Question
c++ I just need the SortedUserType.cpp. Everything else i already coded. (Class) Implement SortedUserType as a class sorted user objects. Create and implement the specification
c++
I just need the SortedUserType.cpp. Everything else i already coded.
(Class) Implement SortedUserType as a class sorted user objects. Create and implement the specification file (SortedUserType.h) and implementation file (SortedUserType.cpp). The SortedUserType class uses NodeUser struct to create a node of a user in the list. For testing purposes, SortedUserType can store a maximum of 5 users (maxList). The user object will be inserted based on the increasing order of the users dateOfBirth.
2.4 (Struct) Implement NodeUser struct in the SortedUserType.h and SortedUserType.cpp.
NodeUser has two fields:
User userInfo; // data info
NodeUser* next; // pointer
2.5 (SortedUserType operations) the SortedUserType class has the following operation:
Normal operators:
(Constructor) SortedUserType (); (Transformer) void ResetList(); (Transformer) void MakeEmpty();// delete all item in the list (Observer) bool IsFull() const; (Observer) int GetLength() const;
Pointer related operators:
(Observer) User* GetUser(User* aUser, bool& found) const; // get a user from the list whose firstName, lastName, and dateOfBirth match with aUser. (Transformer) void PutUser(User* aUser); // put aUser in to the list according to dateOfBirth order. (Transformer) void DeleteUser(User* aUser); // delete a user from the list whose firstName, lastName, and dateOfBirth match with aUser. (Observer) User* GetNextUser(); // get the next user in the list
Note that:
* All functions must follow the algorithms of sorted list ADT
** GetUser, PutUser, and DeleteUser functions must use the comparedTo(User* aUser) function of the User class.
***GetUser(User* aUser, bool& found) must search for the user based on firstName, lastName, and dateOfBirth information. It must follow the algorithm of getting an item in a sorted list ADT. The best way to do this is to call comparedTo(User* aUser) function of the User class to determine the match of two user objects. If the current item does not match, go the next item on the list.
***PutUser(User* aUser) must put aUser into the list based on dateOfBirth order. If the new user has the same dateOfBirth, firstName, and lastName with a user in the list, it cannot be put into the list. Again, calling comparedTo(User* aUser) function is the best way to handle this requirement.
***DeleteUser function only can delete a user from the list whose firstName, lastName, and dateOfBirth match with aUser. Again, calling comparedTo(User* aUser) function is the best way to handle this requirement.
code:
DateType.h:
#include
DateType.cpp:
//File DataType.cpp contains the implementation of class DataType #include "DateType.h" #include
// Numbers of days in each month static int daysInMonth[] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
//Names of the months static string conversionTable[] = {"Error", "January", "February", "March", "April", "May","June", "July", "August", "September", "October", "November", "December"};
void DateType::Initialize (int newMonth, int newDay, int newYear) /* Post: if newMonth, newDay and newYear represents a valid date year is set to newYear; month is set to newMonth; day is set to newDay; otherwise a string exception is thrown, stating the first incorrect parameter */ { if (newMonth < 1 || newMonth>12) throw string("Month is invalid"); else if (newDay < 1 || newDay > daysInMonth[newMonth]) throw string("Day is invalid"); else if (newYear < 1583) throw string("Year is invalid"); year = newYear; month = newMonth; day = newDay; } int DateType::GetMonth() const //Accessor function for data member month { return month; }
string DateType::GetMonthAsString() const //returns data member as a string { return conversionTable[month]; }
int DateType::GetYear() const //Accessor function for data member year. { return year; }
int DateType::GetDay() const //Accessor function for data member day { return day; }
RelationType DateType::ComparedTo(DateType aDate) const /* Pre: self and aDate have been initialized post: function value = LESS, if self comes before aDate. = EQUAL, if self is the same as aDate. = GREATER, if self comes after aDate. */ { if (year < aDate.year) return LESS; else if (year > aDate.year) return GREATER; else if (month < aDate.month) return LESS; else if (month > aDate.month) return GREATER; else if (day < aDate.day) return LESS; else if (day > aDate.day) return GREATER; else return EQUAL; }
DateType DateType::Adjust(int daysAway) const //Pre: self has been initialized //Post: function value =newDate daysAway from self { int newDay = day + daysAway; int newMonth = month; int newYear = year; bool finished = false; int daysInThisMonth; DateType returnDate; while (!finished) { daysInThisMonth = daysInMonth[newMonth]; if (newMonth == 2) if (((newYear % 4 == 0) && !(newYear % 100 == 0)) || (newYear % 400 == 0)) daysInThisMonth++; if (newDay <= daysInThisMonth) finished = true; else { newDay = newDay - daysInThisMonth; newMonth = (newMonth % 12) + 1; if (newMonth == 1) newYear++; } }
returnDate.Initialize(newMonth, newDay, newYear); return returnDate; }
UserDetails.h
#include "DateType.h" #include
UserDetails.cpp
//Implementation of User class #include "UserDetails.h" //Constructor User::User() { strcpy(firstName, ""); strcpy(lastName, ""); date.Initialize(1, 1, 1970); } //function is to initialize a user object with given values. void User::Initialize(char firstName[6], char lastName[6], DateType date) { strcpy(this->firstName,firstName); strcpy(this->lastName,lastName); this->date = date; } //function compare the self user with aUser RelationType User::comparedTo(User* aUser) const { if (this->comparedTo(aUser) == LESS) { return LESS; } else if (this->comparedTo(aUser) == GREATER) { return GREATER; } else { return EQUAL; } } //function returns a string that contains all information of the user string User::ToString() { return string(firstName)+" "+ string(lastName) + ", DOB: " + date.GetMonthAsString() + " " + to_string(date.GetDay()) + ", " + to_string(date.GetYear()); }
SortedUserType.h
//File Sorted UserType.h has the declarations and definitions for sorted user adt #include "User.h" using namespace std;
struct NodeUser;
class SortedUserType { // store a max of 5 users (maxList) public: SortedUserType(); //class constructor
void ResetList(); void MakeEmpty(); //deletes all items in the list bool IsFull() const; int GetLength() const;
//pointer operations User* GetUser(User* user, bool &found) const; void PutUser(User* user); void DeleteUser(User* user); User* GetNextUser();
private: NodeUser* listData; int length; int maxList; NodeUser* currentPos; //variables user in functions };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
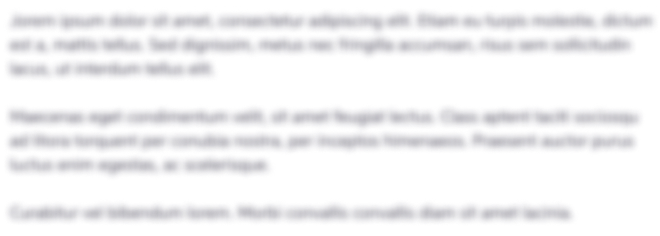
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started