout << "Item: " << bid.title << ", Fund: " << bid.fund << ", Amount: " << bid.amount << endl;
// push this bid to the end bids.push_back(bid); } } catch (csv::Error &e) { std::cerr << e.what() << std::endl; } return bids; }
// FIXME (2a): Implement the quick sort logic over bid.title
/** * Partition the vector of bids into two parts, low and high * * @param bids Address of the vector
}
/** * Perform a quick sort on bid title * Average performance: O(n log(n)) * Worst case performance O(n^2)) * * @param bids address of the vector
// FIXME (1a): Implement the selection sort logic over bid.title
/** * Perform a selection sort on bid title * Average performance: O(n^2)) * Worst case performance O(n^2)) * * @param bid address of the vector
/** * Simple C function to convert a string to a double * after stripping out unwanted char * * credit: http://stackoverflow.com/a/24875936 * * @param ch The character to strip out */ double strToDouble(string str, char ch) { str.erase(remove(str.begin(), str.end(), ch), str.end()); return atof(str.c_str()); }
/** * The one and only main() method */ int main(int argc, char* argv[]) {
// process command line arguments string csvPath; switch (argc) { case 2: csvPath = argv[1]; break; default: csvPath = "eBid_Monthly_Sales_Dec_2016.csv"; }
// Define a vector to hold all the bids vector
// Define a timer variable clock_t ticks;
int choice = 0; while (choice != 9) { cout << "Menu:" << endl; cout << " 1. Load Bids" << endl; cout << " 2. Display All Bids" << endl; cout << " 3. Selection Sort All Bids" << endl; cout << " 4. Quick Sort All Bids" << endl; cout << " 9. Exit" << endl; cout << "Enter choice: "; cin >> choice;
switch (choice) {
case 1: // Initialize a timer variable before loading bids ticks = clock();
// Complete the method call to load the bids bids = loadBids(csvPath);
cout << bids.size() << " bids read" << endl;
// Calculate elapsed time and display result ticks = clock() - ticks; // current clock ticks minus starting clock ticks cout << "time: " << ticks << " clock ticks" << endl; cout << "time: " << ticks * 1.0 / CLOCKS_PER_SEC << " seconds" << endl;
break;
case 2: // Loop and display the bids read for (int i = 0; i < bids.size(); ++i) { displayBid(bids[i]); } cout << endl;
break;
// FIXME (1b): Invoke the selection sort and report timing results
// FIXME (2b): Invoke the quick sort and report timing results
} }
cout << "Good bye." << endl;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
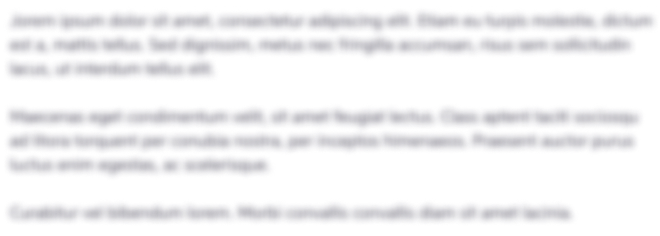
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started